Write a program that gets two car information from the user, compares them, and print out the information of the "better car. The user will first give the information regarding the two cars in the following format: horsepower as an integer, top speed as an integer, fuel efficiency as a double and comfort as an integer.
Write a program that gets two car information from the user, compares them, and print out the information of the "better car. The user will first give the information regarding the two cars in the following format: horsepower as an integer, top speed as an integer, fuel efficiency as a double and comfort as an integer.
Chapter8: Arrays
Section: Chapter Questions
Problem 4GZ
Related questions
Question
C++
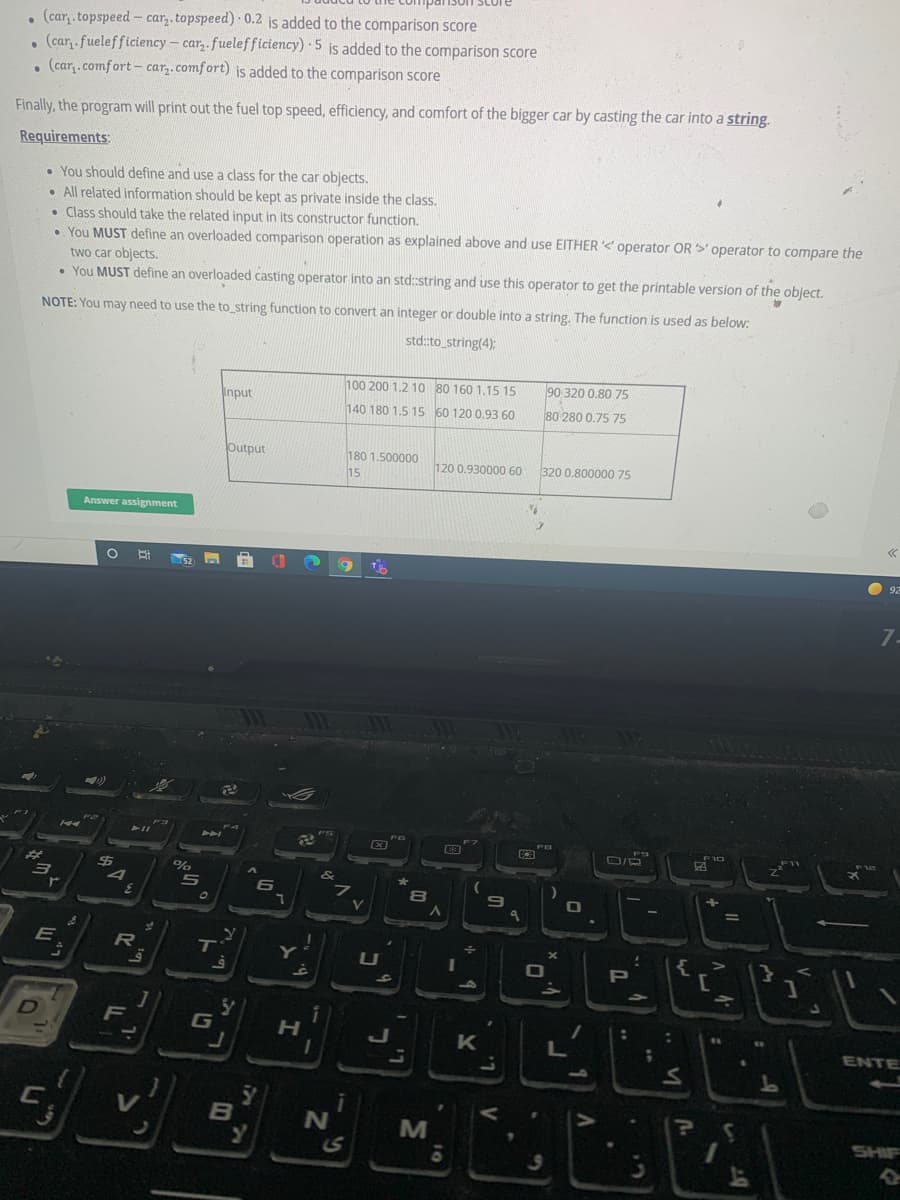
Transcribed Image Text:1SCore
. (car,.topspeed - car. topspeed) 0.2 is added to the comparison score
. (car,.fuelefficiency – car,. fuelefficiency) 5 is added to the comparison score
. (car,.comfort- car,.comfort) is added to the comparison score
Finally, the program will print out the fuel top speed, efficiency, and comfort of the bigger car by casting the car into a string.
Requirements:
• You should define and use a class for the car objects.
• All related information should be kept as private inside the class.
• Class should take the related input in its constructor function.
• You MUST define an overloaded comparison operation as explained above and use EITHER <' operator OR >' operator to compare the
two car objects.
• You MUST define an overloaded casting operator into an std:string and use this operator to get the printable version of the object.
NOTE: You may need to use the to string function to convert an integer or double into a string. The function is used as below:
std:to_string(4);
100 200 1.2 10 80 160 1.15 15
90 320 0.80 75
Input
140 180 1.5 15 60 120 0.93 60
80 280 0.75 75
Output
180 1.500000
15
120 0.930000 60
320 0.800000 75
Answer assignment
O 92
7.
23
6
E
R
T'
U
PI
F
G
ENTE
L.
B
SHIF
..
V
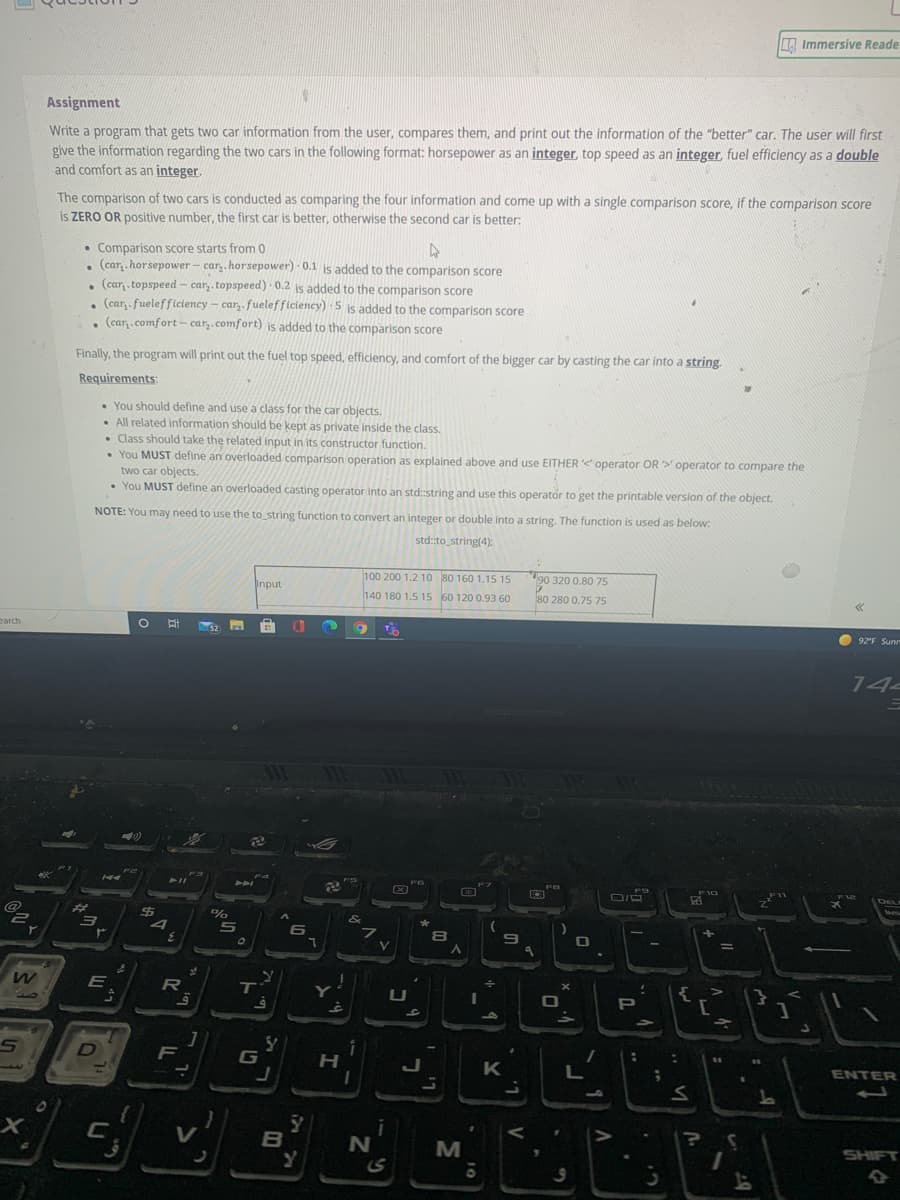
Transcribed Image Text:Immersive Reade
Assignment
Write a program that gets two car information from the user, compares them, and print out the information of the "better" car. The user will first
give the information regarding the two cars in the following format: horsepower as an integer, top speed as an integer, fuel efficiency as a double
and comfort as an integer.
The comparison of two cars is conducted as comparing the four information and come up with a single comparison score, if the comparison score
is ZERO OR positive number, the first car is better, otherwise the second car is better:
• Comparison score starts from 0
, (car, .horsepower - car,. horsepower) 0.1 is added to the comparison score
. (car, . topspeed - car,. topspeed) 0.2 is added to the comparison score
, (car, fuelefficiency – car,. fuelefficiency) 5 is added to the comparison score
, (car,.comfort- car,.comfort) is added to the comparison score
Finally, the program will print out the fuel top speed, efficiency, and comfort of the bigger car by casting the car into a string.
Requirements:
You should define and use a class for the car objects.
• All related information should be kept as private inside the class.
• Class should take the related input in its constructor function.
• You MUST define an overloaded comparison operation as explained above and use EITHER operator OR > operator to compare the
two car objects.
• You MUST define an overloaded casting operator into an std::string and use this operator to get the printable version of the object.
NOTE: You may need to use the to string function to convert an integer or double into a string. The function is used as below:
std::to string(4):
100 200 1.2 10 80 160 1.15 15
90 320 0.80 75
Input
140 180 1.5 15 60 120 0.93 60
80 280 0.75 75
earch
92'F Suns
14
イイ
%23
%
5
フ
ア
E
RI
T
U
P
F
G
K
ENTER
V
B
SHIFT
V
ト
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
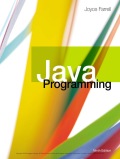
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
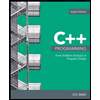
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
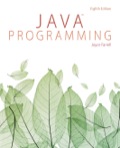
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
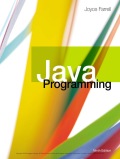
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
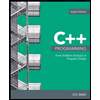
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
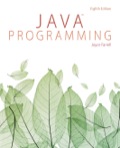
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
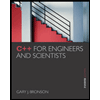
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr