Write a program that loads in historical data on popular baby-names for the last 100 years. You can type in a name, and it graphs the rank of that name over time. A high ranking, like 5, means the name was the 5th most popular that year (top of the graph), while a low ranking like 879 means the name was not that popular (bottom of the graph). Write it in python the latest version The first function you will write and test is the clean_data function. The function is described in the starter code in a function header comment above the function. Here is the function: def clean_data(list, x): #TODO: write your code here pass Take a look at the first few lines of the data file: A 83 140 228 286 426 612 486 577 836 0 0 Aaliyah 0 0 0 0 0 0 0 0 0 380 215 Aaron 193 208 218 274 279 232 132 36 32 31 41 Each line is a baby name followed by how popular the name was in 11 different years. Each number is a ranking, so Aaron was 193th most popular name in 1900 and 41st most popular name in 2000. The lower the number, the more popular the name. However, if the name was not popular enough to make the list in a particular year, a zero was recorded. If we plot the data as is, a name that was so unpopular that it didn’t make the list (ranked 0) will appear most popular (since lower means more popular). Therefore, we need to clean up the data a bit in order for it to make sense. The function clean_data has two parameters. The first parameter is a list (e.g. a list of rankings by year for a particular baby name). The second parameter is a value x. The function will do in-place modification of the parameter list and replace all zeros with the value x. You will write this function generally. Here are three of the cases you should test: # test 1 # before data is cleaned list1 = [0, 4, 3, 2, 0, 6, 7, 8, 3, 0, 7, 7, 8, 3, 0] x1 = 5 # after data is cleaned list1 = [5, 4, 3, 2, 5, 6, 7, 8, 3, 5, 7, 7, 8, 3, 5] # test 2 # before data is cleaned list2 = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0] x2 = 10001 # after data is cleaned list2 = [10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001, 10001] # test 3 # before data is cleaned list3 = [857, 797, 972, 883, 0, 0, 0, 0, 0, 0, 0] x3 = 1113 # after data is cleaned list3 = [857, 797, 972, 883, 1113, 1113, 1113, 1113, 1113, 1113, 1113] here is what I wrote for the clean data function: def clean_data(lists, x): for i in range(0, len(lists)): if lists[i] == 0: lists[i] = x return lists Then: Next step is You are going to writing a script that takes in a list of names as a single input string namestring = input("Enter baby names: ") For example, the prompt with the input could look like this: python3 main.py Enter baby names: Mary Megan Isabelle Your code should produce the following plot [1]: [1] The first image uploaded Plot should appear in your file tree with the filename: MaryMeganIsabelle.png. Another example is if the prompt with the input is: python3 main.py Enter baby names: Mario Marie Mary Marta Your code should produce the following plot [2]: [2] The second image uploaded Plot should appear in your file tree with the filename: MarioMarieMaryMarta.png. Once you are done developing, remove plt.show(), type: plt.ion() before your plot commands and type: plt.savefig("filename.png") After the plotting commands (but name the file properly) to create an image file that will get created in the file tree on the left. Make sure you final code runs to completion without hanging in the terminal. In other words, it is ready for the next command or to be run again. Your code should run for lots of different name combinations. You only need to handle names that are in the data file. You don’t to handle the case where the name is spelled wrong, not capitalized properly, or any other error handling. Assume the names are typed in correctly. Your code should be able to plot any number of names listed. the data file is called: names-data.txt: here is some of the data in the file: A 83 140 228 286 426 612 486 577 836 0 0 Aaliyah 0 0 0 0 0 0 0 0 0 380 215 Aaron 193 208 218 274 279 232 132 36 32 31 41 Abagail 0 0 0 0 0 0 0 0 0 0 958 Abbey 0 0 0 0 0 0 0 0 537 451 428 Abbie 431 552 742 924 0 0 0 0 752 644 601 Abbigail 0 0 0 0 0 0 0 0 0 953 562 Abby 0 0 0 0 0 906 782 548 233 211 209 Abdiel 0 0 0 0 0 0 0 0 0 925 721 Abdul 0 0 0 0 0 0 0 903 0 0 0 Abdullah 0 0 0 0 0 0 0 0 0 1000 863 Abe 248 328 532 764 733 0 0 0 0 0 0 Abel 664 613 626 575 542 491 497 422 381 385 354 Abigail 0 0 0 0 854 654 615 317 150 50 14 Abigale 0 0 0 0 0 0 0 0 0 0 959 Abigayle 0 0 0 0 0 0 0 0 0 0 720 Abner 569 637 930 0 0 0 0 0 977 0 996 Abraham 144 158 261 350 408 410 503 347 274 238 214 Abram 677 735 0 0 0 0 0 0 875 0 802 Abril 0 0 0 0 0 0 0 0 0 0 869 Ada 85 110 154 196 244 331 445 627 962 0 0 Adah 727 860 0 0 0 0 0 0 0 0 0 Adalberto 0 0 0 0 722 773 725 863 891 0 0 Adaline 832 828 0 0 0 0 0 0 0 0 0 Adam 178 200 280 376 444 407 144 38 22 39 46 Adan 0 0 0 985 920 977 936 729 601 516 504 Addie 165 213 249 324 460 686 0 0 0 0 0
A 83 140 228 286 426 612 486 577 836 0 0
Aaliyah 0 0 0 0 0 0 0 0 0 380 215
Aaron 193 208 218 274 279 232 132 36 32 31 41
Abagail 0 0 0 0 0 0 0 0 0 0 958
Abbey 0 0 0 0 0 0 0 0 537 451 428
Abbie 431 552 742 924 0 0 0 0 752 644 601
Abbigail 0 0 0 0 0 0 0 0 0 953 562
Abby 0 0 0 0 0 906 782 548 233 211 209
Abdiel 0 0 0 0 0 0 0 0 0 925 721
Abdul 0 0 0 0 0 0 0 903 0 0 0
Abdullah 0 0 0 0 0 0 0 0 0 1000 863
Abe 248 328 532 764 733 0 0 0 0 0 0
Abel 664 613 626 575 542 491 497 422 381 385 354
Abigail 0 0 0 0 854 654 615 317 150 50 14
Abigale 0 0 0 0 0 0 0 0 0 0 959
Abigayle 0 0 0 0 0 0 0 0 0 0 720
Abner 569 637 930 0 0 0 0 0 977 0 996
Abraham 144 158 261 350 408 410 503 347 274 238 214
Abram 677 735 0 0 0 0 0 0 875 0 802
Abril 0 0 0 0 0 0 0 0 0 0 869
Ada 85 110 154 196 244 331 445 627 962 0 0
Adah 727 860 0 0 0 0 0 0 0 0 0
Adalberto 0 0 0 0 722 773 725 863 891 0 0
Adaline 832 828 0 0 0 0 0 0 0 0 0
Adam 178 200 280 376 444 407 144 38 22 39 46
Adan 0 0 0 985 920 977 936 729 601 516 504
Addie 165 213 249 324 460 686 0 0 0 0 0
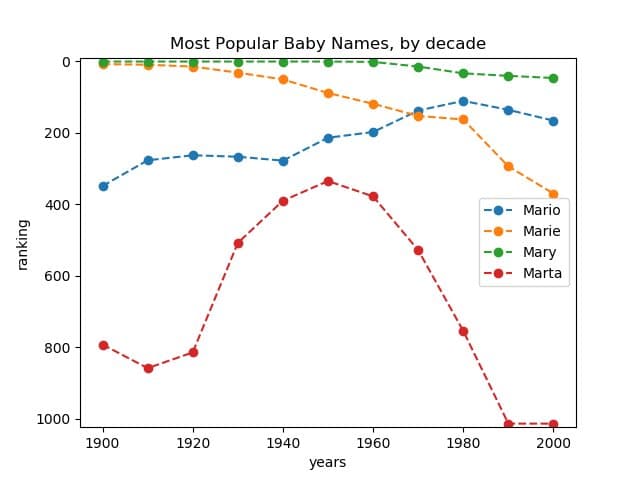
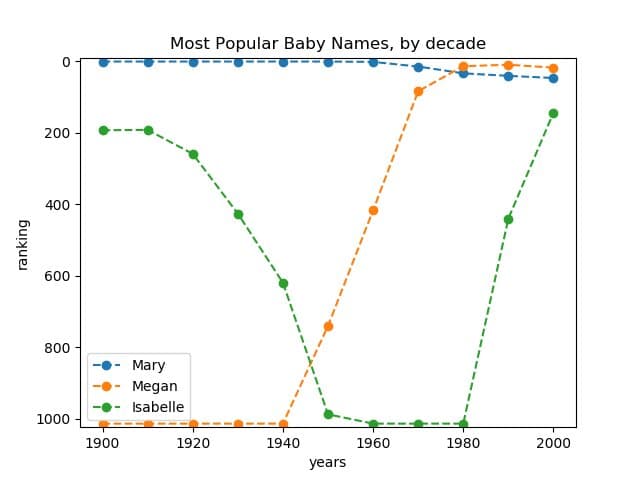

Step by step
Solved in 4 steps with 2 images

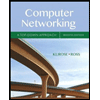
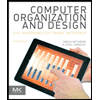
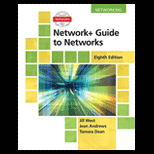
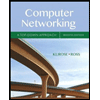
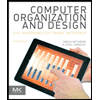
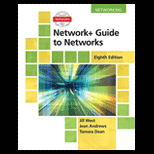
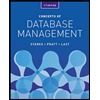
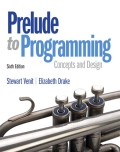
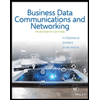