Write a program that outputs the nodes of a graph in a breadth first traversal. in c ++ 10 0 1 3 -999 1 4 -999 2 5 -999 3 2 -999 4 -999 5 7 8 -999 6 4 7 -999 7 -999 8 -999 9 7 8 -999 #include #include #include #include using namespace std; template struct nodeType { Type info; nodeType *link; }; template class linkedListIterator { public: linkedListIterator() { current = nullptr; } linkedListIterator(nodeType *ptr) { current = ptr; } Type operator*() { return current->info; } linkedListIterator operator++() { current = current->link; return *this; } bool operator==(const linkedListIterator& right) const { return (current == right.current); } bool operator!=(const linkedListIterator& right) const { return (current != right.current); } private: nodeType *current; }; template class linkedListType { public: const linkedListType& operator=(const linkedListType&) { if (this != &otherList) { copyList(otherList); } return *this; } void initializeList() { destroyList(); } bool isEmptyList() const { return (first == nullptr); } void print() const { nodeType *current; current = first; while (current != nullptr) { cout << current->info << " "; current = current->link; } } int length() const { return count; } void destroyList() { nodeType *temp; while (first != nullptr) { temp = first; first = first->link; delete temp; } last = nullptr; count = 0; } Type front() const { assert(first != nullptr); return first->info; } Type back() const { assert(last != nullptr); return last->info; } virtual bool search(const Type& searchItem) const = 0; virtual void insertFirst(const Type& newItem) = 0; virtual void insertLast(const Type& newItem) = 0; virtual void deleteNode(const Type& deleteItem) = 0; linkedListIterator begin() { linkedListIterator temp(first); return temp; } linkedListIterator end() { linkedListIterator temp(nullptr); return temp; } linkedListType() { first = nullptr; last = nullptr; count = 0; } linkedListType(const linkedListType& otherList) { first = nullptr; copyList(otherList); } ~linkedListType() { destroyList(); } protected: int count; nodeType *first; nodeType *last; private: void copyList(const linkedListType& otherList) { nodeType *newNode; nodeType *current; if (first != nullptr) destroyList(); if (otherList.first == nullptr) { first = nullptr; last = nullptr; count = 0; } else { current = otherList.first; count = otherList.count; first = new nodeType; first->info = current->info; first->link = nullptr; #include #include using namespace std; class Graph { int V; list *adj; void DFSUtil(int v, bool visited[]); public: Graph(int V); void addEdge(int v, int w); void DFS(int v); }; Graph::Graph(int V) { this->V = V; adj = new list[V]; } void Graph::addEdge(int v, int w) { adj[v].push_back(w); } void Graph::DFSUtil(int v, bool visited[]) { visited[v] = true; cout << v << " "; list::iterator i; for (i = adj[v].begin(); i != adj[v].end(); ++i) { if (!visited[*i]) { DFSUtil(*i, visited); } } } void Graph::DFS(int v) { bool *visited = new bool[V]; for (int i = 0; i < V; i++) { visited[i] = false; } DFSUtil(v, visited); } int main() { Graph g(10); g.addEdge(0, 1); g.addEdge(0, 3); g.addEdge(1, 4); g.addEdge(3, 2); g.addEdge(2, 5); g.addEdge(5, 7); g.addEdge(5, 8); cout << "Following is Depth First Traversal \n"; g.DFS(0); cout << endl; system("pause"); } please help me figure out how to do it
1 4 -999
2 5 -999
3 2 -999
4 -999
5 7 8 -999
6 4 7 -999
7 -999
8 -999
9 7 8 -999
#include<iostream>
#include<list>
using namespace std;
class Graph
{
int V;
list<int> *adj;
void DFSUtil(int v, bool visited[]);
public:
Graph(int V);
void addEdge(int v, int w);
void DFS(int v);
};
Graph::Graph(int V)
{
this->V = V;
adj = new list<int>[V];
}
void Graph::addEdge(int v, int w)
{
adj[v].push_back(w);
}
void Graph::DFSUtil(int v, bool visited[])
{
visited[v] = true;
cout << v << " ";
list<int>::iterator i;
for (i = adj[v].begin(); i != adj[v].end(); ++i)
{
if (!visited[*i])
{
DFSUtil(*i, visited);
}
}
}
void Graph::DFS(int v)
{
bool *visited = new bool[V];
for (int i = 0; i < V; i++)
{
visited[i] = false;
}
DFSUtil(v, visited);
}
int main()
{
Graph g(10);
g.addEdge(0, 1);
g.addEdge(0, 3);
g.addEdge(1, 4);
g.addEdge(3, 2);
g.addEdge(2, 5);
g.addEdge(5, 7);
g.addEdge(5, 8);
cout << "Following is Depth First Traversal \n";
g.DFS(0);
cout << endl;
system("pause");
}

Step by step
Solved in 4 steps with 3 images

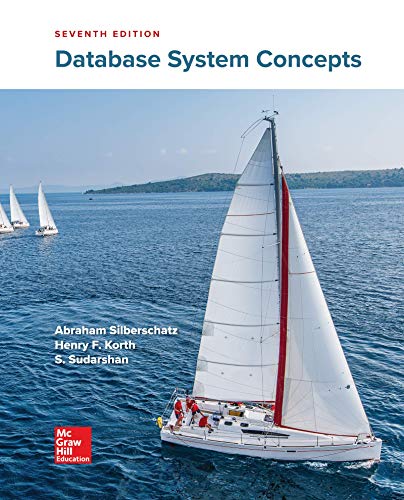
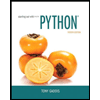
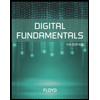
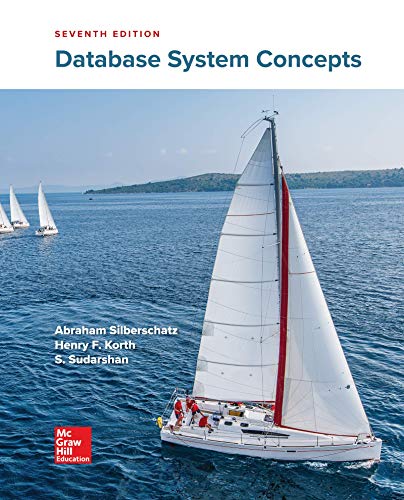
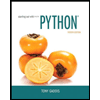
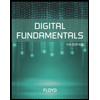
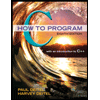
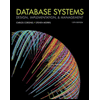
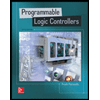