Write a program that prompts a user to input the city name, distance, and travel time for three destination citites. Your program will use 2 new typedef structs to store the information, then find and output the tour with the shortest distance. Your first new typedef should: • be named timeType, with a struct named timeType_struct • contain 3 data members: o hours, minutes, and seconds as integer values Your second new typef should: • be named tourType, with a struct named tourType_struct contain 3 data members: o a 50 character city name o an integer distance a travel time as data type timeType Your program will need the following 2 functions: a function that prompts a user to input the values for the data members of a tourType struct and returns the tourType struct to the calling function • a void function that takes a tourType parameter and prints the data in the struct Within your main function you will need to: • create a 3 element tourType array use your value-returning function to prompt for and read data into the array • loop through the array to find the tour with the shortest distance use your void function to print the tour with the shortest distance NOTES: Be sure to include your function prototypes before your main function, and your function definitions after your main function. Sample output (sample input shown with underline): Enter tour data for 3 cities : Enter a city name: Chicago Enter an integer distance: 300 Enter travel time as HH:MM:SS: 06:00:01 Enter a city name: Pittsburgh Enter an integer distance: 600 Enter travel time as HH:MM:SS: 10:30:00 Enter a city name: Miami Enter an integer distance: 500 Enter travel time as HH:MM:SS: 08:00:00 Shortest Tour City name: Chicago Distance: 300 Travel time: 06:00:01
Write a program that prompts a user to input the city name, distance, and travel time for three destination citites. Your program will use 2 new typedef structs to store the information, then find and output the tour with the shortest distance. Your first new typedef should: • be named timeType, with a struct named timeType_struct • contain 3 data members: o hours, minutes, and seconds as integer values Your second new typef should: • be named tourType, with a struct named tourType_struct contain 3 data members: o a 50 character city name o an integer distance a travel time as data type timeType Your program will need the following 2 functions: a function that prompts a user to input the values for the data members of a tourType struct and returns the tourType struct to the calling function • a void function that takes a tourType parameter and prints the data in the struct Within your main function you will need to: • create a 3 element tourType array use your value-returning function to prompt for and read data into the array • loop through the array to find the tour with the shortest distance use your void function to print the tour with the shortest distance NOTES: Be sure to include your function prototypes before your main function, and your function definitions after your main function. Sample output (sample input shown with underline): Enter tour data for 3 cities : Enter a city name: Chicago Enter an integer distance: 300 Enter travel time as HH:MM:SS: 06:00:01 Enter a city name: Pittsburgh Enter an integer distance: 600 Enter travel time as HH:MM:SS: 10:30:00 Enter a city name: Miami Enter an integer distance: 500 Enter travel time as HH:MM:SS: 08:00:00 Shortest Tour City name: Chicago Distance: 300 Travel time: 06:00:01
Chapter6: Using Arrays
Section: Chapter Questions
Problem 2CP
Related questions
Question
In C programing complete the prompt in the picture:
This is the code we are given to start with:
void printTour(const tourType dest);
tourType getData();
int main()
{
}
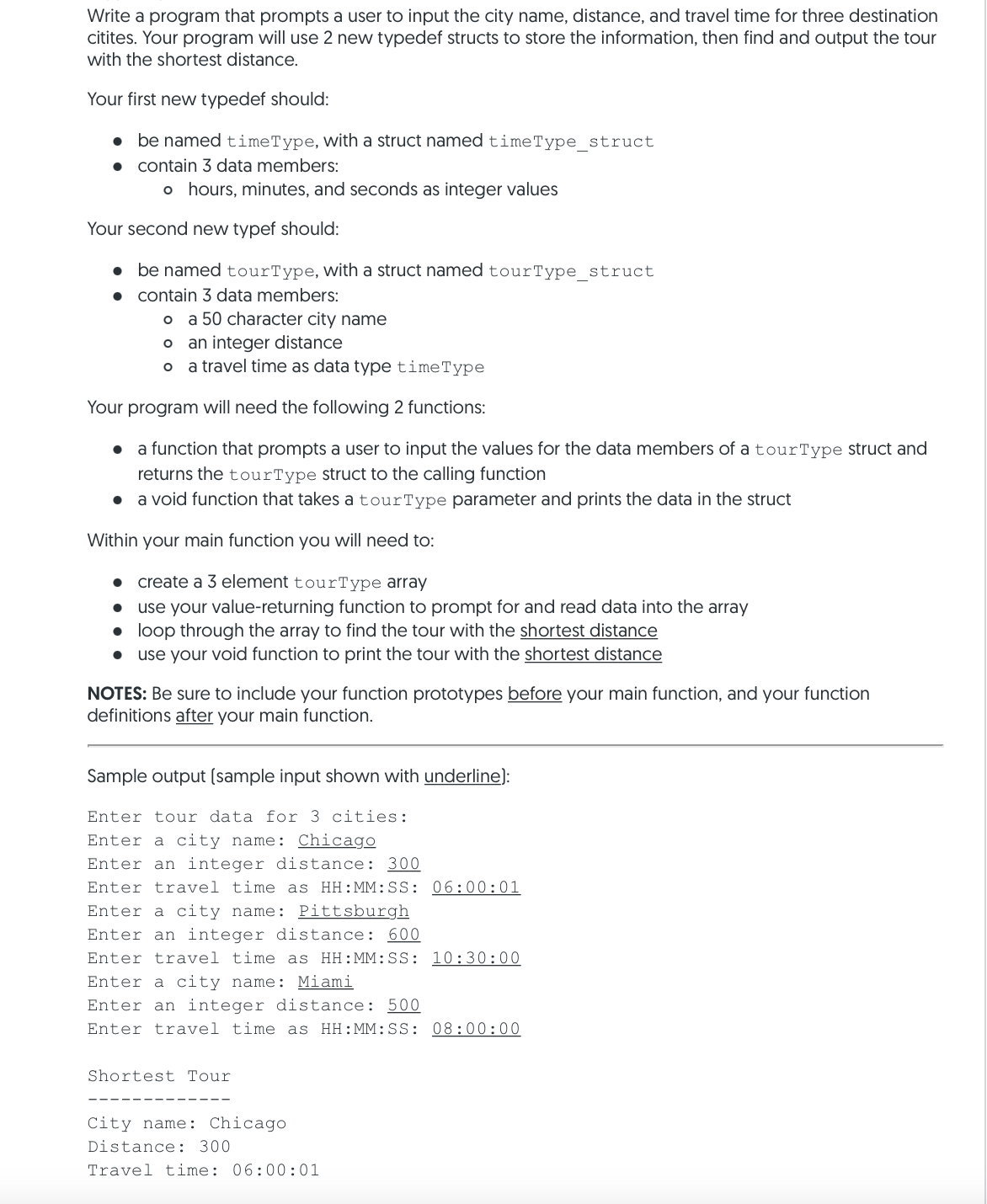
Transcribed Image Text:Write a program that prompts a user to input the city name, distance, and travel time for three destination
citites. Your program will use 2 new typedef structs to store the information, then find and output the tour
with the shortest distance.
Your first new typedef should:
• be named timeType, with a struct named timeType_struct
contain 3 data members:
o hours, minutes, and seconds as integer values
Your second new typef should:
• be named tourType, with a struct named tourType_struct
contain 3 data members:
o a 50 character city name
an integer distance
o a travel time as data type timeType
Your program will need the following 2 functions:
a function that prompts a user to input the values for the data members of a tourType struct and
returns the tourType struct to the calling function
• a void function that takes a tourType parameter and prints the data in the struct
Within your main function you will need to:
create a 3 element tourType array
use your value-returning function to prompt for and read data into the array
• loop through the array to find the tour with the shortest distance
use your void function to print the tour with the shortest distance
NOTES: Be sure to include your function prototypes before your main function, and your function
definitions after your main function.
Sample output (sample input shown with underline):
Enter tour data for 3 cities:
Enter a city name: Chicago
Enter an integer distance: 300
Enter travel time as HH:MM:SS: 06:00: 01
Enter a city name: Pittsburgh
Enter an integer distance: 600
Enter travel time as HH:MM:SS: 10:30:00
Enter a city name: Miami
Enter an integer distance: 500
Enter travel time as HH:MM:SS: 08:00:00
Shortest Tour
City name: Chicago
Distance: 300
Travel time: 06:00:01
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
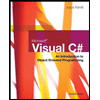
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
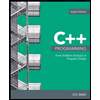
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
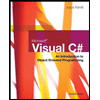
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
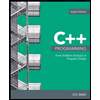
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning