Write a program that prompts the user to enter a numeric temperature value that may be a float. A second prompt should then ask the user to indicate whether the user wants the entered temperature value converted to degrees Celsius or degrees Fahrenheit by entering either an 'F' or a 'C' for a second prompt. For example, if the user enters 212 at the first prompt and the character C for the second prompt you are to convert 212 degrees F to the Celsius equivalent (which would be 100.0). Converted temperatures should be rounded to 1 decimal place. If the user enters 26 at the first prompt and the character F at the second one, then your programs should convert 26 degrees C to the Fahrenheit equivalent (which is 78.8 deg F)
Python program with explainations
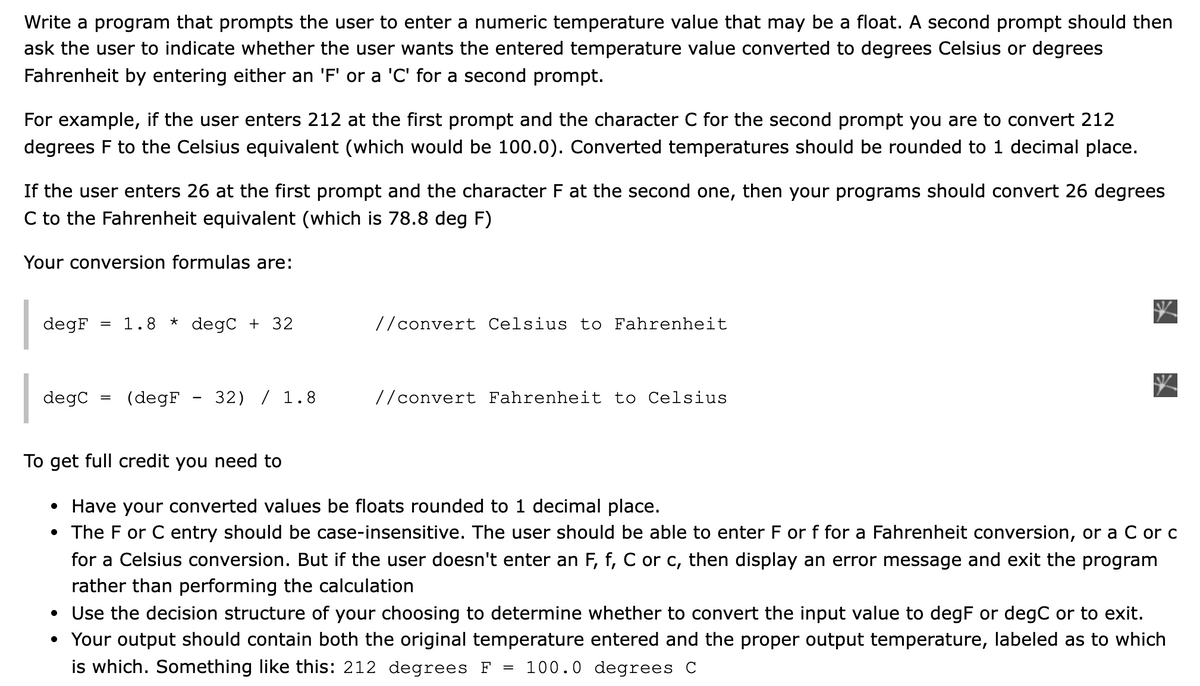
![Your prompt strings might look something like this, although you can choose your own words.
Please enter a temperature value to convert:
Convert to Fahrenheit or Celsius? Enter either F or C:
A possible example program run might look like this, where 50 deg C is converted to 122.0 deg F. Your output should indicated
which number is in which temperature system, like the following.
7k Python Shell
File Edit
Shell Debug Options Windows Help
Python 3.3.0 (v3.3.0:bd8afb90ebf2, Sep 29 2012, 10:55:4
8) [MSC v.1600 32 bit (Intel)] on win32
Type "copyright", "credits" or "license ()" for more inf
ormation.
|>>>
RESTART
>>>
Enter a temperature value to convert: 50
Convert to Fahrenheit or Celsius? Enter F or C: f
50.0 deg C = 122.0 degrees F
|>>> |
And, what if the user does not enter an F, f, C, or c? Suppose they type in k or simply hit enter without typing any character at
all?. Then your output should look similar to the following. You do not have to account for all possible user input errors, and for
this program you can assume the user will not enter a number that is lower than absolute zero.
76 Python Shell
File Edit
Shell Debug Options Windows Help
Python 3.3.0 (v3.3.0:bd8afb90ebf2, Sep 29 2012, 10:55:4
8) [MSC v.1600 32 bit (Intel)] on win32
Type "copyright", "credits" or "license ()" for more inf
ormation.
>>>
RESTART
==
>>>
Enter a temperature value to convert: 45.2
Convert to Fahrenheit or Celsius? Enter F or C: m
You did not enter an F or C.
Goodbye
>>> |
Tf vou get good and stuck email me VOur code and we'll get it working](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb12e7222-506e-487d-a20e-061b70f8136b%2F39285844-6079-48b9-9c3d-92a8fc40fcb2%2Fihny1si_processed.png&w=3840&q=75)

Below is the code text so you don't have to type, but make sure to match the text with the image above. It should be like the image in your editor with proper indentation.
temp = float(input("Enter a temperature value to convert: "))
convert = input("Conver to Fahrenheit or Celsius? Enter F or C: ")
if (convert == 'f' or convert == 'F') :
degF = 1.8 * temp + 32
print("\n" + str(round(temp, 1)) + " degrees Celsius = " + str(round(degF, 1)) + " degrees Fahrenheit" )
elif (convert == 'c' or convert == 'C') :
degC = (temp - 32) / 1.8
print("\n" + str(round(temp, 1)) + " degrees Fahrenheit = " + str(round(degC, 1)) + " degrees Celsius" )
else:
print("\nYou did not enter an F or C. Goodbye.")
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

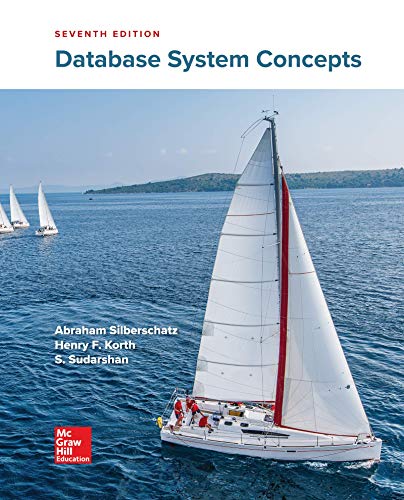
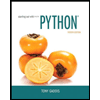
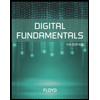
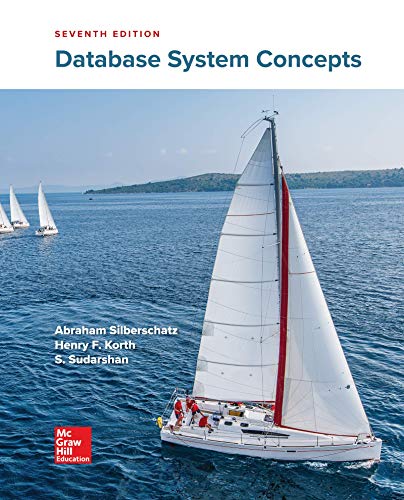
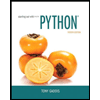
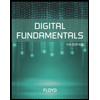
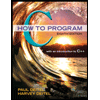
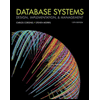
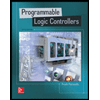