Write a program that quizzes the user on the names of the capital cities for all the states in the United States. Each state is presented to the user, and they must enter the name of the capital. The program then either tells the user they're correct or tells them the correct answer. The user's answer should not be case-sensitive. At the end, the program tells the user the total number they got correct.
Java
Lab 5 Problem Statement
Write a
What is the capital of Alabama? Montogomery The correct answer is Montgomery. What is the capital of Alaska? juneau That is the correct answer! ... You got 32 out of 50 correct! |
The state capitals will be stored in a 2D String array of size 2 x 50, with the first column the name of the state and the second the name of the capital city, for all 50 states. To create the state capitals array, follow these instructions:
- Copy the String from the attached file into your program (found below these instructions). The String is a pipe delimited list of states and capitals listed as state|capital|state|capital|... The list is alphabetical.
- Read the String into a Scanner object using something like: Scanner scanStr = new Scanner(stateCapitals);
- You must set the delimiter for the scanner before using it, before the loop: scanStr.useDelimiter("\\|");
- Create the necessary 2D array by declaring the array and then using a for loop to scan each element using scanStr.next() .
- You must also close the scanner when you're done with it, after the loop: scanStr.close();
- Text file with stateCapitals String:
- String stateCapitals = "Alabama|Montgomery|Alaska|Juneau|Arizona|" +
"Phoenix|Arkansas|Little Rock|California|Sacramento|Colorado|" +
"Denver|Connecticut|Hartford|Delaware|Dover|Florida|" +
"Tallahassee|Georgia|Atlanta|Hawaii|Honolulu|Idaho|" +
"Boise|Illinois|Springfield|Indiana|Indianapolis|Iowa|Des Moines|"
+
"Kansas|Topeka|Kentucky|Frankfort|Louisiana|Baton Rouge|" +
"Maine|Augusta|Maryland|Annapolis|Massachusetts|Boston|" +
"Michigan|Lansing|Minnesota|St. Paul|Mississippi|Jackson|" +
"Missouri|Jefferson City|Montana|Helena|Nebraska|Lincoln|" +
"Nevada|Carson City|New Hampshire|Concord|New Jersey|" +
"Trenton|New Mexico|Santa Fe|New York|Albany|North Carolina|" +
"Raleigh|North Dakota|Bismarck|Ohio|Columbus|Oklahoma|" +
"Oklahoma City|Oregon|Salem|Pennsylvania|Harrisburg|" +
"Rhode Island|Providence|South Carolina|Columbia|South Dakota|" +
"Pierre|Tennessee|Nashville|Texas|Austin|Utah|Salt Lake City|" +
"Vermont|Montpelier|Virginia|Richmond|Washington|Olympia|" +
"West Virginia|Charleston|Wisconsin|Madison|Wyoming|Cheyenne";
The entire program can be written in the main class, but you must modularize your work into appropriate methods that are called from main. You will need the following:
- String[][] getData() - a method that creates and returns the 2D array of states and capitals as described above.
- int play(String[][] data) - a method that presents each state to the user, reads in their capital city, displays if they are correct or not, as shown above, and keeps count of and returns how many correct answers there are.
- void showResult(int numberCorrect) - a method that displays to the user how many capitals they got correct after they have gone through all 50 states.
Notes:
- Do not use any global variables.
- Be sure to test your code with states that have a multi-word capital cities, like Little Rock or Salt Lake City.
- Remember to make the answers case-insensitive, so that Olympia, olympia, and OLYMPIA are all good answers to the question "What is the capital of Washington?"

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

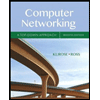
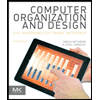
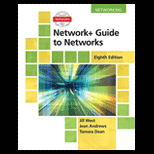
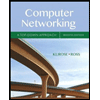
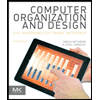
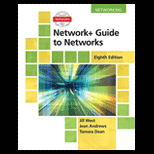
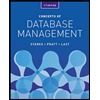
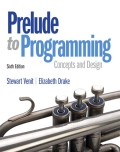
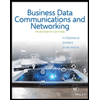