Write a program that reads a course's title and enrolled student information (student id, student score) and displays the input data followed by the frequency of each score in the course. The end of input is indicated by letter q on the last line For example, for the input: 10 90 12 50 17 85 15 90 42 75 20 90 30 75 78 50 61 50 21 85 22 50 q The output is: COP 3804 class list. 10 90 12 50 17 85 15 90 42 75 20 90 30 75 78 50 61 50 21 85 22 50 COP 3804 class scores 90 3 50 4 85 2 75 2 Given classes: • class Student represents a student • class Roster represents a course class LabProgram contains the main method that reads course data (i.e. course title and students' information), creates a Roster object with the input data and produces the output by calling relevant methods of the Roster object Your task is to complete the following methods: class Student:
import java.util.Scanner;
public class LabProgram {
public static Roster getInput(){
/* Reads course title, creates a roster object with the input title. Note that */
/* the course title might have spaces as in "COP 3804" (i.e. use nextLine) */
/* reads input student information one by one, creates a student object */
/* with each input student and adds the student object to the roster object */
/* the input is formatted as in the sample input and is terminated with a "q" */
/* returns the created roster */
/* Type your code here */
}
public static void main(String[] args) {
Roster course = getInput();
course.display();
course.dislayScores();
}
}
public class Student {
String id;
int score;
public Student(String id, int score) {
/* Student Employee */
/* Type your code here */
}
public String getID() {
/* returns student's id */
/* Type your code here */
}
public int getScore() {
/* returns student's score */
/* Type your code here */
}
}
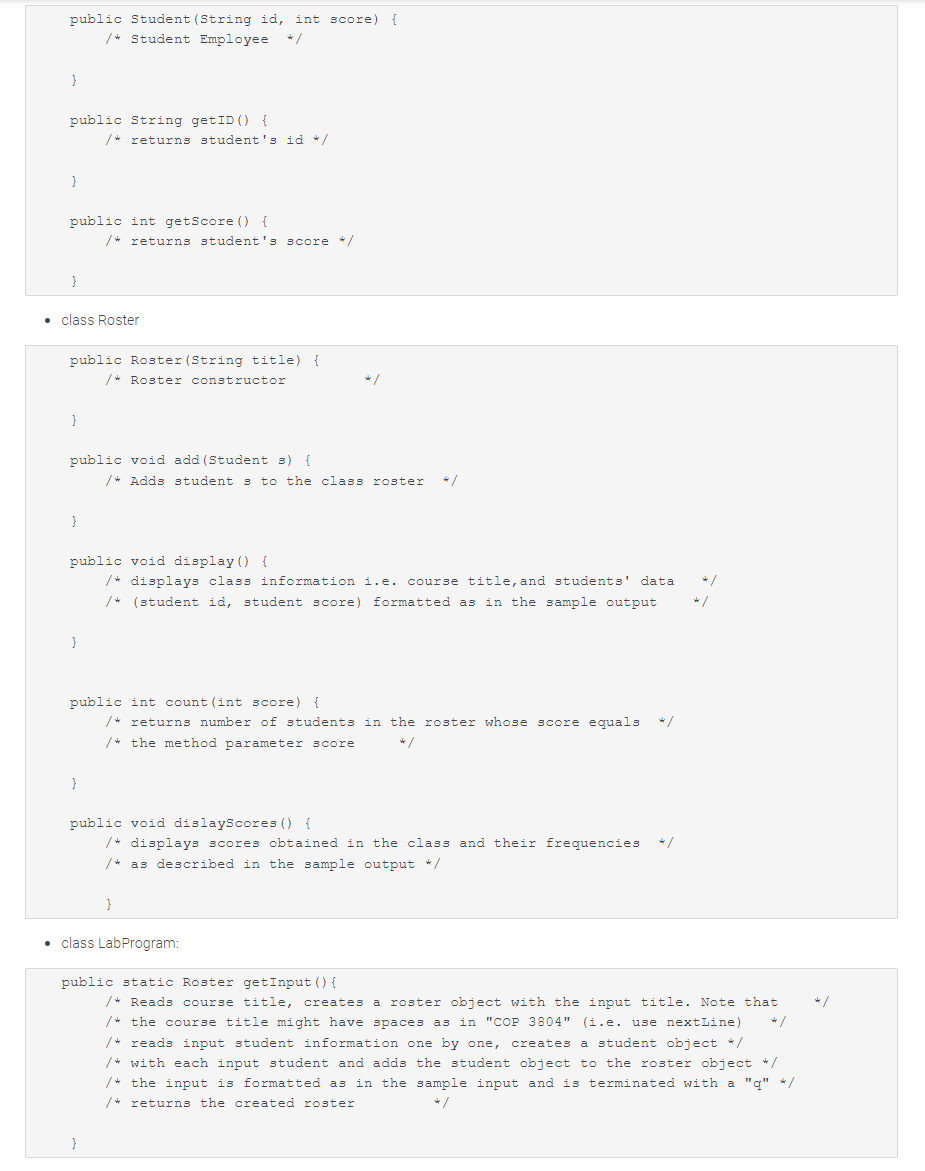
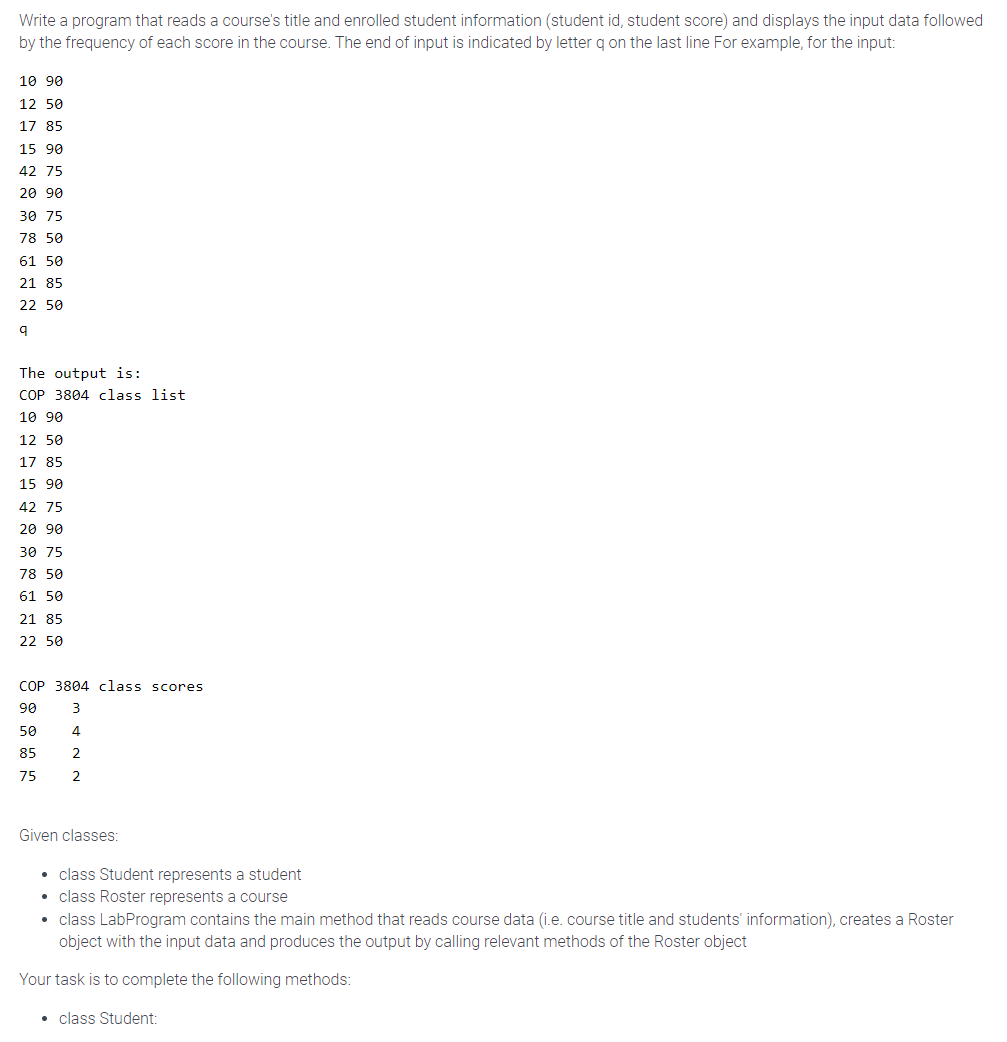

Step by step
Solved in 3 steps with 1 images

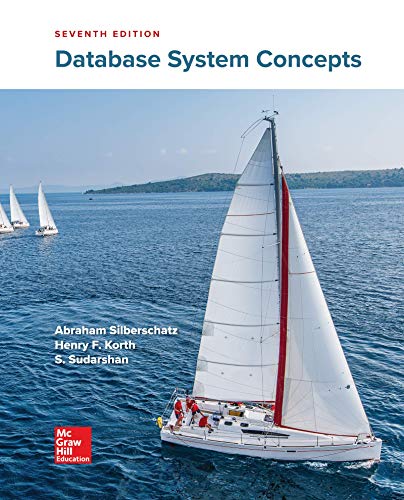
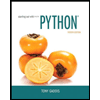
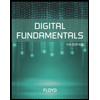
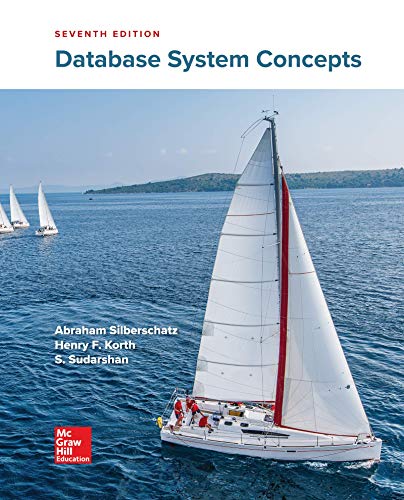
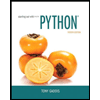
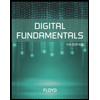
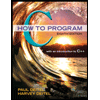
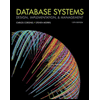
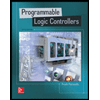