Write a program that reads a list of integers, and outputs whether the list contains all multiples of 10, no multiples of 10, or mixed values. Define a function named is_list_mult10 that takes a list as a parameter, and returns a boolean that represents whether the list contains all multiples of ten. Define a function named is_list_no_mult10 that takes a list as a parameter and returns a boolean that represents whether the list contains no multiples of ten. Then, write a main program that takes an integer, representing the size of the list, followed by the list values. The first integer is not in the list.
Write a
Then, write a main program that takes an integer, representing the size of the list, followed by the list values. The first integer is not in the list.
Ex: If the input is:
5 20 40 60 80 100the output is:
all multiples of 10Ex: If the input is:
5 11 -32 53 -74 95the output is:
no multiples of 10Ex: If the input is:
5 10 25 30 40 55the output is:
mixed valuesThe program must define and call the following two functions. is_list_mult10() returns true if all integers in the list are multiples of 10 and false otherwise. is_list_no_mult10() returns true if no integers in the list are multiples of 10 and false otherwise.
def is_list_mult10(my_list)
def is_list_no_mult10(my_list)
My Code
ef is_list_mult10(my_list):
for i in my_list:
if i%10 != 0:
return False
return True
def is_list_no_mult10(my_list):
for i in my_list:
if i%10 == 0:
return False
return True
num = int(input())
my_list = []
for i in range(0,num):
my_list.append(int(input()))
is_multiple = is_list_mult10(my_list)
is_not_Multiple = is_list_no_mult10(my_list)
if (is_multiple == True) and (is_not_Multiple == False):
print("all multiples of 10")
elif is_multiple == False and is_not_Multiple == True:
print("no multiples of 10")
else:
print("mixed values")
[Picture of What I have Right and Wrong]
![1:Compare output A
Your output
Input
2:Compare output A
Your output
4:Unit test
Input
3:Compare output
5:Unit test A
Input
Your output
5
20
40
60
80
100
all multiples of 10
5
11
-32
53
-74
95
no multiples of 10
5
WNP (n
10
(noon O
25
30
40
55
mixed values
is_list_mult10([10, 200, 4000])
is_list_no_mult10([9, 11, 13])
2/2
2/2
2/2
0/2
0/2](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F59491c6e-91a5-4fb5-8e7e-dce2244c96d7%2F11021030-3e4c-4f0b-8993-5a4211cb9783%2Flexqaul_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

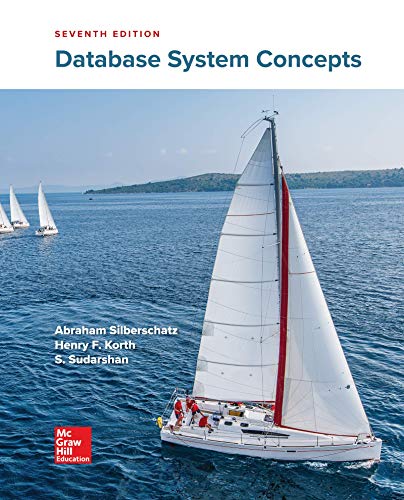
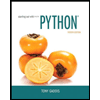
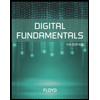
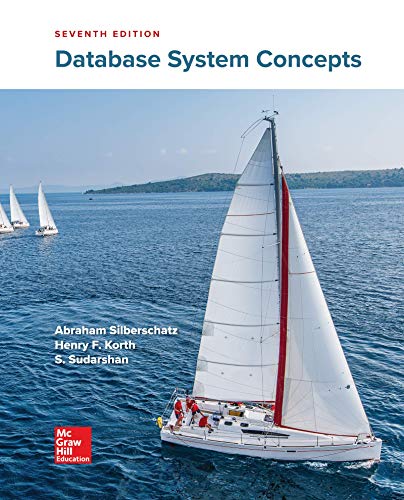
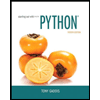
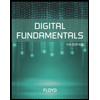
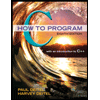
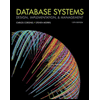
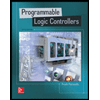