Write a program that reads an integer array all from the console and writes out the subsequence of the array that sums to the largest value. If there are more than one such subsequence, then the program should write out the subsequence that starts at the smallest position in the array and, if there are more than one subsequence of largest sum starting at the same smallest position, it should write out the shortest such subsequence. For example, suppose all-(1, 2, 3, -1) then the output would be Largest sum is 6 obtained from the subsequence [123] This is the correct answer because I other subsequences have a smaller sum. The set of subsequences of all is (1) (1.2), (1,2,3), (1.23-1), (21 (2.3). (2,3,-1) (3) (3.-1) (-1) As another example, if all-(-3, 3, -3, 3) then the output would be Largest sum is 3 obtained from the subsequence [3] In this case, the subsequences are (-3), (-3,3), (-3,3,-3), (-3,3,-3,3), (3), (3,-3), (3,-3,3), (-3,3), (3) While the subsequence (3,-3,3) also sums to 3 and starts at position 1 in the array, we write out the subsequence (3) since it is shorter. Use the template code below to read the size of the array N and the array elements from the console. (N should not be bigger than MAX SIZE but there is no need to write code to check for this condition). The Moodle checker expects that the output is exactly as shown above and in the examples below (in particular, ensure there is a space after [and a space before ] when writing out the subsequence). For example: Input Largest sum is 6 obtained from the subsequence [123] Largest sum is 3 obtained from the subsequence [3] 10-1-2-1-1-1-1-1-1-1-1 Largest sun is -1 obtained from the subsequence [ 1 ] 10 10 -2 -1 -1 -1-19-1-1 -1 Largest sun is 13 obtained from the subsequence [ 10 -2 -1 -1 -1 -19] Largest sum is obtained from the subsequence [] 4-33-33 Result 0 K
Write a program that reads an integer array all from the console and writes out the subsequence of the array that sums to the largest value. If there are more than one such subsequence, then the program should write out the subsequence that starts at the smallest position in the array and, if there are more than one subsequence of largest sum starting at the same smallest position, it should write out the shortest such subsequence. For example, suppose all-(1, 2, 3, -1) then the output would be Largest sum is 6 obtained from the subsequence [123] This is the correct answer because I other subsequences have a smaller sum. The set of subsequences of all is (1) (1.2), (1,2,3), (1.23-1), (21 (2.3). (2,3,-1) (3) (3.-1) (-1) As another example, if all-(-3, 3, -3, 3) then the output would be Largest sum is 3 obtained from the subsequence [3] In this case, the subsequences are (-3), (-3,3), (-3,3,-3), (-3,3,-3,3), (3), (3,-3), (3,-3,3), (-3,3), (3) While the subsequence (3,-3,3) also sums to 3 and starts at position 1 in the array, we write out the subsequence (3) since it is shorter. Use the template code below to read the size of the array N and the array elements from the console. (N should not be bigger than MAX SIZE but there is no need to write code to check for this condition). The Moodle checker expects that the output is exactly as shown above and in the examples below (in particular, ensure there is a space after [and a space before ] when writing out the subsequence). For example: Input Largest sum is 6 obtained from the subsequence [123] Largest sum is 3 obtained from the subsequence [3] 10-1-2-1-1-1-1-1-1-1-1 Largest sun is -1 obtained from the subsequence [ 1 ] 10 10 -2 -1 -1 -1-19-1-1 -1 Largest sun is 13 obtained from the subsequence [ 10 -2 -1 -1 -1 -19] Largest sum is obtained from the subsequence [] 4-33-33 Result 0 K
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 24PE
Related questions
Question
100%
Write a
![Write a program that reads an integer array all from the console and writes out the subsequence of the array that sums to the largest value. If there are more than one such subsequence, then the program should write out the subsequence that starts at the smallest
position in the array and, if there are more than one subsequence of largest sum starting at the same smallest position, it should write out the shortest such subsequence.
For example, suppose a[] = {1, 2, 3, -1) then the output would be
Largest sum is 6 obtained from the subsequence [123]
This is the correct answer because all other subsequences have a smaller sum. The set of subsequences of all is
(1), (1,2), (1,2,3), (1,2,3,-1), (2), (2,3), (2,3,-1), (3), (3, -1) (-1)
As another example, if all-(-3, 3, -3, 3) then the output would be
Largest sum is 3 obtained from the subsequence [ 3 ]
In this case, the subsequences are
(-3), (-3,3), (-3,3,-3), (-3,3,-3,3), (3), (3,-3), (3,-3,3), (-3,3), (3)
While the subsequence (3,-3,3) also sums to 3 and starts at position 1 in the array, we write out the subsequence (3) since it is shorter.
Use the template code below to read the size of the array N and the array elements from the console. (N should not be bigger than MAX SIZE but there is no need to write code to check for this condition).
The Moodle checker expects that the output is exactly as shown above and in the examples below (in particular, ensure there is a space after [and a space before ] when writing out the subsequence).
For example:
Input
4123-1
4-3 3-3 3
10 -1 -2 -1 -1-1-1-1-1-1-1
10 10 -2 -1 -1 -1 -19 -1 -1 -1
@
Result
Largest sun is 6 obtained from the subsequence [123]
Largest sum is 3 obtained from the subsequence [ 3 ]
Largest sum is -1 obtained from the subsequence [ 1 ]
Largest sum is 13 obtained from the subsequence [ 10 -2-1-1-1-19 1
Largest sum is e obtained from the subsequence [ ]
K](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fde139120-ad09-48a8-a33f-c24049aab818%2F10a58eed-fba2-4412-8bb1-463b4792ed9f%2Fbfvd9er_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Write a program that reads an integer array all from the console and writes out the subsequence of the array that sums to the largest value. If there are more than one such subsequence, then the program should write out the subsequence that starts at the smallest
position in the array and, if there are more than one subsequence of largest sum starting at the same smallest position, it should write out the shortest such subsequence.
For example, suppose a[] = {1, 2, 3, -1) then the output would be
Largest sum is 6 obtained from the subsequence [123]
This is the correct answer because all other subsequences have a smaller sum. The set of subsequences of all is
(1), (1,2), (1,2,3), (1,2,3,-1), (2), (2,3), (2,3,-1), (3), (3, -1) (-1)
As another example, if all-(-3, 3, -3, 3) then the output would be
Largest sum is 3 obtained from the subsequence [ 3 ]
In this case, the subsequences are
(-3), (-3,3), (-3,3,-3), (-3,3,-3,3), (3), (3,-3), (3,-3,3), (-3,3), (3)
While the subsequence (3,-3,3) also sums to 3 and starts at position 1 in the array, we write out the subsequence (3) since it is shorter.
Use the template code below to read the size of the array N and the array elements from the console. (N should not be bigger than MAX SIZE but there is no need to write code to check for this condition).
The Moodle checker expects that the output is exactly as shown above and in the examples below (in particular, ensure there is a space after [and a space before ] when writing out the subsequence).
For example:
Input
4123-1
4-3 3-3 3
10 -1 -2 -1 -1-1-1-1-1-1-1
10 10 -2 -1 -1 -1 -19 -1 -1 -1
@
Result
Largest sun is 6 obtained from the subsequence [123]
Largest sum is 3 obtained from the subsequence [ 3 ]
Largest sum is -1 obtained from the subsequence [ 1 ]
Largest sum is 13 obtained from the subsequence [ 10 -2-1-1-1-19 1
Largest sum is e obtained from the subsequence [ ]
K
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
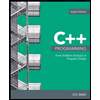
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
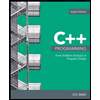
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage