Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1 and at most 20. The program performs the following tasks: • Read the file name of the tsv file from the user. Assume the file name has a maximum of 25 characters. • Open the tsv file and read the student information. Assume each last name or first name has a maximum of 25 characters. • Compute the average exam score of each student. Assign a letter grade to each student based on the average exam score in the following scale: • A: 90= 2 3 int main (void) { 4 9 10 11 Tyrese 88 61 36 Brenda 90 86 45 12 13 14} 15 /* TODO: Declare any necessary variables here. */ /* TODO: Read a file name from the user and read the tsv file here. */ /* TODO: Compute student grades and exam averages, then output results to a text file here. */ return 0;
Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1 and at most 20. The program performs the following tasks: • Read the file name of the tsv file from the user. Assume the file name has a maximum of 25 characters. • Open the tsv file and read the student information. Assume each last name or first name has a maximum of 25 characters. • Compute the average exam score of each student. Assign a letter grade to each student based on the average exam score in the following scale: • A: 90= 2 3 int main (void) { 4 9 10 11 Tyrese 88 61 36 Brenda 90 86 45 12 13 14} 15 /* TODO: Declare any necessary variables here. */ /* TODO: Read a file name from the user and read the tsv file here. */ /* TODO: Compute student grades and exam averages, then output results to a text file here. */ return 0;
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
1st Edition
ISBN:9780357392676
Author:FREUND, Steven
Publisher:FREUND, Steven
Chapter8: Working With Trendlines, Pivottables, Pivotcharts, And Slicers
Section: Chapter Questions
Problem 3EYK
Related questions
Question
(C Language) Given a text file containing the availability of food items, write a
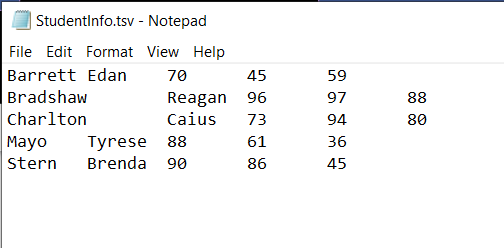
Transcribed Image Text:StudentInfo.tsv- Notepad
File Edit Format View Help
Barrett Edan
70
Bradshaw
Charlton
Reagan
Caius
Mayo
Tyrese
88
Stern Brenda 90
45
96
73
61
86
59
97
94
36
45
88
80
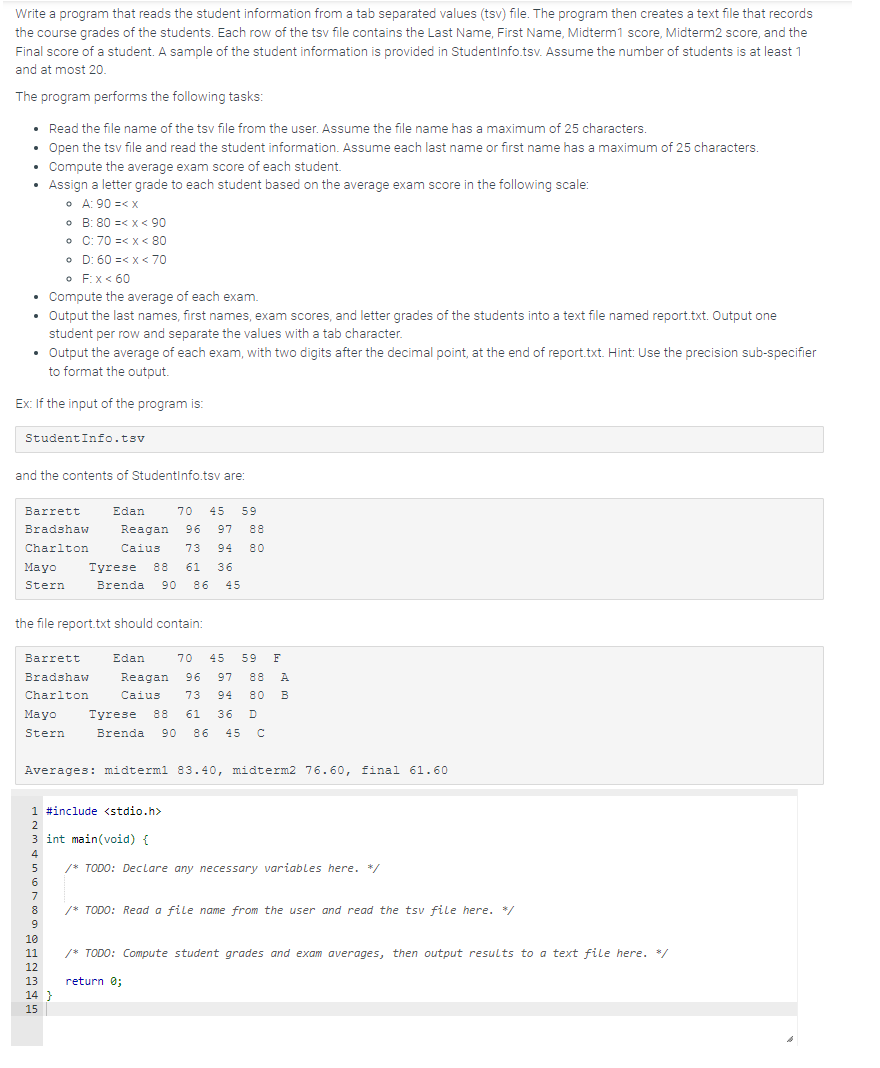
Transcribed Image Text:Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records
the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the
Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1
and at most 20.
The program performs the following tasks:
• Read the file name of the tsv file from the user. Assume the file name has a maximum of 25 characters.
• Open the tsv file and read the student information. Assume each last name or first name has a maximum of 25 characters.
• Compute the average exam score of each student.
• Assign a letter grade to each student based on the average exam score in the following scale:
• A: 90 =< x
• F: x < 60
• Compute the average of each exam.
Output the last names, first names, exam scores, and letter grades of the students into a text file named report.txt. Output one
student per row and separate the values with a tab character.
• Output the average of each exam, with two digits after the decimal point, at the end of report.txt. Hint: Use the precision sub-specifier
to format the output.
Ex: If the input of the program is:
StudentInfo.tsv
and the contents of Studentinfo.tsv are:
Mayo
Stern
•
•
•
Barrett
Bradshaw
Charlton
B: 80 < x < 90
C: 70 =< x < 80
D: 60 =< x < 70
Mayo
Stern
Barrett
Bradshaw
Charlton
the file report.txt should contain:
4
5
6
7
8
9
Edan 70 45 59
Reagan 96 97 88
Caius 73 94 80
10
11
12
13
14}
15
Tyrese 88 61 36
Brenda 90 86 45
Averages: midterm1 83.40, midterm2 76.60, final 61.60
1 #include <stdio.h>
2
3 int main (void) {
Edan 70 45 59 F
Reagan 96 97 88 A
Caius 73 94 80 B
Tyrese 88 61 36 D
Brenda 90 86 45 C
/* TODO: Declare any necessary variables here. */
/* TODO: Read a file name from the user and read the tsv file here. */
/* TODO: Compute student grades and exam averages, then output results to a text file here. */
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
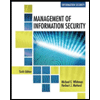
Management Of Information Security
Computer Science
ISBN:
9781337405713
Author:
WHITMAN, Michael.
Publisher:
Cengage Learning,
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
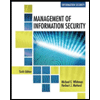
Management Of Information Security
Computer Science
ISBN:
9781337405713
Author:
WHITMAN, Michael.
Publisher:
Cengage Learning,
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
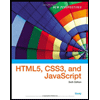
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning