Write a program that uses a two-dimensional array to store the highest and lowest temperatures for each month of the year. The program should output the average high, average low, and the highest and lowest temperatures for the year. Your program must consist of the following functions: Function getData: This function reads and stores data in the two- dimensional array. а. Function averageHigh: This function calculates and returns the aver- age high temperature for the year. b. Function averageLow: This function calculates and returns the average low temperature for the year. c. Function indexHighTemp: This function returns the index of the highest high temperature in the array. d. Function indexLowTemp: This function returns the index of the lowest low temperature in the array. е.
Can this code be inplimented in C++ and look exactly like the output file. All of the output should be displayed in the outfile called weatherout.txt and all of the input temperatures should be read and used from the input file called weather.txt. The temperatures should be displayed in two colums like shown in the sample output.
I have included the main function and skeleton of the solution that can be used to compelete the code and guide in the right direction.
const int NO_OF_MONTHS = 12;
void getData(int twoDim[][2], int rows);
int averageHigh(int twoDim[][2], int rows);
int averageLow(int twoDim[][2], int rows);
int indexHighTemp(int twoDim[][2], int rows);
int indexLowTemp(int twoDim[][2], int rows);
void display(int twoDim[][2], int);
ifstream inFile("weather.txt");
ofstream outFile("Wearther.out");
int main()
{
int hiLowArray[NO_OF_MONTHS][2];
int indexHigh;
int indexLow;
getData(hiLowArray, NO_OF_MONTHS);
display(hiLowArray,NO_OF_MONTHS);
cout << "\nAverage high temperature: "
<< averageHigh(hiLowArray, NO_OF_MONTHS) << endl;
outFile << "\nAverage high temperature: "
<< averageHigh(hiLowArray, NO_OF_MONTHS) << endl;
cout << "\nAverage low temperature: "
<< averageLow(hiLowArray, NO_OF_MONTHS) << endl;
outFile << "\nAverage low temperature: "
<< averageLow(hiLowArray, NO_OF_MONTHS) << endl;
indexHigh = indexHighTemp(hiLowArray, NO_OF_MONTHS);
cout << "\nHighest temperature: " << hiLowArray[indexHigh][0] << endl;
outFile << "\nHighest temperature: " << hiLowArray[indexHigh][0] << endl;
indexLow = indexLowTemp(hiLowArray, NO_OF_MONTHS);
cout << "\nLowest temperature: " << hiLowArray[indexLow][1] << endl;
outFile << "\nLowest temperature: " << hiLowArray[indexLow][1] << endl;
return 0;
}
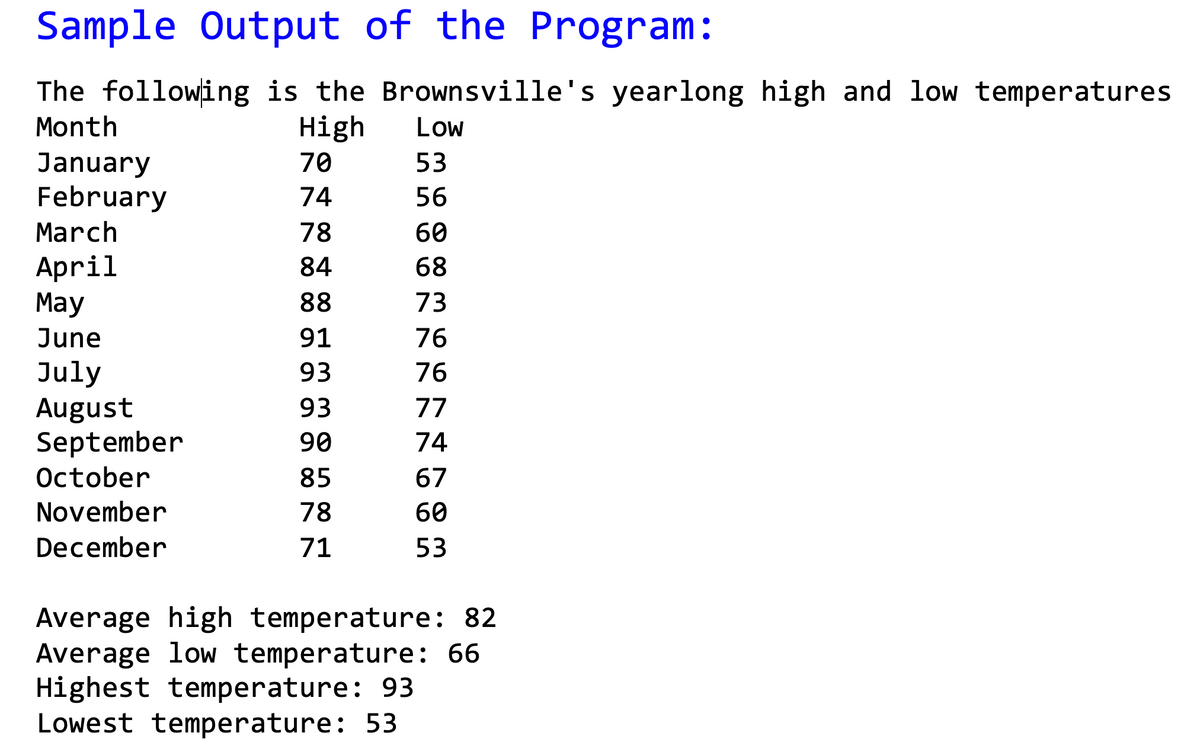
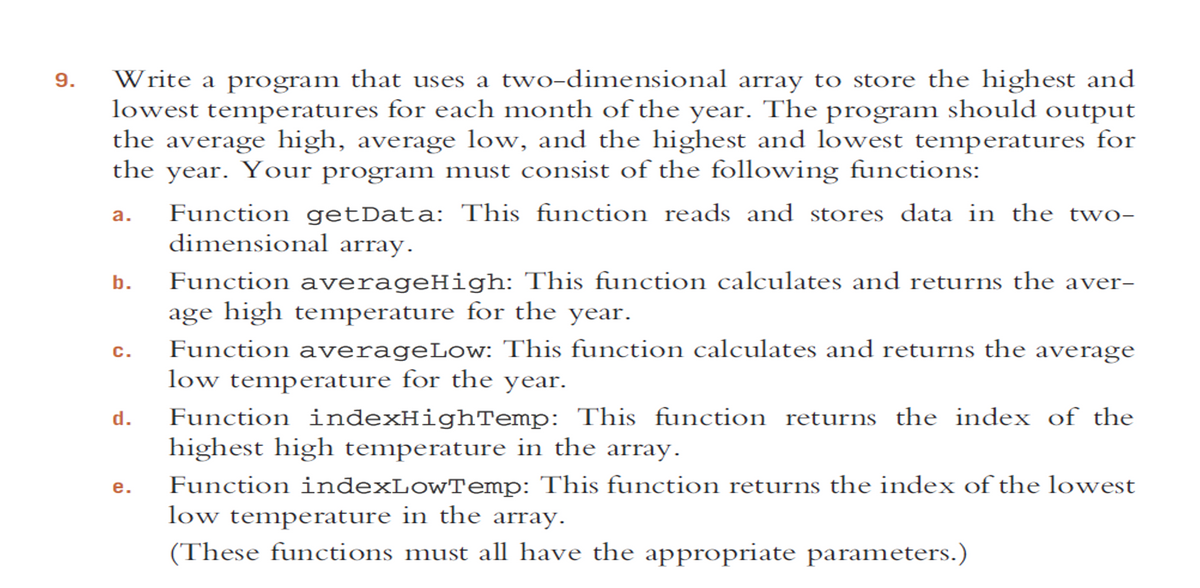

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

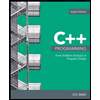
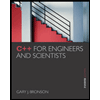
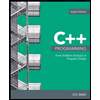
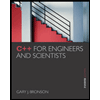