Write a program to calculate total cost of the event based on the type of event and display details using Abstract class and method. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, attribute names and method names should be the same as specified in the problem statement. Consider an abstract class called Event with following protected attributes. Attributes Datatype name String detail String type String organiser String Prototype for the parametrized constructor, Event(String name, String detail, String type, String organiser) Include appropriate getters and setters Include the following abstract method in the class Event. Method Name Description abstract Double calculateAmount() an abstract method Consider a class named Exhibition which extends Event class with the following private attributes Attributes Datatype noOfStalls Integer rentPerStall Double Prototype for the parametrized constructor, public Exhibition(String name, String detail, String type, String organiser, Integer noOfStalls,Double rentPerStall) Include appropriate getters and setters Use super( ) to call and assign values in base class constructor. Include the following abstract method in the class Exhibition. Method Name Description Double calculateAmount () This method returns the product of noOfStalls and rentPerStall Consider a class named StageEvent which extends Event class with the following private attributes. Attribute Datatype noOfShows Integer costPerShow Double Prototype for the parametrized constructor, public StageEvent(String name, String detail, String type, String organiser, Integer noOfShows,Double costPerShow) Include appropriate getters and setters Use super( ) to call and assign values in base class constructor. Include the following abstract method in the class StageEvent. Method Name Description Double calculateAmount() This method returns the product of noOfShows and costPerShow Consider a driver class called Main. In the main method, obtain input from the user and create objects accordingly. The link to download the template code is given below Code Template Input format: Input format for Exhibition is in the CSV format (name,detail,type,organiser,noOfStalls,rentPerStall) Input format for StageEvent is in the CSV format (name,detail,type,organiser,noOfShows,costPerShow) Output format: Print "Invalid choice" if the input is invalid to our application and terminate. Display one digit after the decimal point for Double datatype. Refer to sample Input and Output for formatting specifications. [All text in bold corresponds to the input and rest corresponds to output] Sample Input and output 1: Enter your choice 1.Exhibition 2.StageEvent 1 Enter the details in CSV format Book expo,Special sale,Academics,Martin,100,1000 Exhibition Details Event Name:Book expo Detail:Special sale Type:Academics Organiser Name:Martin Total Cost:100000.0 Sample Input and Output 2: Enter your choice 1.Exhibition 2.StageEvent 2 Enter the details in CSV format JJ magic show,Comedy magic,Entertainment,Steffania,5,1000 Stage Event Details Event Name:JJ magic show Detail:Comedy magic Type:Entertainment Organiser Name:Steffania Total Cost:5000.0 Sample Input and Output 3: Enter your choice 1.Exhibition 2.StageEvent 3 Invalid choice
Write a program to calculate total cost of the event based on the type of event and display details using Abstract class and method. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, attribute names and method names should be the same as specified in the problem statement. Consider an abstract class called Event with following protected attributes. Attributes Datatype name String detail String type String organiser String Prototype for the parametrized constructor, Event(String name, String detail, String type, String organiser) Include appropriate getters and setters Include the following abstract method in the class Event. Method Name Description abstract Double calculateAmount() an abstract method Consider a class named Exhibition which extends Event class with the following private attributes Attributes Datatype noOfStalls Integer rentPerStall Double Prototype for the parametrized constructor, public Exhibition(String name, String detail, String type, String organiser, Integer noOfStalls,Double rentPerStall) Include appropriate getters and setters Use super( ) to call and assign values in base class constructor. Include the following abstract method in the class Exhibition. Method Name Description Double calculateAmount () This method returns the product of noOfStalls and rentPerStall Consider a class named StageEvent which extends Event class with the following private attributes. Attribute Datatype noOfShows Integer costPerShow Double Prototype for the parametrized constructor, public StageEvent(String name, String detail, String type, String organiser, Integer noOfShows,Double costPerShow) Include appropriate getters and setters Use super( ) to call and assign values in base class constructor. Include the following abstract method in the class StageEvent. Method Name Description Double calculateAmount() This method returns the product of noOfShows and costPerShow Consider a driver class called Main. In the main method, obtain input from the user and create objects accordingly. The link to download the template code is given below Code Template Input format: Input format for Exhibition is in the CSV format (name,detail,type,organiser,noOfStalls,rentPerStall) Input format for StageEvent is in the CSV format (name,detail,type,organiser,noOfShows,costPerShow) Output format: Print "Invalid choice" if the input is invalid to our application and terminate. Display one digit after the decimal point for Double datatype. Refer to sample Input and Output for formatting specifications. [All text in bold corresponds to the input and rest corresponds to output] Sample Input and output 1: Enter your choice 1.Exhibition 2.StageEvent 1 Enter the details in CSV format Book expo,Special sale,Academics,Martin,100,1000 Exhibition Details Event Name:Book expo Detail:Special sale Type:Academics Organiser Name:Martin Total Cost:100000.0 Sample Input and Output 2: Enter your choice 1.Exhibition 2.StageEvent 2 Enter the details in CSV format JJ magic show,Comedy magic,Entertainment,Steffania,5,1000 Stage Event Details Event Name:JJ magic show Detail:Comedy magic Type:Entertainment Organiser Name:Steffania Total Cost:5000.0 Sample Input and Output 3: Enter your choice 1.Exhibition 2.StageEvent 3 Invalid choice
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Write a program to calculate total cost of the event based on the type of event and display details using Abstract class and method.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, attribute names and method names should be the same as specified in the problem statement.
Consider an abstract class called Event with following protected attributes.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, attribute names and method names should be the same as specified in the problem statement.
Consider an abstract class called Event with following protected attributes.
Attributes | Datatype |
name | String |
detail | String |
type | String |
organiser | String |
Prototype for the parametrized constructor, Event(String name, String detail, String type, String organiser)
Include appropriate getters and setters
Include appropriate getters and setters
Include the following abstract method in the class Event.
Method Name | Description |
abstract Double calculateAmount() | an abstract method |
Consider a class named Exhibition which extends Event class with the following private attributes
Attributes | Datatype |
noOfStalls | Integer |
rentPerStall | Double |
Prototype for the parametrized constructor,
public Exhibition(String name, String detail, String type, String organiser, Integer noOfStalls,Double rentPerStall)
Include appropriate getters and setters
public Exhibition(String name, String detail, String type, String organiser, Integer noOfStalls,Double rentPerStall)
Include appropriate getters and setters
Use super( ) to call and assign values in base class constructor.
Include the following abstract method in the class Exhibition.
Method Name | Description |
Double calculateAmount () | This method returns the product of noOfStalls and rentPerStall |
Consider a class named StageEvent which extends Event class with the following private attributes.
Attribute | Datatype |
noOfShows | Integer |
costPerShow | Double |
Prototype for the parametrized constructor,
public StageEvent(String name, String detail, String type, String organiser, Integer noOfShows,Double costPerShow)
Include appropriate getters and setters
Use super( ) to call and assign values in base class constructor.
Include the following abstract method in the class StageEvent.
Method Name | Description |
Double calculateAmount() | This method returns the product of noOfShows and costPerShow |
Consider a driver class called Main. In the main method, obtain input from the user and create objects accordingly.
The link to download the template code is given below
Code Template
Input format:
Input format for Exhibition is in the CSV format (name,detail,type,organiser,noOfStalls,rentPerStall)
Input format for StageEvent is in the CSV format (name,detail,type,organiser,noOfShows,costPerShow)
Output format:
Print "Invalid choice" if the input is invalid to our application and terminate.
Display one digit after the decimal point for Double datatype.
Refer to sample Input and Output for formatting specifications.
[All text in bold corresponds to the input and rest corresponds to output]
Sample Input and output 1:
Enter your choice
1.Exhibition
2.StageEvent
1
Enter the details in CSV format
Book expo,Special sale,Academics,Martin,100,1000
Exhibition Details
Event Name:Book expo
Detail:Special sale
Type:Academics
Organiser Name:Martin
Total Cost:100000.0
Sample Input and Output 2:
Enter your choice
1.Exhibition
2.StageEvent
2
Enter the details in CSV format
JJ magic show,Comedy magic,Entertainment,Steffania,5,1000
Stage Event Details
Event Name:JJ magic show
Detail:Comedy magic
Type:Entertainment
Organiser Name:Steffania
Total Cost:5000.0
Sample Input and Output 3:
Enter your choice
1.Exhibition
2.StageEvent
3
Invalid choiceExpert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Recommended textbooks for you
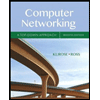
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
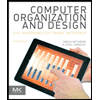
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
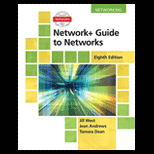
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
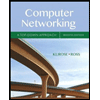
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
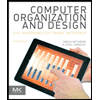
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
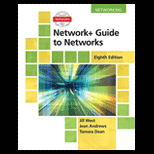
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
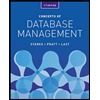
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
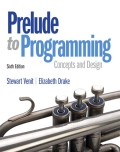
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
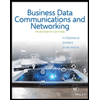
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY