Write a program to finish the following tasks: • It allows the user to input a value to determine the size of an int array • Fill the array with random numbers (there is no requirement on the range, just use what ever rand() gives to you) • Find out the largest number in the array and print it out
Write a program to finish the following tasks: • It allows the user to input a value to determine the size of an int array • Fill the array with random numbers (there is no requirement on the range, just use what ever rand() gives to you) • Find out the largest number in the array and print it out
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.1: One-dimensional Arrays
Problem 7E: (Practice) Write a program to input eight integer numbers in an array named temp. As each number is...
Related questions
Question
C++

Transcribed Image Text:Write a program to finish the following tasks:
• It allows the user to input a value to determine the size of an int array
• Fill the array with random numbers (there is no requirement on the range, just use what ever randO gives to you)
• Find out the largest number in the array and print it out
Expert Solution

Code:
#include <iostream>
#include <stdlib.h>
#include <time.h>
using namespace std;
int main() {
int n;
cout << "Enter the size of the array: ";
cin >> n;
int array[n];
srand(time(0));
for(int i = 0; i < n; i++)
array[i] = rand();
int max = array[0];
for(int i = 0; i < n; i++) {
if(max < array[i])
max = array[i];
}
cout << "\nLargest number: " << max << endl;
return 0;
}
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
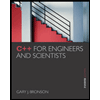
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
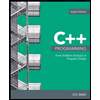
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
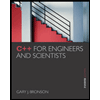
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
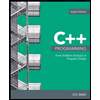
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
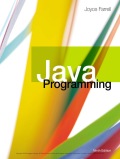
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT