Write a program to simulate a Banking Application with the following functionality: Setup an Account Holders Profile with Name, Address, phone number and e-mail as user inputs Open an account - Checking and Savings Deposit Money into Checking or Savings account as per users preference Withdraw Money from Checking or Savings account as per users preference Technical Requirements: Use I/O methods, if statements, switch statements, while, do-while and for loops, inputs and outputs and UTIL scanner methods
Write a program to simulate a Banking Application with the following functionality: Setup an Account Holders Profile with Name, Address, phone number and e-mail as user inputs Open an account - Checking and Savings Deposit Money into Checking or Savings account as per users preference Withdraw Money from Checking or Savings account as per users preference Technical Requirements: Use I/O methods, if statements, switch statements, while, do-while and for loops, inputs and outputs and UTIL scanner methods
Chapter1: Creating Java Programs
Section: Chapter Questions
Problem 18RQ
Related questions
Question
100%
Write a program to simulate a Banking Application with the following functionality: Setup an Account Holders Profile with Name, Address, phone number and e-mail as user inputs Open an account - Checking and Savings Deposit Money into Checking or Savings account as per users preference Withdraw Money from Checking or Savings account as per users preference Technical Requirements: Use I/O methods, if statements, switch statements, while, do-while and for loops, inputs and outputs and UTIL scanner methods
Expert Solution

Step 1
The program is written in java
import java.io.*;
import java.lang.*;
import java.util.Scanner;
import java.util.Random;
class bank{
public String Name;
public String Address;
publiclong phone;
public String email;
publiclong acc_no;
publicfloat checking_money;
publicfloat savings_money;
bank(String na,String adr,long ph, String em)
{
Random random = new Random();
this.Name=na;
this.Address=adr;
this.phone=ph;
this.email=em;
this.acc_no=Math.abs(random.nextLong());
this.checking_money=0;
this.savings_money=0;
System.out.println("Account created successfully");
display();
}
publicvoid display()
{
System.out.println("Name: "+this.Name+"\nAddress: "+this.Address+"\nphone: "+this.phone+"\nemail: "+this.email+"\nAccount number: "+this.acc_no+"\nBalance in checking account: "+this.checking_money+"\nBalance in savings account: "+this.savings_money);
}
publicvoid withdraw(float m,int i)
{
if(i==1)
{
if(this.checking_money>m)
{
this.checking_money=this.checking_money-m;
System.out.println("Successfully withdrawed money");
display();
}
else{
System.out.println("insufficient balance");
}
}
else{
if(this.savings_money>m)
{
this.savings_money=this.savings_money-m;
System.out.println("Successfully withdrawed money");
display();
}
else{
System.out.println("insufficient balance");
}
}
}
publicvoid Deposit(float m,int i)
{
if(i==1)
{
this.checking_money=this.checking_money+m;
}
else{
this.savings_money=this.savings_money+m;
}
System.out.println("Successfully deposited money");
display();
}
}
public class Main
{
public static void main(String[] args) {
int ch,i=0,n;
String name,addr,email;
long phone,accno;
float amount;
bank[] b=new bank[10];
Scanner s=new Scanner(System.in);
do{
System.out.println("1.Open account\n2.Deposit Money\n3.Withdraw money\n4.exit\nEnter your choice");
ch=s.nextInt();
switch(ch)
{
case 1:System.out.println("Enter details\n");
s.nextLine();
System.out.println("Enter your name ");
name=s.nextLine();
System.out.println("Enter your address ");
addr=s.nextLine();
System.out.println("Enter your phone number ");
phone=s.nextLong();
s.nextLine();
System.out.println("Enter your email ");
email=s.nextLine();
b[i]=new bank(name,addr,phone,email);
i++;
break;
case 2:System.out.println("Enter the account number: ");
accno=s.nextLong();
for(int j=0;j<i;j++)
{
if(b[j].acc_no==accno)
{
System.out.println("Enter the amount to Deposit");
amount=s.nextFloat();
System.out.println("Enter the type of account\n1.Checking\n2.Savings ");
n=s.nextInt();
b[j].Deposit(amount,n);
}
}
break;
case3: System.out.println("Enter the account number: ");
accno=s.nextLong();
for(int j=0;j<i;j++)
{
if(b[j].acc_no==accno)
{
System.out.println("Enter the amount to withdraw ");
amount=s.nextFloat();
System.out.println("Enter the type of acount\n1.Checking\n2.Savings ");
n=s.nextInt();
b[j].withdraw(amount,n);
}
}
break;
}
}while(ch!=4);
}
}
Step by step
Solved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
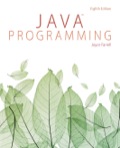
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
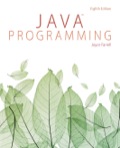
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT