Write a program to solve quadratic equations for real roots. No need to use imaginary numbers. ax^2 +bx +c= 0 Requirements: -Define an array of type double with size 3 in main() to store the 3 coefficients. -Read the 3 coefficients from user input. -Create the function prototype and definition for the function getRootCount(discriminant, coefficients) Parameters: discriminant: output to be calculated by the function coefficients: input array of 3 coefficients Return: number of roots: 0, 1, or 2
Write a
ax^2 +bx +c= 0
Requirements:
-Define an array of type double with size 3 in main() to store the 3 coefficients.
-Read the 3 coefficients from user input.
-Create the function prototype and definition for the function
getRootCount(discriminant, coefficients)
Parameters:
discriminant: output to be calculated by the function
coefficients: input array of 3 coefficients
Return: number of roots: 0, 1, or 2
-Create the function prototype and definition for a function to determine the numbers of roots:
getRootCount(discriminant, coefficients);
Parameters:
discriminant: output value to be calculated by the function (reference parameter)
coefficients: input array of 3 coefficients
Return: number of roots: 0, 1, or 2 (int)
-Create the function prototype and definition for a function to solve equations and get the root(s):
solveEquation(discriminant, coefficients, roots);
Parameters:
discriminant: input value to the function.
The discriminant is calculated by getRootCount(), so main() gets it and passes to this function.
coefficients: input array of 3 coefficients
roots: output array of roots.
Size of roots array is either 1 or 2, as determined by main() after calling getRootCount()
Return: none (void)
-If the equation is valid, the main() function calls getRootCount() to determine the number of roots and also to get the discriminant calculated by this function.
-If there is at least one root, then main() defines an array of roots with the exact size (based on count of roots), then it calls solveEquation().
-Finally main() displays the one or two roots in the roots array, or displays a message if there is no root.
-You must use the following given main() code to test your 2 functions first before adding a loop to main():
int main()
{
int const SIZE = 3;
double coefficients[SIZE];
double discriminant;
cout << "This program solves quadratic formulas\n";
cout << "Enter 3 coefficients separate by spaces: ";
cin >> coefficients[0] >> coefficients[1] >> coefficients[2] ;
if (coefficients[0] == 0)
cout << "First coefficient cannot be 0.";
else
{
int rootCount = getRootCount(discriminant, coefficients);
if (rootCount == 0)
cout << "There is no root" << endl;
else
{
double roots[rootCount];
solveEquation(discriminant, coefficients, roots);
if (rootCount == 1)
cout << "There is one root: " << roots[0] << endl;
else
cout << "There are 2 roots: " << roots[0] << " " << roots[1] << endl;
}
}
}
Sample outputs with given main code and different coefficient inputs:
This program solves quadratic formulas
Enter 3 coefficients separate by spaces: 1 -8 5
There are 2 roots: 7.31662 0.683375
This program solves quadratic formulas
Enter 3 coefficients separate by spaces: 1 2 1
There is one root: -1
This program solves quadratic formulas
Enter 3 coefficients separate by spaces: 5 20 32
There is no root
This program solves quadratic formulas
Enter 3 coefficients separate by spaces: 0 4 5
First coefficient cannot be 0.
If your 2 functions do not work with the given main code, do not bother to add more code to main() in the next step.
-After checking that your functions works with the given main() code, then add the most efficient loop in main() to let the program accept multiple equations and stop only when all 3 coefficients are 0.
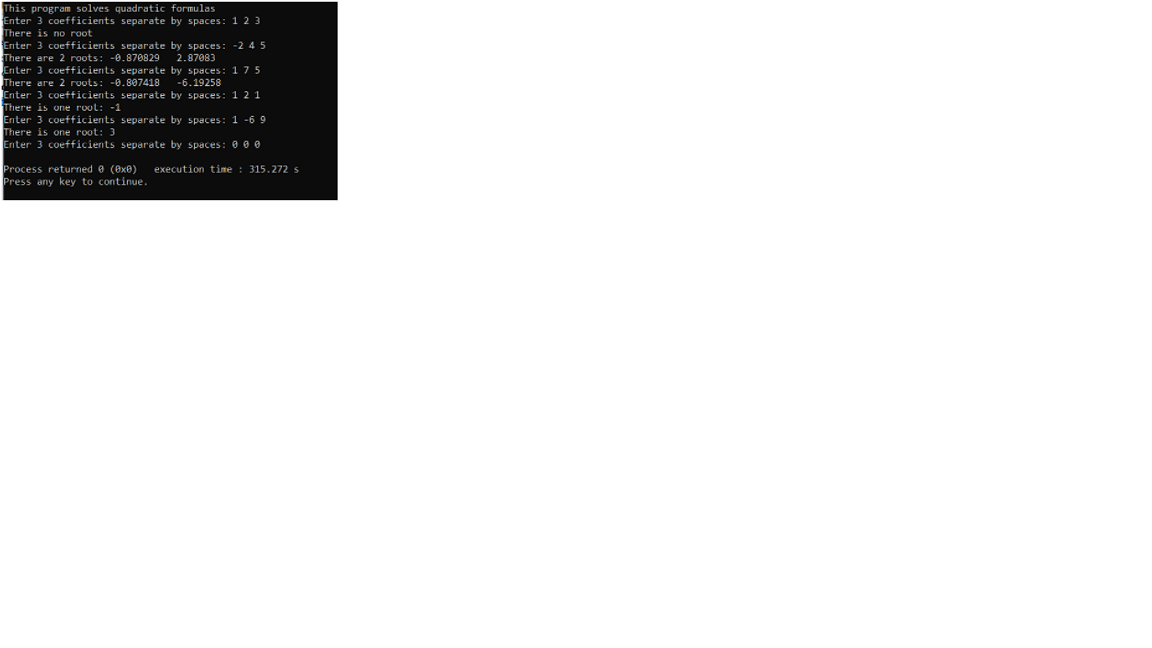

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

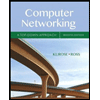
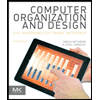
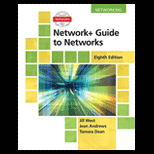
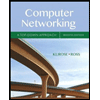
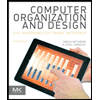
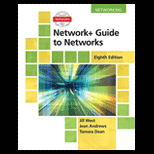
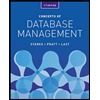
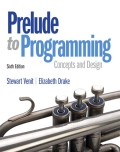
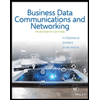