Write a Python program for applying CNN with considering the following requirements: Shuffle the cifar 10 training set x_train( each input with corresponding label in y_train ) Select 2500 images and you must ensure that each class must contain at least 180 samples Apply CNN that keeps noisy examples. Overall, the pseudocode of CNN is as follows: The code must contain at least one lambda expression The code must contain at one comprehension list It is Not allowed to use the numpy library. Euclidean distance is the distance between two samples in Euclidean space. The formula can be expressed as
Write a Python program for applying CNN with considering the following requirements: Shuffle the cifar 10 training set x_train( each input with corresponding label in y_train ) Select 2500 images and you must ensure that each class must contain at least 180 samples Apply CNN that keeps noisy examples. Overall, the pseudocode of CNN is as follows: The code must contain at least one lambda expression The code must contain at one comprehension list It is Not allowed to use the numpy library. Euclidean distance is the distance between two samples in Euclidean space. The formula can be expressed as
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a Python program for applying CNN with considering the following requirements:
- Shuffle the cifar 10 training set x_train( each input with corresponding label in y_train )
- Select 2500 images and you must ensure that each class must contain at least 180 samples
- Apply CNN that keeps noisy examples. Overall, the pseudocode of CNN is as follows:
- The code must contain at least one lambda expression
- The code must contain at one comprehension list
- It is Not allowed to use the numpy library.
Euclidean distance is the distance between two samples in Euclidean space. The formula can be expressed as:
![+ X Python Tryit Editor
W X Python Tryit Editor
W X Python Operators
X Answered: You rece b
X CSC 605_HW1 - Jup E
X Downloads/csc/
f
localhost:8888/notebooks/Downloads/csc/CSC%20605_HW1.ipynb#2)-Convert-the-following-function-to-lambda-expression O
->
قائمة القراءة
SQL for Beginners:. û
B مرحبّار فياض - . .Black
Google hiljs A
YouTube
Gmail M
التطبيقات
قائمة القراءة
Cjupyter CSC .•_HW' Last Checkpoint: ácll io uual (autosaved)
Logout
File
Edit
View
Insert
Cell
Kernel
Widgets
Help
Python 3 O
Not Trusted
+
Run
Markdown
Condensed Nearest Neighbor (CNN)
Data reduction techniques aim to simplify the training data by removing noisy and redundant data, so that, machine learning algorithms can learn faster with
little or no performance degradation, as if the entire training set T is used.
CNN was the first data reduction Algorithm. This algorithm is an incremental method that starts with adding one example of each class to the subset S
randomly from training set T. Then, for each example x in T is classified using the examples in S. if the example x is incorrectly classified, it will be added to
S. This guarantees all instances in T are classified correctly. Based on this criterion, noisy examples will be retained because they are commonly classified
wrongly by their k – NN (whrere k = 1)
The CIFAR-10 dataset The CIFAR-10 dataset consists of 60000 32x32 colour images in 10 classes, with 6000 images per class. There are 50000 training
images and 10000 test images.
In order to load CIFAR-10 dataset, First, download tensorflow library using the following command:
install tensorflow
Then, use the following code:
In [63]: import tensorflow
import cv2
from tensorflow import keras
from PIL import Image
import numpy as p
(x_train, y_train), (_, _) = tf.keras.datasets.cifar10.load_data()
x_train = [cv2.cvtColor(image, cv2.COLOR_BGR2GRAY).flatten().tolist() for image in x_train]
##x_train=np.asarray(x_train)
print(len(x_train))
print(len(x_train[0])) # 32*32
<IPython.core.display.Javascript object>
50000
1024
3:03 PM
O Type here to search
96°F
O C 1) ENG
9/27/2021
()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F723158fd-7202-4703-8c7a-3adefb105dcc%2Fa98a63c1-5664-4458-a809-739892cb86f4%2Fx86kc6u_processed.png&w=3840&q=75)
Transcribed Image Text:+ X Python Tryit Editor
W X Python Tryit Editor
W X Python Operators
X Answered: You rece b
X CSC 605_HW1 - Jup E
X Downloads/csc/
f
localhost:8888/notebooks/Downloads/csc/CSC%20605_HW1.ipynb#2)-Convert-the-following-function-to-lambda-expression O
->
قائمة القراءة
SQL for Beginners:. û
B مرحبّار فياض - . .Black
Google hiljs A
YouTube
Gmail M
التطبيقات
قائمة القراءة
Cjupyter CSC .•_HW' Last Checkpoint: ácll io uual (autosaved)
Logout
File
Edit
View
Insert
Cell
Kernel
Widgets
Help
Python 3 O
Not Trusted
+
Run
Markdown
Condensed Nearest Neighbor (CNN)
Data reduction techniques aim to simplify the training data by removing noisy and redundant data, so that, machine learning algorithms can learn faster with
little or no performance degradation, as if the entire training set T is used.
CNN was the first data reduction Algorithm. This algorithm is an incremental method that starts with adding one example of each class to the subset S
randomly from training set T. Then, for each example x in T is classified using the examples in S. if the example x is incorrectly classified, it will be added to
S. This guarantees all instances in T are classified correctly. Based on this criterion, noisy examples will be retained because they are commonly classified
wrongly by their k – NN (whrere k = 1)
The CIFAR-10 dataset The CIFAR-10 dataset consists of 60000 32x32 colour images in 10 classes, with 6000 images per class. There are 50000 training
images and 10000 test images.
In order to load CIFAR-10 dataset, First, download tensorflow library using the following command:
install tensorflow
Then, use the following code:
In [63]: import tensorflow
import cv2
from tensorflow import keras
from PIL import Image
import numpy as p
(x_train, y_train), (_, _) = tf.keras.datasets.cifar10.load_data()
x_train = [cv2.cvtColor(image, cv2.COLOR_BGR2GRAY).flatten().tolist() for image in x_train]
##x_train=np.asarray(x_train)
print(len(x_train))
print(len(x_train[0])) # 32*32
<IPython.core.display.Javascript object>
50000
1024
3:03 PM
O Type here to search
96°F
O C 1) ENG
9/27/2021
()
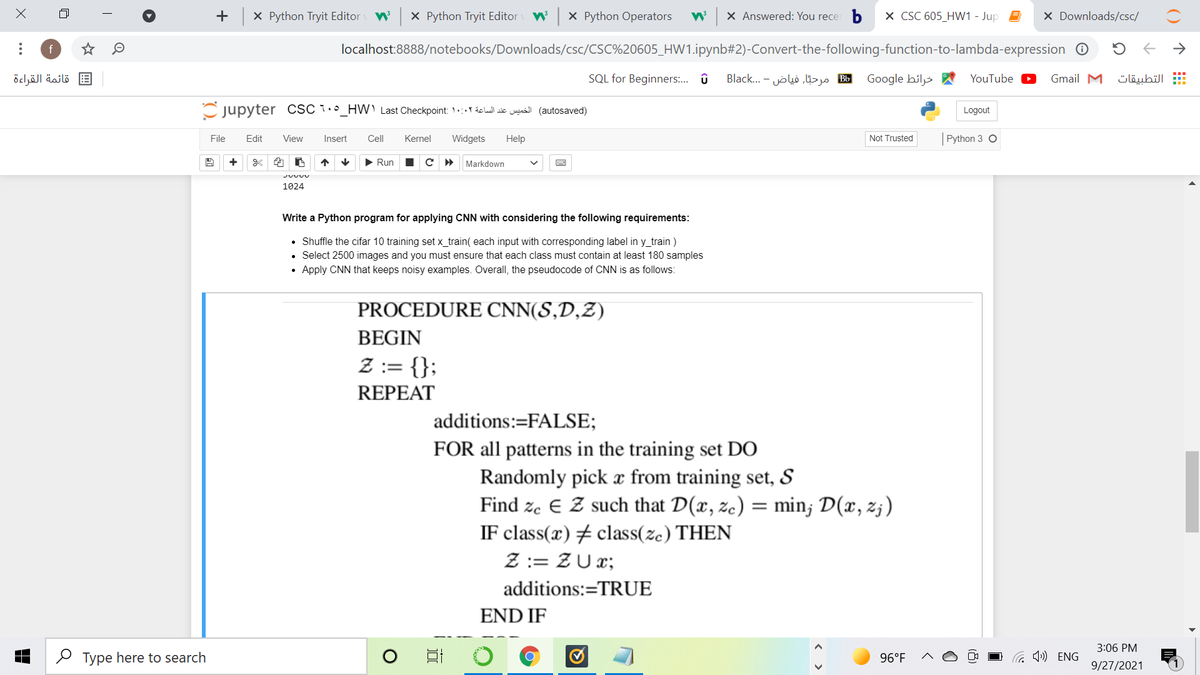
Transcribed Image Text:+ X Python Tryit Editor
W X Python Tryit Editor
W X Python Operators
X Answered: You rece b
X CSC 605_HW1 - Jup E
X Downloads/csc/
f
localhost:8888/notebooks/Downloads/csc/CSC%20605_HW1.ipynb#2)-Convert-the-following-function-to-lambda-expression O
->
قائمة القراءة
SQL for Beginners:. û
B مرحبّار فياض - . .Black
Google hiljs A
YouTube
Gmail M
التطبيقات
Cjupyter CSC 1.•_HW) Last Checkpoint:r ácldl io uual (autosaved)
Logout
Widgets
Python 3 O
File
Edit
View
Insert
Cell
Kernel
Help
Not Trusted
+
Run
Markdown
1024
Write a Python program for applying CNN with considering the following requirements:
• Shuffle the cifar 10 training set x_train( each input with corresponding label in y_train )
• Select 2500 images and you must ensure that each class must contain at least 180 samples
• Apply CNN that keeps noisy examples. Overall, the pseudocode of CNN is as follows:
PROCEDURE CNN(S,D,Z)
BEGIN
Z := {};
REPEAT
additions:=FALSE;
FOR all patterns in the training set DO
Randomly pick æ from training set, S
Find z. E Z such that D(x, zc) = min; D(x, z;)
IF class(x) # class(zc) THEN
Z := Z U x;
additions:=TRUE
END IF
3:06 PM
O Type here to search
96°F
O G ») ENG
9/27/2021
()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
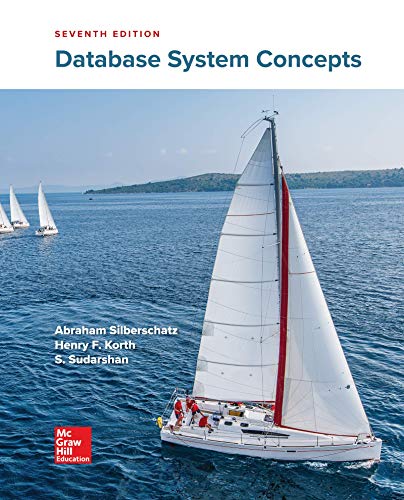
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
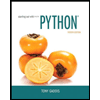
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
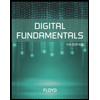
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
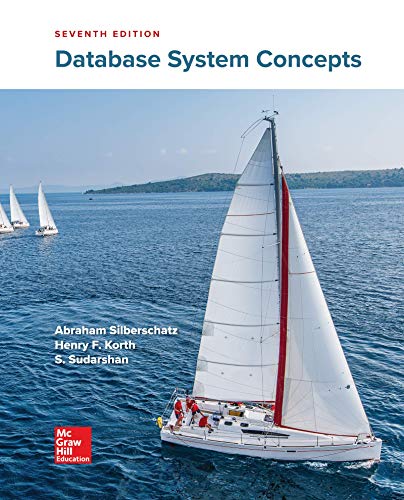
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
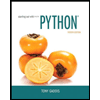
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
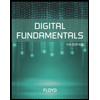
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
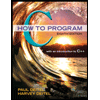
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
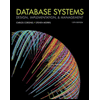
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
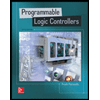
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education