Write a Python program that analyses temperature information of some cities given in an input file named "temperature.txt". "WeatherReport.txt" which contains a table summarizing the data where each city is listed with its low, high, and average temperatures, and its weather condition {Hot, Warm, Nice, Cold, Freezing, Extreme}. Each line in the input file contains the city name, followed by 5 temperature readings taken during the day. Samples of input and output files are given below. The program outputs a text file named Requirements: The program must have at least 2 functions: 1. The main function: It reads the data from the input file, stores it in a list, then displays the data in a table with the weather condition for each city. It must call the function weatherCondition to find the condition. It may also call other functions if needed. 2. The weatherCondition(low, high, average) function: It takes three parameters (low, high, and average temperatures), and returns the condition of the weather as a string according to the following table: Temperature Low (L) Weather High (H) H 2 40 Average (A) Condition L>0 Hot A> 25 L>0 H< 40 Warm 202Α2 25 L>0 H< 40 Nice L>0 H< 40 Cold A< 20 L<0 H< 40 Freezing Otherwise Extreme For example, if the average temperature is 21 and it always stays between 0 and 40 during the day, then the weather condition is Nice. (Hint: Study the table carefully and
Write a Python program that analyses temperature information of some cities given in an input file named "temperature.txt". "WeatherReport.txt" which contains a table summarizing the data where each city is listed with its low, high, and average temperatures, and its weather condition {Hot, Warm, Nice, Cold, Freezing, Extreme}. Each line in the input file contains the city name, followed by 5 temperature readings taken during the day. Samples of input and output files are given below. The program outputs a text file named Requirements: The program must have at least 2 functions: 1. The main function: It reads the data from the input file, stores it in a list, then displays the data in a table with the weather condition for each city. It must call the function weatherCondition to find the condition. It may also call other functions if needed. 2. The weatherCondition(low, high, average) function: It takes three parameters (low, high, and average temperatures), and returns the condition of the weather as a string according to the following table: Temperature Low (L) Weather High (H) H 2 40 Average (A) Condition L>0 Hot A> 25 L>0 H< 40 Warm 202Α2 25 L>0 H< 40 Nice L>0 H< 40 Cold A< 20 L<0 H< 40 Freezing Otherwise Extreme For example, if the average temperature is 21 and it always stays between 0 and 40 during the day, then the weather condition is Nice. (Hint: Study the table carefully and
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Warning. :-
Already answered but it's wrong don't copy also.
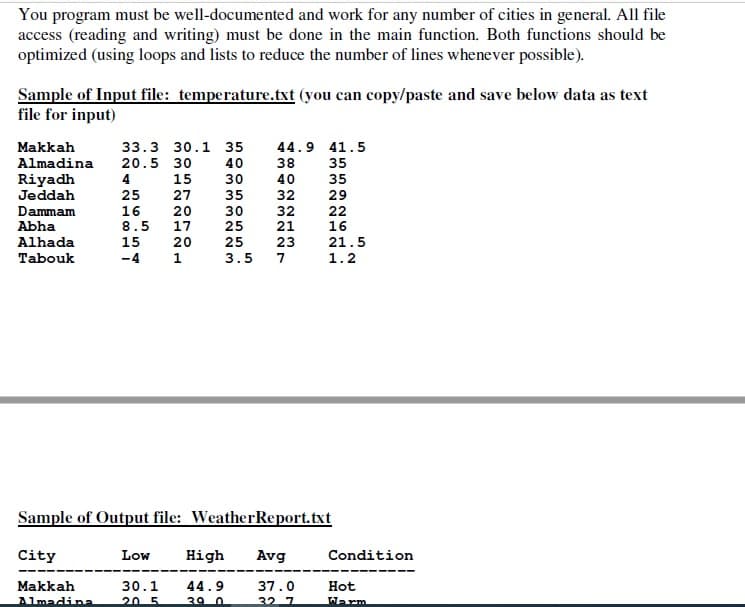
Transcribed Image Text:You program must be well-documented and work for any number of cities in general. All file
access (reading and writing) must be done in the main function. Both functions should be
optimized (using loops and lists to reduce the number of lines whenever possible).
Sample of Input file: temperature.txt (you can copy/paste and save below data as text
file for input)
Makkah
Almadina
Riyadh
Jeddah
33.3 30.1 35
44.9 41.5
20.5 30
38
35
4
15
40
35
25
27
35
32
29
Dammam
Abha
16
8.5
20
17
30
25
32
21
22
16
Alhada
15
20
25
23
21.5
Tabouk
-4
1
3.5
7
1.2
Sample of Output file: WeatherReport.txt
City
Low
High
Avg
Condition
37.0
32. 7
Makkah
30.1
44.9
39 0
Hot
Almadina
20 5
Warm
0 O N N-
50O5 O55
M 4 M m32 N3
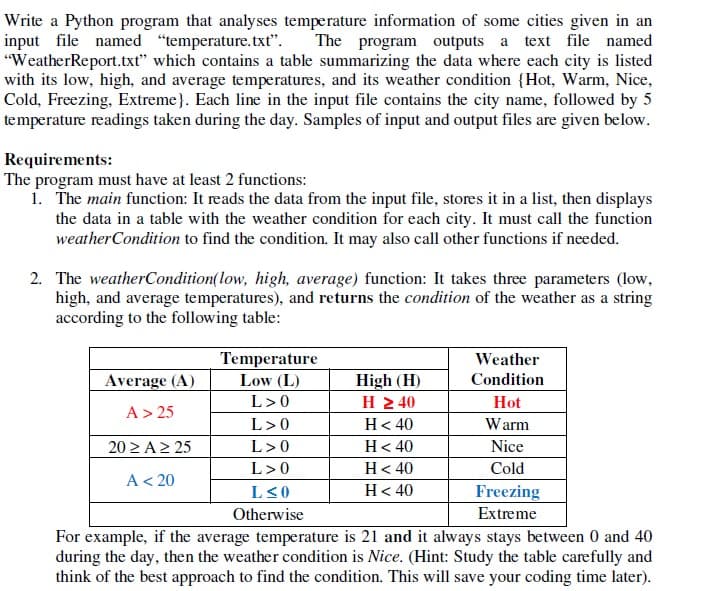
Transcribed Image Text:Write a Python program that analyses temperature information of some cities given in an
input file named "temperature.txt".
"WeatherReport.txt" which contains a table summarizing the data where each city is listed
with its low, high, and average temperatures, and its weather condition {Hot, Warm, Nice,
Cold, Freezing, Extreme}. Each line in the input file contains the city name, followed by 5
temperature readings taken during the day. Samples of input and output files are given below.
The program outputs a text file named
Requirements:
The program must have at least 2 functions:
1. The main function: It reads the data from the input file, stores it in a list, then displays
the data in a table with the weather condition for each city. It must call the function
weatherCondition to find the condition. It may also call other functions if needed.
2. The weatherCondition(low, high, average) function: It takes three parameters (low,
high, and average temperatures), and returns the condition of the weather as a string
according to the following table:
Temperature
Low (L)
Weather
High (H)
H 2 40
Average (A)
Condition
L>0
Hot
A> 25
L>0
H< 40
Warm
20 ΣΑ 25
L>0
H< 40
Nice
L>0
H< 40
Cold
A< 20
L<0
H< 40
Freezing
Otherwise
Extreme
For example, if the average temperature is 21 and it always stays between 0 and 40
during the day, then the weather condition is Nice. (Hint: Study the table carefully and
think of the best approach to find the condition. This will save your coding time later).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
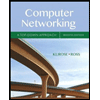
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
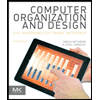
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
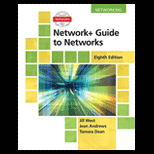
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
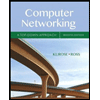
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
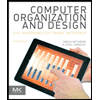
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
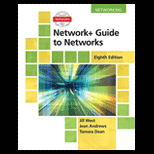
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
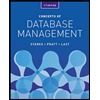
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
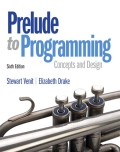
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
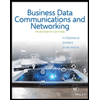
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY