Write a python program that computes the zakat obligatory charity, on camels. The program asks the user to input the number of camels, and then the zakat is calc ulated based on the following table: The number of camels ranging Zakat Amount from to 1 4 No zakat 5 24 1 sheep () for every 5 camels 25 35 1 bint Makhadh ( ): Female camel greater than 1 year old, but less than 2 years old. 36 45 1 bint Laboun ( :): Female camel greater than 2 years old, but less than 3 years old. 46 60 1 Hiqqah (*: Female camel greater than 3 years old, but less than 4 years old. 61 75 1 Jathaah (): Female camel greater than 4 years old. 76 90 2 bint Laboun 91 120 2 Hiqqah 121 No limit 1 bint Laboun for every 40 camels and 1 Hiqqah for every 50 camels according to the rule below
Write a python program that computes the zakat obligatory charity, on camels. The program asks the user to input the number of camels, and then the zakat is calc ulated based on the following table: The number of camels ranging Zakat Amount from to 1 4 No zakat 5 24 1 sheep () for every 5 camels 25 35 1 bint Makhadh ( ): Female camel greater than 1 year old, but less than 2 years old. 36 45 1 bint Laboun ( :): Female camel greater than 2 years old, but less than 3 years old. 46 60 1 Hiqqah (*: Female camel greater than 3 years old, but less than 4 years old. 61 75 1 Jathaah (): Female camel greater than 4 years old. 76 90 2 bint Laboun 91 120 2 Hiqqah 121 No limit 1 bint Laboun for every 40 camels and 1 Hiqqah for every 50 camels according to the rule below
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
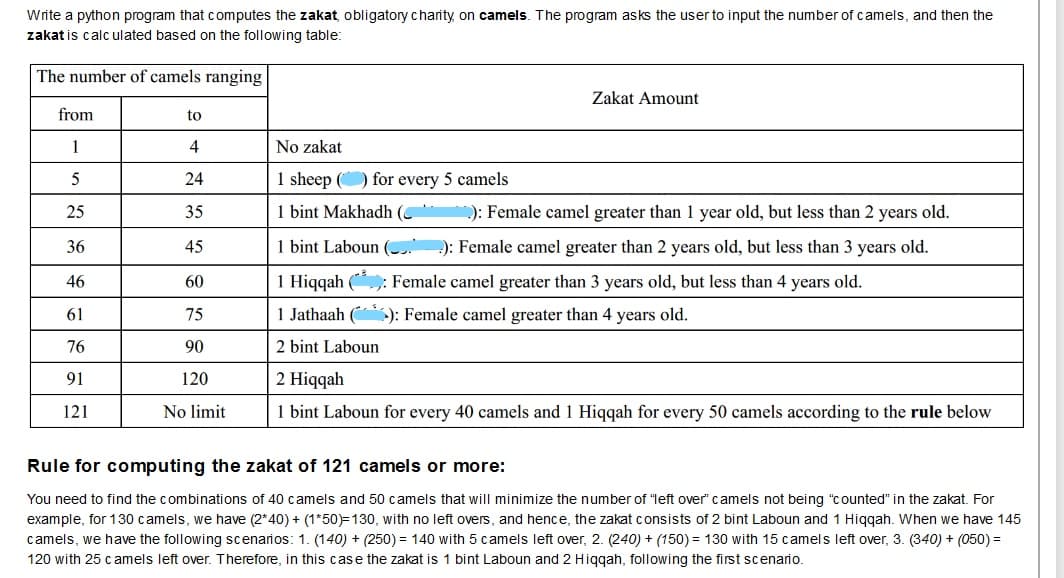
Transcribed Image Text:Write a python program that computes the zakat obligatory charity, on camels. The program asks the user to input the number of camels, and then the
zakat is calculated based on the following table:
The number of camels ranging
Zakat Amount
from
to
1
4
No zakat
24
1 sheep (O for every 5 camels
25
35
1 bint Makhadh (C
:): Female camel greater than 1 year old, but less than 2 years old.
36
45
1 bint Laboun ( ): Female camel greater than 2 years old, but less than 3 years old.
46
60
1 Hiqqah (*: Female camel greater than 3 years old, but less than 4 years old.
61
75
1 Jathaah (): Female camel greater than 4 years old.
76
90
2 bint Laboun
91
120
2 Hiqqah
121
No limit
1 bint Laboun for every 40 camels and 1 Hiqqah for every 50 camels according to the rule below
Rule for computing the zakat of 121 camels or more:
You need to find the combinations of 40 camels and 50 camels that will minimize the number of "left over" camels not being "counted" in the zakat. For
example, for 130 camels, we have (2*40) + (1*50)=130, with no left overs, and hence, the zakat consists of 2 bint Laboun and 1 Higgah. When we have 145
camels, we have the following scenarios: 1. (140) + (250) = 140 with 5 camels left over, 2. (240) + (150) = 130 with 15 camels left over, 3. (340) + (050) =
120 with 25 camels left over. Therefore, in this case the zakat is 1 bint Laboun and 2 Hiqqah, following the first scenario.
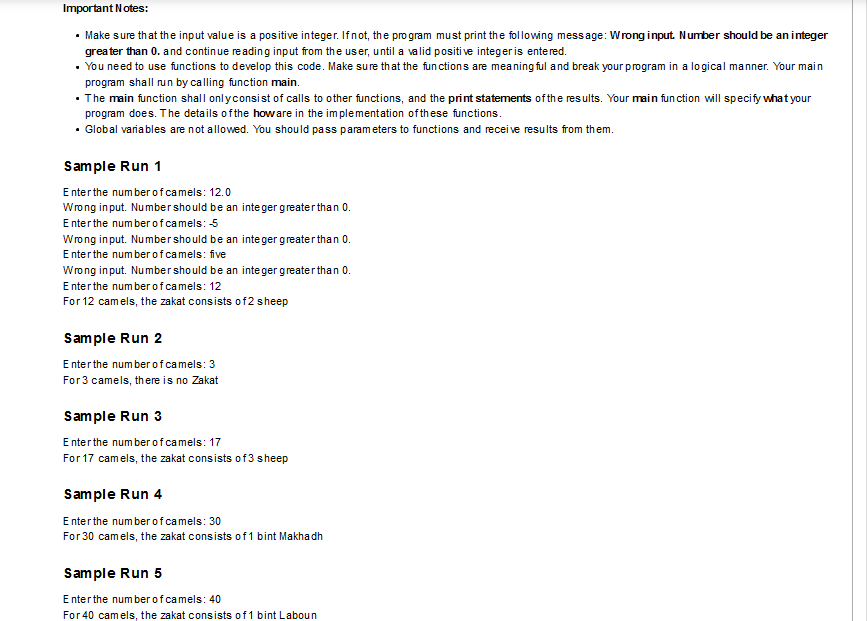
Transcribed Image Text:Important Notes:
• Make sure that the input value is a positive integer. Ifnot, the pro gram must print the following message: Wrong input. Number should be an integer
greater than 0. and continue reading input from the user, until a valid positi ve integer is entered.
• You need to use functions to develop this code. Make sure that the functions are meaning ful and break your program in a lo gical manner. Your main
program shall run by calling function main.
• The main function shall onlyconsist of calls to other functions, and the print statements of the results. Your main fun ction will specify what your
program does. The details of the howare in the im plementation ofthese functions.
• Gobal variables are not allowed. You should pass parameters to functions and receive results from them.
Sample Run 1
E nterthe number ofcamels: 12.0
Wrong in put. Numbershould be an integer greater than 0.
E nterthe number o fcamels: 5
Wrong in put. Numbershould be an inte ger greaterthan 0.
E nterthe num berof camels: five
Wrong in put. Numbershould be an integer greaterthan 0.
E nterthe number o fcamels: 12
For 12 camels, the zakat consists of2 sheep
Sample Run 2
E nterthe number ofcamels: 3
For3 camels, there is no Zakat
Sample Run 3
E nterthe number ofcamels: 17
For 17 camels, the zakat consists of3 sheep
Sample Run 4
E nterthe number ofcamels: 30
For 30 camels, the zakat consists of1 bint Makhadh
Sample Run 5
E nterthe number ofcamels: 40
For 40 camels, the zakat consists of 1 bint Laboun
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
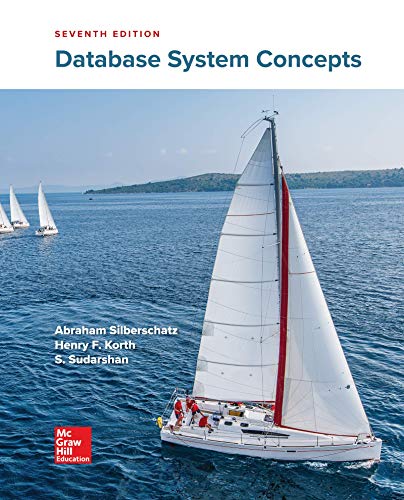
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
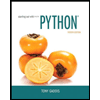
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
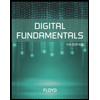
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
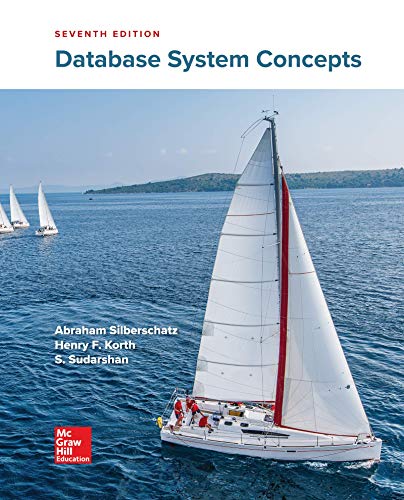
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
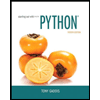
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
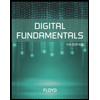
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
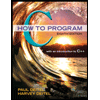
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
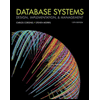
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
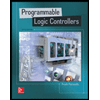
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education