Write a Python program to find the size of a singly linked list. The Program also asks the user to input a name of a programming/scripting language ( PHP, Python, C++, etc.). The Program should also check if the inputted string is already added to the list. If existing in the list, prevent adding a new node in the list. The program must output the list of inputted strings and the total size of the link list every time a new node is added or removed. Sample Output: Original list: PHP Python C# C++ Java Size of the list: 5
Write a Python program to find the size of a singly linked list. The Program also asks the user to input a name of a programming/scripting language ( PHP, Python, C++, etc.). The Program should also check if the inputted string is already added to the list. If existing in the list, prevent adding a new node in the list. The program must output the list of inputted strings and the total size of the link list every time a new node is added or removed. Sample Output: Original list: PHP Python C# C++ Java Size of the list: 5
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter13: Structures
Section13.4: Linked Lists
Problem 2E
Related questions
Question
PYTHON: LINKED LISTS
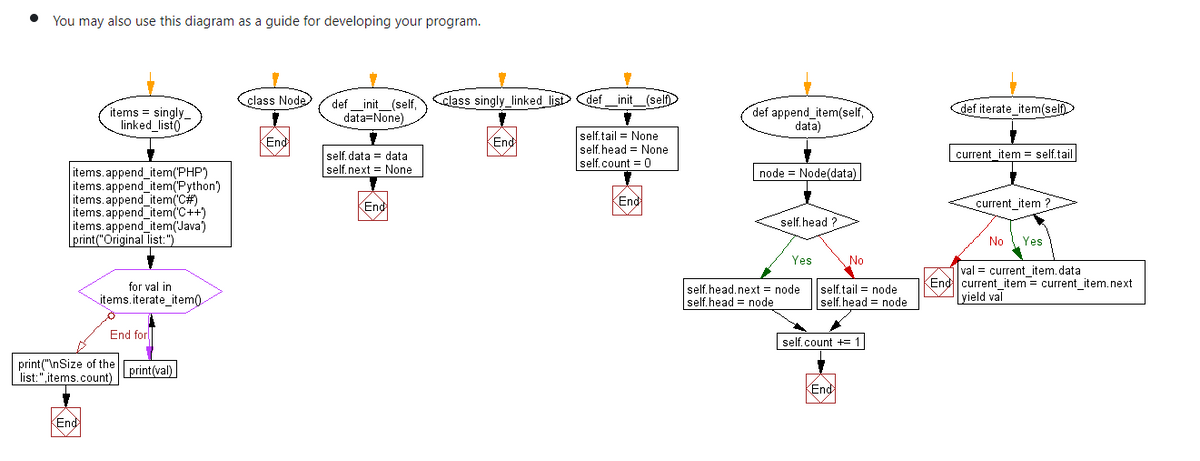
Transcribed Image Text:You may also use this diagram as a guide for developing your program.
Cclass Node
Colass singly linked list Cdef_init_(self
items = singly
linked_list)
def_init_(self,
data=None)
def append_item(self
data)
def iterate_item(self
self.tail = None
self.head = None
self.count = 0
End
KEnd
self. data = data
self.next = None
current item = self.tail
items.append_item('PHP)
items. append_item(Python)
items.append_item('C#)
items. append item(C++)
items. append_item(Java)
print("Original list:")
node = Node(data)
End
End
current_item ?
self.head ?
No 1 Yes
Yes
No
val = current item.data
End current_item = current_item.next
yield val
for val in
self.head.next = node
self. head = node
self.tail = node
self.head = node
jtems.iterate item()
End for
self.count +=
print("\nSize of the
list:",items.count)
print(val)
End
KEnd
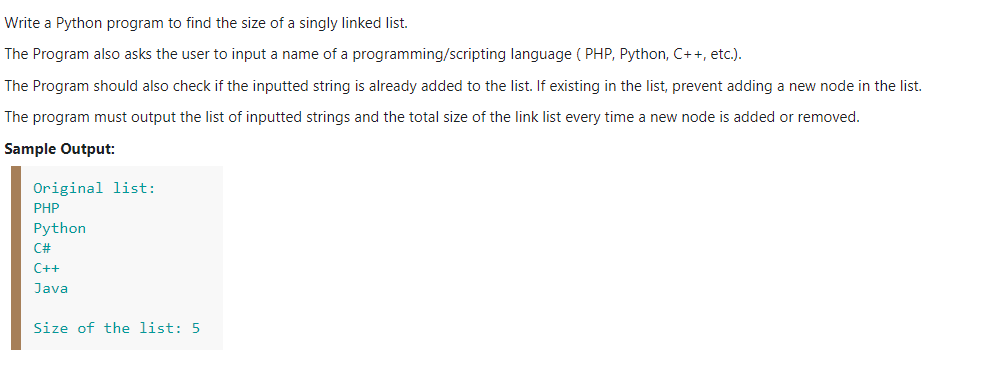
Transcribed Image Text:Write a Python program to find the size of a singly linked list.
The Program also asks the user to input a name of a programming/scripting language ( PHP, Python, C++, etc.).
The Program should also check if the inputted string is already added to the list. If existing in the list, prevent adding a new node in the list.
The program must output the list of inputted strings and the total size of the link list every time a new node is added or removed.
Sample Output:
Original list:
PHP
Python
C#
C++
Java
Size of the list: 5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
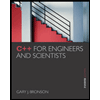
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
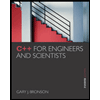
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr