Write a Python program which takes any data set (1, 1), (2, 92),... (*N, YN) and computes the best fit polynomial P(x) = a + a₁ + a₂x² +.... +akak,
Write a Python program which takes any data set (1, 1), (2, 92),... (*N, YN) and computes the best fit polynomial P(x) = a + a₁ + a₂x² +.... +akak,
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 24PE
Related questions
Question
Please use python and show codes. The second picture is another example we did
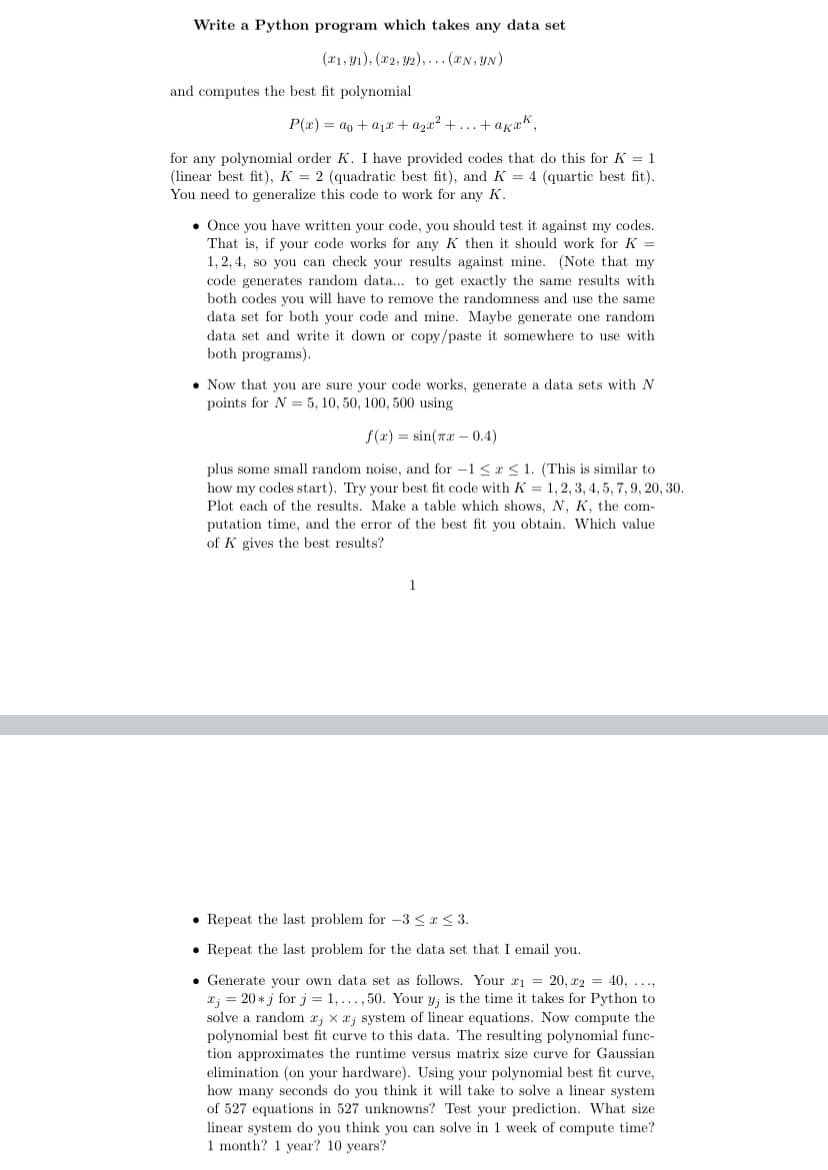
Transcribed Image Text:Write a Python program which takes any data set
(x1, y1), (2, 2),... (@N, YN)
and computes the best fit polynomial
P(x)= a + a₁ + a₂x²+...+ akak,
for any polynomial order K. I have provided codes that do this for K = 1
(linear best fit), K = 2 (quadratic best fit), and K = 4 (quartic best fit).
You need to generalize this code to work for any K.
. Once you have written your code, you should test it against my codes.
That is, if your code works for any K then it should work for K =
1,2,4, so you can check your results against mine. (Note that my
code generates random data... to get exactly the same results with
both codes you will have to remove the randomness and use the same.
data set for both your code and mine. Maybe generate one random.
data set and write it down or copy/paste it somewhere to use with
both programs).
Now that you are sure your code works, generate a data sets with N
points for N 5, 10, 50, 100, 500 using
f(x)=sin(ra-0.4)
plus some small random noise, and for -1 ≤x≤1. (This is similar to
how my codes start). Try your best fit code with K = 1, 2, 3, 4, 5, 7, 9, 20, 30.
Plot each of the results. Make a table which shows, N, K, the com-
putation time, and the error of the best fit you obtain. Which value
of K gives the best results?
1
• Repeat the last problem for -3 ≤x≤ 3.
Repeat the last problem for the data set that I email you.
. Generate your own data set as follows. Your x₁ = 20, 22 = 40, ...,
x = 20 * j for j = 1,...,50. Your y, is the time it takes for Python to
solve a random x; x xj system of linear equations. Now compute the
polynomial best fit curve to this data. The resulting polynomial func-
tion approximates the runtime versus matrix size curve for Gaussian
elimination (on your hardware). Using your polynomial best fit curve,
how many seconds do you think it will take to solve a linear system.
of 527 equations in 527 unknowns? Test your prediction. What size
linear system do you think you can solve in 1 week of compute time?
1 month? 1 year? 10 years?
![import time
import math
import matplotlib.pyplot as plt
import numpy as np
#def f(x):
#
return 1.76 *x + 2.5
def f(x):
return 2*x**4 - 0.5*x**3 + 0.5*x**2 - x + 0.25
N = 7
a = -2
b = 2
step = (b-a)/N
X = np.linspace(a, b, N)
Y = np.zeros (N)
the_random_noise = np.random.random (N)
for n in range (0,N):
X[n]X[n] +
((-1) **n) *0.1*step*the_random_noise[n]
for n in range (0,N):
Y[n] = f(X[n]) +
((-1) **n) *0.5*the_random_noise[n]
#Try quartic best fit: K = 4 (5 equations in 5
unknowns a0, al, a2, a3, a4):
# N = 7 Data points
b0= 0
O O O O O
b1 = 0
b2 =
b3
b4
0
A1 = 0
A2 = 0
A3 = 0
A4 = 0
0
= 0
=
A5 = 0
A6 = 0
A7 = 0
A8 = 0
for i in range(0, N):
b0b0 + Y[i]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34c2a7c6-20d8-45e7-8c90-b1c224068cda%2Fb8016b41-042c-429d-9be6-48da8f099262%2F9luxiu_processed.jpeg&w=3840&q=75)
Transcribed Image Text:import time
import math
import matplotlib.pyplot as plt
import numpy as np
#def f(x):
#
return 1.76 *x + 2.5
def f(x):
return 2*x**4 - 0.5*x**3 + 0.5*x**2 - x + 0.25
N = 7
a = -2
b = 2
step = (b-a)/N
X = np.linspace(a, b, N)
Y = np.zeros (N)
the_random_noise = np.random.random (N)
for n in range (0,N):
X[n]X[n] +
((-1) **n) *0.1*step*the_random_noise[n]
for n in range (0,N):
Y[n] = f(X[n]) +
((-1) **n) *0.5*the_random_noise[n]
#Try quartic best fit: K = 4 (5 equations in 5
unknowns a0, al, a2, a3, a4):
# N = 7 Data points
b0= 0
O O O O O
b1 = 0
b2 =
b3
b4
0
A1 = 0
A2 = 0
A3 = 0
A4 = 0
0
= 0
=
A5 = 0
A6 = 0
A7 = 0
A8 = 0
for i in range(0, N):
b0b0 + Y[i]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
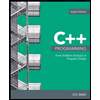
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
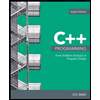
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning