Write a string class. To avoid conflicts with other similarly named classes, we will call our version MyString. This object is designed to make working with sequences of characters a little more convenient and less error-prone than handling raw c-strings, (although it will be implemented as a c-string behind the scenes). The MyString class will handle constructing strings, reading/printing, and accessing characters. In addition, the MyString object will have the ability to make a full deep-copy of itself when copied. Your class must have only one data member, a c-string implemented as a dynamic array. In particular, you must not use a data member to keep track of the size or length of the MyString. #include "mystring.h" #include #include #include using namespace std; using namespace cs_mystring; void BasicTest(); void RelationTest(); void CopyTest(); MyString AppendTest(const MyString& ref, MyString val); string boolString(bool convertMe); int main() { BasicTest(); RelationTest(); CopyTest(); } void BasicTest() { MyString s; cout << "----- Testing basic String creation & printing" << endl; const MyString strs[] = {MyString("Wow"), MyString("C++ is neat!"), MyString(""), MyString("a-z")}; for (int i = 0; i < 4; i++){ cout << "string [" << i <<"] = " << strs[i] << endl; } cout << endl << "----- Testing access to characters (using const)" << endl; const MyString s1("abcdefghijklmnopqsrtuvwxyz"); cout << "Whole string is " << s1 << endl; cout << "now char by char: "; for (int i = 0; i < s1.length(); i++){ cout << s1[i]; } cout << endl << "----- Testing access to characters (using non-const)" << endl; MyString s2("abcdefghijklmnopqsrtuvwxyz"); cout << "Start with " << s2; for (int i = 0; i < s2.length(); i++){ s2[i] = toupper(s2[i]); } cout << " and convert to " << s2 << endl; } string boolString(bool convertMe) { if (convertMe) { return "true"; } else { return "false"; } } void RelationTest() { cout << "\n----- Testing relational operators between MyStrings\n"; const MyString strs[] = {MyString("app"), MyString("apple"), MyString(""), MyString("Banana"), MyString("Banana")}; for (int i = 0; i < 4; i++) { cout << "Comparing " << strs[i] << " to " << strs[i+1] << endl; cout << " Is left < right? " << boolString(strs[i] < strs[i+1]) << endl; cout << " Is left <= right? " << boolString(strs[i] <= strs[i+1]) << endl; cout << " Is left > right? " << boolString(strs[i] > strs[i+1]) << endl; cout << " Is left >= right? " << boolString(strs[i] >= strs[i+1]) << endl; cout << " Does left == right? " << boolString(strs[i] == strs[i+1]) << endl; cout << " Does left != right ? " << boolString(strs[i] != strs[i+1]) << endl; } cout << "\n----- Testing relations between MyStrings and char *\n"; MyString s("he"); const char *t = "hello"; cout << "Comparing " << s << " to " << t << endl; cout << " Is left < right? " << boolString(s < t) << endl; cout << " Is left <= right? " << boolString(s <= t) << endl; cout << " Is left > right? " << boolString(s > t) << endl; cout << " Is left >= right? " << boolString(s >= t) << endl; cout << " Does left == right? " << boolString(s == t) << endl; cout << " Does left != right ? " << boolString(s != t) << endl; MyString u("wackity"); const char *v = "why"; cout << "Comparing " << v << " to " << u << endl; cout << " Is left < right? " << boolString(v < u) << endl; cout << " Is left <= right? " << boolString(v <= u) << endl; cout << " Is left > right? " << boolString(v > u) << endl; cout << " Is left >= right? " << boolString(v >= u) << endl; cout << " Does left == right? " << boolString(v == u) << endl; cout << " Does left != right ? " << boolString(v != u) << endl; } MyString AppendTest(const MyString& ref, MyString val) { val[0] = 'B'; return val; } void CopyTest() { cout << "\n----- Testing copy constructor and operator= on MyStrings\n"; MyString orig("cake"); MyString copy(orig); // invoke copy constructor copy[0] = 'f'; // change first letter of the *copy* cout << "original is " << orig << ", copy is " << copy << endl; MyString copy2; copy2 = orig; copy2[0] = 'f'; cout << "original is " << orig << ", copy is " << copy2 << endl; copy2 = "Copy Cat"; copy2 = copy2; cout << "after self assignment, copy is " << copy2 << endl; cout << "Testing pass & return MyStrings by value and ref" << endl; MyString val = "winky"; MyString sum = AppendTest("Boo", val); cout << "after calling Append, sum is " << sum << endl; cout << "val is " << val << endl; val = sum; cout << "after assign, val is " << val << endl; }
Write a string class. To avoid conflicts with other similarly named classes, we will call our version MyString. This object is designed to make working with sequences of characters a little more convenient and less error-prone than handling raw c-strings, (although it will be implemented as a c-string behind the scenes). The MyString class will handle constructing strings, reading/printing, and accessing characters. In addition, the MyString object will have the ability to make a full deep-copy of itself when copied.
Your class must have only one data member, a c-string implemented as a dynamic array. In particular, you must not use a data member to keep track of the size or length of the MyString.
#include "mystring.h"
#include <cctype>
#include <iostream>
#include <string>
using namespace std;
using namespace cs_mystring;
void BasicTest();
void RelationTest();
void CopyTest();
MyString AppendTest(const MyString& ref, MyString val);
string boolString(bool convertMe);
int main()
{
BasicTest();
RelationTest();
CopyTest();
}
void BasicTest()
{
MyString s;
cout << "----- Testing basic String creation & printing" << endl;
const MyString strs[] =
{MyString("Wow"), MyString("C++ is neat!"),
MyString(""), MyString("a-z")};
for (int i = 0; i < 4; i++){
cout << "string [" << i <<"] = " << strs[i] << endl;
}
cout << endl << "----- Testing access to characters (using const)" << endl;
const MyString s1("abcdefghijklmnopqsrtuvwxyz");
cout << "Whole string is " << s1 << endl;
cout << "now char by char: ";
for (int i = 0; i < s1.length(); i++){
cout << s1[i];
}
cout << endl << "----- Testing access to characters (using non-const)" << endl;
MyString s2("abcdefghijklmnopqsrtuvwxyz");
cout << "Start with " << s2;
for (int i = 0; i < s2.length(); i++){
s2[i] = toupper(s2[i]);
}
cout << " and convert to " << s2 << endl;
}
string boolString(bool convertMe) {
if (convertMe) {
return "true";
} else {
return "false";
}
}
void RelationTest()
{
cout << "\n----- Testing relational operators between MyStrings\n";
const MyString strs[] =
{MyString("app"), MyString("apple"), MyString(""),
MyString("Banana"), MyString("Banana")};
for (int i = 0; i < 4; i++) {
cout << "Comparing " << strs[i] << " to " << strs[i+1] << endl;
cout << " Is left < right? " << boolString(strs[i] < strs[i+1]) << endl;
cout << " Is left <= right? " << boolString(strs[i] <= strs[i+1]) << endl;
cout << " Is left > right? " << boolString(strs[i] > strs[i+1]) << endl;
cout << " Is left >= right? " << boolString(strs[i] >= strs[i+1]) << endl;
cout << " Does left == right? " << boolString(strs[i] == strs[i+1]) << endl;
cout << " Does left != right ? " << boolString(strs[i] != strs[i+1]) << endl;
}
cout << "\n----- Testing relations between MyStrings and char *\n";
MyString s("he");
const char *t = "hello";
cout << "Comparing " << s << " to " << t << endl;
cout << " Is left < right? " << boolString(s < t) << endl;
cout << " Is left <= right? " << boolString(s <= t) << endl;
cout << " Is left > right? " << boolString(s > t) << endl;
cout << " Is left >= right? " << boolString(s >= t) << endl;
cout << " Does left == right? " << boolString(s == t) << endl;
cout << " Does left != right ? " << boolString(s != t) << endl;
MyString u("wackity");
const char *v = "why";
cout << "Comparing " << v << " to " << u << endl;
cout << " Is left < right? " << boolString(v < u) << endl;
cout << " Is left <= right? " << boolString(v <= u) << endl;
cout << " Is left > right? " << boolString(v > u) << endl;
cout << " Is left >= right? " << boolString(v >= u) << endl;
cout << " Does left == right? " << boolString(v == u) << endl;
cout << " Does left != right ? " << boolString(v != u) << endl;
}
MyString AppendTest(const MyString& ref, MyString val)
{
val[0] = 'B';
return val;
}
void CopyTest()
{
cout << "\n----- Testing copy constructor and operator= on MyStrings\n";
MyString orig("cake");
MyString copy(orig); // invoke copy constructor
copy[0] = 'f'; // change first letter of the *copy*
cout << "original is " << orig << ", copy is " << copy << endl;
MyString copy2;
copy2 = orig;
copy2[0] = 'f';
cout << "original is " << orig << ", copy is " << copy2 << endl;
copy2 = "Copy Cat";
copy2 = copy2;
cout << "after self assignment, copy is " << copy2 << endl;
cout << "Testing pass & return MyStrings by value and ref" << endl;
MyString val = "winky";
MyString sum = AppendTest("Boo", val);
cout << "after calling Append, sum is " << sum << endl;
cout << "val is " << val << endl;
val = sum;
cout << "after assign, val is " << val << endl;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 9 images

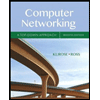
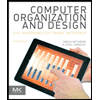
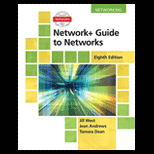
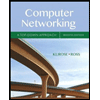
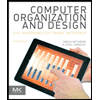
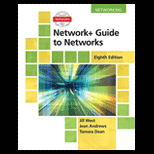
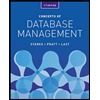
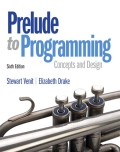
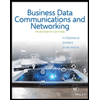