Write an assembly language program that requests input from the keyboard for two positive integers greater than 0 then it calculates and displays the greatest common divisor (GCD). The greatest common divisor (GCD) of two integers (numbers), is the largest number that divides them both without a reminder. For example, 21 is the GCD of 252 and 105 (because 252 = 21 × 12 and 105 = 21 × 5). Since 21 is the greatest common number that divides both integers it is the GCD. Your program must define and use the following procedures: • Procedure Main: This is the main procedure of the program which makes the correct sequence of calls to other procedures and displays the program title as shown in the sample run. Procedure Read_Numbers: This procedure prompts for input and reads the value of two positive integers. This procedure makes use of the procedure Check_Value (defined below) which checks if a value is out of range and displays the error message. If the returned value in BL is 0 (error) then it must prompt for the input again. Procedure Check_Value: This procedure takes as input parameters an integer value in EAX, a minimum value in EBX. If the value of EAX is greater than the value of EBX, this procedure returns in BL the value 1. Otherwise, it displays the error message "Error, incorrect input. Try again!" and returns in BL the value 0. • Procedure GCD: This procedure takes as input parameters in EAX and EBX representing the two input integers and finds the greatest common divisor (GCD) using the following algorithm (subtraction method). In addition, this procedure provides a trace of execution as shown in the sample run. function gcd (a, b) while a + b if a > b a := a - b else b := b - a return a
Write an assembly language program that requests input from the keyboard for two positive integers greater than 0 then it calculates and displays the greatest common divisor (GCD). The greatest common divisor (GCD) of two integers (numbers), is the largest number that divides them both without a reminder. For example, 21 is the GCD of 252 and 105 (because 252 = 21 × 12 and 105 = 21 × 5). Since 21 is the greatest common number that divides both integers it is the GCD. Your program must define and use the following procedures: • Procedure Main: This is the main procedure of the program which makes the correct sequence of calls to other procedures and displays the program title as shown in the sample run. Procedure Read_Numbers: This procedure prompts for input and reads the value of two positive integers. This procedure makes use of the procedure Check_Value (defined below) which checks if a value is out of range and displays the error message. If the returned value in BL is 0 (error) then it must prompt for the input again. Procedure Check_Value: This procedure takes as input parameters an integer value in EAX, a minimum value in EBX. If the value of EAX is greater than the value of EBX, this procedure returns in BL the value 1. Otherwise, it displays the error message "Error, incorrect input. Try again!" and returns in BL the value 0. • Procedure GCD: This procedure takes as input parameters in EAX and EBX representing the two input integers and finds the greatest common divisor (GCD) using the following algorithm (subtraction method). In addition, this procedure provides a trace of execution as shown in the sample run. function gcd (a, b) while a + b if a > b a := a - b else b := b - a return a
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Plz, use Irvine 32 library to solve the task.
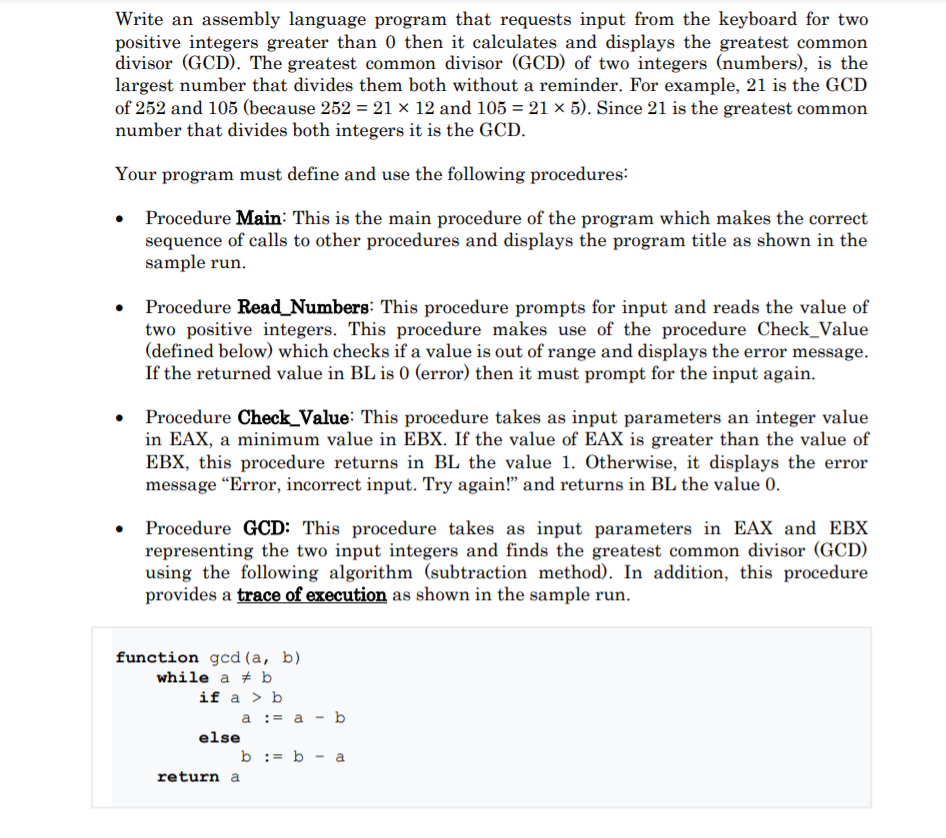
Transcribed Image Text:Write an assembly language program that requests input from the keyboard for two
positive integers greater than 0 then it calculates and displays the greatest common
divisor (GCD). The greatest common divisor (GCD) of two integers (numbers), is the
largest number that divides them both without a reminder. For example, 21 is the GCD
of 252 and 105 (because 252 = 21 × 12 and 105 = 21 × 5). Since 21 is the greatest common
number that divides both integers it is the GCD.
Your program must define and use the following procedures:
Procedure Main: This is the main procedure of the program which makes the correct
sequence of calls to other procedures and displays the program title as shown in the
sample run.
Procedure Read_Numbers: This procedure prompts for input and reads the value of
two positive integers. This procedure makes use of the procedure Check_Value
(defined below) which checks if a value is out of range and displays the error message.
If the returned value in BL is 0 (error) then it must prompt for the input again.
• Procedure Check_Value: This procedure takes as input parameters an integer value
in EAX, a minimum value in EBX. If the value of EAX is greater than the value of
EBX, this procedure returns in BL the value 1. Otherwise, it displays the error
message "Error, incorrect input. Try again!" and returns in BL the value 0.
• Procedure GCD: This procedure takes as input parameters in EAX and EBX
representing the two input integers and finds the greatest common divisor (GCD)
using the following algorithm (subtraction method). In addition, this procedure
provides a trace of execution as shown in the sample run.
function gcd (a, b)
while a + b
if a > b
a := a - b
else
b := b - a
return a
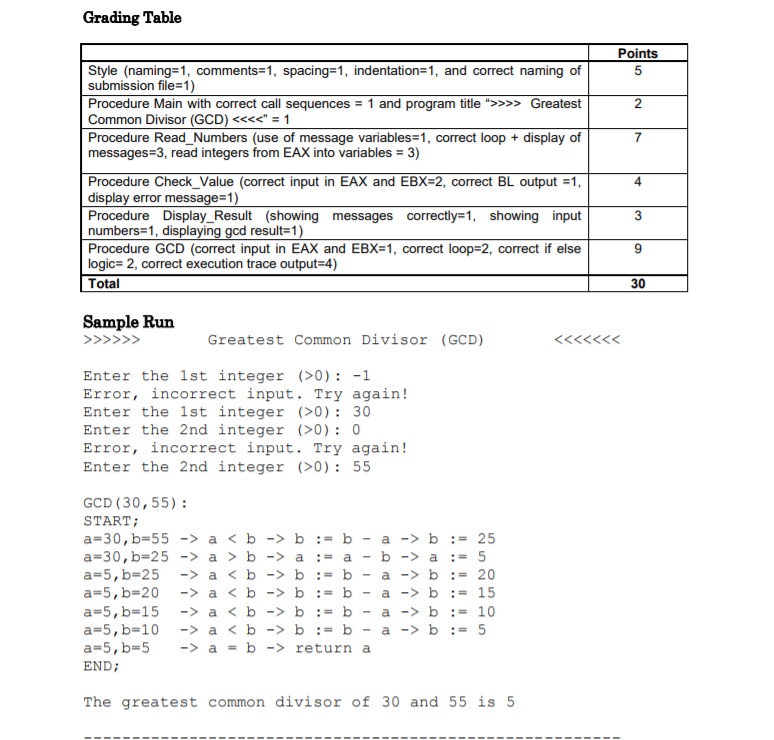
Transcribed Image Text:Grading Table
Points
Style (naming=1, comments=1, spacing=1, indentation=1, and correct naming of
submission file=1)
Procedure Main with correct call sequences = 1 and program title ">>>> Greatest
Common Divisor (GCD) <<<<" = 1
Procedure Read_Numbers (use of message variables=1, correct loop + display of
messages=3, read integers from EAX into variables = 3)
Procedure Check_Value (correct input in EAX and EBX=2, correct BL output =1,
display error message=1)
Procedure Display_Result (showing messages correctly=1, showing input
numbers=1, displaying gcd result=1)
Procedure GCD (correct input in EAX and EBX=1, correct loop=2, correct if else
logic= 2, correct execution trace output=4)
Total
4.
9
30
Sample Run
Greatest Common Divisor (GCD)
>>>>>>>
Enter the 1st integer (>0): -1
Error, incorrect input. Try again!
Enter the 1st integer (>0): 30
Enter the 2nd integer (>0): 0
Error, incorrect input. Try again!
Enter the 2nd integer (>0): 55
GCD (30,55):
START;
a=30, b=55 -> a < b -> b := b - a -> b := 25
a=30, b=25 -> a > b -> a := a
a=5,b=25
a=5,b=20
a=5,b=15
a=5,b=10
a=5,b=5
b -> a := 5
a -> b := 20
a -> b := 15
-> a <b -> b := b
-> a < b -> b := b
-> a < b -> b := b
-> a < b -> b := b - a -> b := 5
-> a = b -> return a
a -> b := 10
END;
The greatest common divisor of 30 and 55 is 5
3,
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
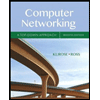
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
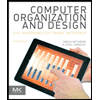
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
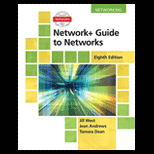
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
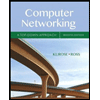
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
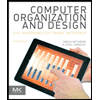
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
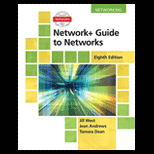
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
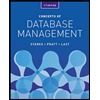
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
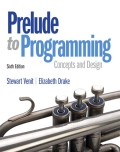
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
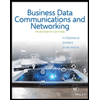
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY