Write an outline and logic for the following code: EMPTY = '-' BLACK = ‘X’ WHITE = ‘O’ BOARD_SIZE = 8 DIRECTIONS = [(0, 1), (0, -1), (1, 0), (-1, 0), (1, 1), (-1, -1), (1, -1), (-1, 1)] def initialize_board(): board = [[EMPTY for _ in range(BOARD_SIZE)] for _ in range(BOARD_SIZE)] board[3][3] = WHITE board[3][4] = BLACK board[4][3] = BLACK board[4][4] = WHITE return board def print_board(board): print(" 1 2 3 4 5 6 7 8") for i in range(BOARD_SIZE): print(f"{i + 1} ", end="") for j in range(BOARD_SIZE): print(f" {board[i][j]}", end="") print() def is_valid_move(board, row, col, player): if board[row][col] != EMPTY: return False for direction in DIRECTIONS: dr, dc = direction r, c = row + dr, col + dc while 0 <= r < BOARD_SIZE and 0 <= c < BOARD_SIZE: if board[r][c] == EMPTY: break if board[r][c] == player: return True r += dr c += dc return False def make_move(board, row, col, player): if not is_valid_move(board, row, col, player): return False board[row][col] = player for direction in DIRECTIONS: dr, dc = direction r, c = row + dr, col + dc to_flip = [] while 0 <= r < BOARD_SIZE and 0 <= c < BOARD_SIZE: if board[r][c] == EMPTY: break if board[r][c] == player: for flip_row, flip_col in to_flip: board[flip_row][flip_col] = player break to_flip.append((r, c)) r += dr c += dc return True def is_game_over(board): for i in range(BOARD_SIZE): for j in range(BOARD_SIZE): if board[i][j] == EMPTY: return False return True def count_pieces(board): black_count = 0 white_count = 0 for i in range(BOARD_SIZE): for j in range(BOARD_SIZE): if board[i][j] == BLACK: black_count += 1 elif board[i][j] == WHITE: white_count += 1 return black_count, white_count def determine_winner(black_count, white_count): if black_count > white_count: return BLACK elif white_count > black_count: return WHITE else: return EMPTY def play_game(): board = initialize_board() player = BLACK while True: print_board(board) print("Player", player, "'s turn.") print("Enter your move (row col): ") move = input().split() if len(move) != 2: print("Invalid move. Please enter row and col separated by space.") continue row, col = int(move[0]) - 1, int(move[1]) - 1 if not is_valid_move(board, row, col, player): print("Invalid move. Please choose a valid move.") continue make_move(board, row, col, player) if is_game_over(board): break player = WHITE if player == BLACK else BLACK black_count, white_count = count_pieces(board) winner = determine_winner(black_count, white_count) print_board(board) print("Game Over!") print("Black: ", black_count) print("White: ", white_count) if winner == BLACK: print("Black wins!") elif winner == WHITE: print("White wins!") else: print("It's a draw!") if __name__ == "__main__": play_game()
Write an outline and logic for the following code:
EMPTY = '-'
BLACK = ‘X’
WHITE = ‘O’
BOARD_SIZE = 8
DIRECTIONS = [(0, 1), (0, -1), (1, 0), (-1, 0), (1, 1), (-1, -1), (1, -1), (-1, 1)]
def initialize_board():
board = [[EMPTY for _ in range(BOARD_SIZE)] for _ in range(BOARD_SIZE)]
board[3][3] = WHITE
board[3][4] = BLACK
board[4][3] = BLACK
board[4][4] = WHITE
return board
def print_board(board):
print(" 1 2 3 4 5 6 7 8")
for i in range(BOARD_SIZE):
print(f"{i + 1} ", end="")
for j in range(BOARD_SIZE):
print(f" {board[i][j]}", end="")
print()
def is_valid_move(board, row, col, player):
if board[row][col] != EMPTY:
return False
for direction in DIRECTIONS:
dr, dc = direction
r, c = row + dr, col + dc
while 0 <= r < BOARD_SIZE and 0 <= c < BOARD_SIZE:
if board[r][c] == EMPTY:
break
if board[r][c] == player:
return True
r += dr
c += dc
return False
def make_move(board, row, col, player):
if not is_valid_move(board, row, col, player):
return False
board[row][col] = player
for direction in DIRECTIONS:
dr, dc = direction
r, c = row + dr, col + dc
to_flip = []
while 0 <= r < BOARD_SIZE and 0 <= c < BOARD_SIZE:
if board[r][c] == EMPTY:
break
if board[r][c] == player:
for flip_row, flip_col in to_flip:
board[flip_row][flip_col] = player
break
to_flip.append((r, c))
r += dr
c += dc
return True
def is_game_over(board):
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == EMPTY:
return False
return True
def count_pieces(board):
black_count = 0
white_count = 0
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == BLACK:
black_count += 1
elif board[i][j] == WHITE:
white_count += 1
return black_count, white_count
def determine_winner(black_count, white_count):
if black_count > white_count:
return BLACK
elif white_count > black_count:
return WHITE
else:
return EMPTY
def play_game():
board = initialize_board()
player = BLACK
while True:
print_board(board)
print("Player", player, "'s turn.")
print("Enter your move (row col): ")
move = input().split()
if len(move) != 2:
print("Invalid move. Please enter row and col separated by space.")
continue
row, col = int(move[0]) - 1, int(move[1]) - 1
if not is_valid_move(board, row, col, player):
print("Invalid move. Please choose a valid move.")
continue
make_move(board, row, col, player)
if is_game_over(board):
break
player = WHITE if player == BLACK else BLACK
black_count, white_count = count_pieces(board)
winner = determine_winner(black_count, white_count)
print_board(board)
print("Game Over!")
print("Black: ", black_count)
print("White: ", white_count)
if winner == BLACK:
print("Black wins!")
elif winner == WHITE:
print("White wins!")
else:
print("It's a draw!")
if __name__ == "__main__":
play_game()

Step by step
Solved in 3 steps

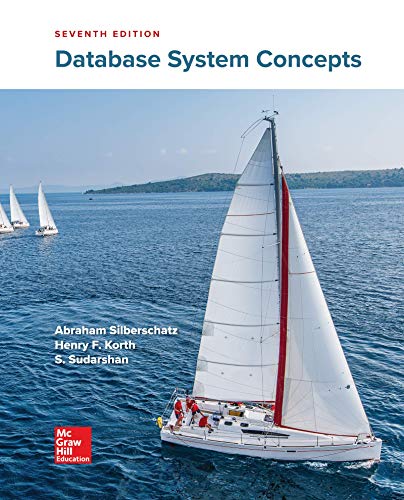
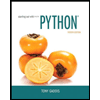
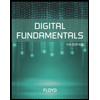
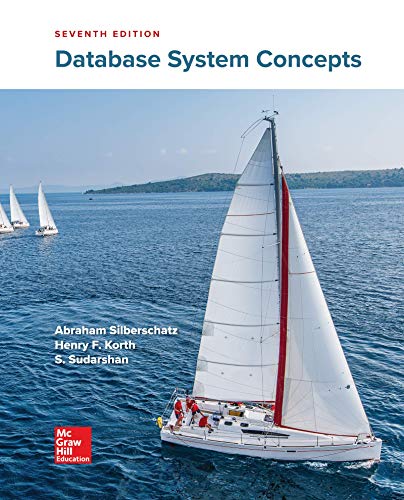
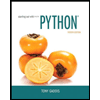
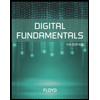
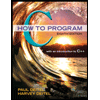
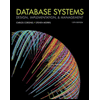
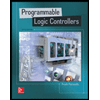