Write code in C# You must create parts of a small application that allows the user to manage the information on items for sale at a local store. The system must allow a user to add, delete and search for items. The system can hold a maximum of 10000 items. The class definition for an Item is given in the UML diagram below Note: getInfo() returns a string containing ALL of the state information neatly organized. Part 1: Create a class based on the specification above. Reminder: – means private and + means public. Part 2: a) Write a function/method called “addItem” that takes: An array of items The number of items in the array An integer representing a barcode A string representing the name of an item A string representing the description of an item A double value representing the price The function must create and add the item to the array if there is space. The function must return “true” if the addition was successful and “false” otherwise. b) Write a function/method called “listItems” that takes: An array of items The number of items in the array The function must return a string containing the information on each item in the array. c) Write a function called “pricelookup” that takes : An array of items The number of items in the array An integer representing a barcode The function must return the price of that item if it exits and -1 if no item with that barcode exits. d) Write a function called “deleteItem” that takes: An array of items The number of items in the array An integer representing a barcode The function must remove/delete the item from the array if an item with that barcode exists. It must return a Boolean value where “true” means that the item was found and deleted. It must return “false” when the item was not found. Part 3 Write a main program that (in order): Creates an array large enough to hold all of the items. Repeatedly asks the user for a barcode, name , description and price (based on the UML for Item) and adds item objects to the arrays created. This is done until a barcode of -1 is entered by the user.( Note if -1 is entered a name, description and price should not be requested) A list of all items should be displayed by using the functions/ methods created in part 2. The user should then be asked to enter a barCode to search for. The price of the item corresponding to the barCode entered should be displayed or “Item not found“ should be displayed. You must use the functions/ methods created in part 2 The user should then be asked to enter a barCode. The item corresponding to the barCode entered should be removed from the array if it exists. You must use the functions/ methods created in part 2. A list of all items should be displayed by using the functions/ methods created in part 2.
Write code in C#
You must create parts of a small application that allows the user to manage the information on items for sale at a local store.
The system must allow a user to add, delete and search for items. The system can hold a maximum of 10000 items.
The class definition for an Item is given in the UML diagram below
Note: getInfo() returns a string containing ALL of the state information neatly organized.
Part 1:
Create a class based on the specification above.
Reminder: – means private and + means public.
Part 2:
a)
Write a function/method called “addItem” that takes:
- An array of items
- The number of items in the array
- An integer representing a barcode
- A string representing the name of an item
- A string representing the description of an item
- A double value representing the price
The function must create and add the item to the array if there is space. The function must return “true” if the addition was successful and “false” otherwise.
b)
Write a function/method called “listItems” that takes:
- An array of items
- The number of items in the array
The function must return a string containing the information on each item in the array.
c)
Write a function called “pricelookup” that takes :
- An array of items
- The number of items in the array
- An integer representing a barcode
The function must return the price of that item if it exits and -1 if no item with that barcode exits.
- d) Write a function called “deleteItem” that takes:
- An array of items
- The number of items in the array
- An integer representing a barcode
The function must remove/delete the item from the array if an item with that barcode exists. It must return a Boolean value where “true” means that the item was found and deleted. It must return “false” when the item was not found.
Part 3
Write a main program that (in order):
- Creates an array large enough to hold all of the items.
- Repeatedly asks the user for a barcode, name , description and price (based on the UML for Item) and adds item objects to the arrays created. This is done until a barcode of -1 is entered by the user.( Note if -1 is entered a name, description and price should not be requested)
- A list of all items should be displayed by using the functions/ methods created in part 2.
- The user should then be asked to enter a barCode to search for. The price of the item corresponding to the barCode entered should be displayed or “Item not found“ should be displayed. You must use the functions/ methods created in part 2
- The user should then be asked to enter a barCode. The item corresponding to the barCode entered should be removed from the array if it exists. You must use the functions/ methods created in part 2.
- A list of all items should be displayed by using the functions/ methods created in part 2.
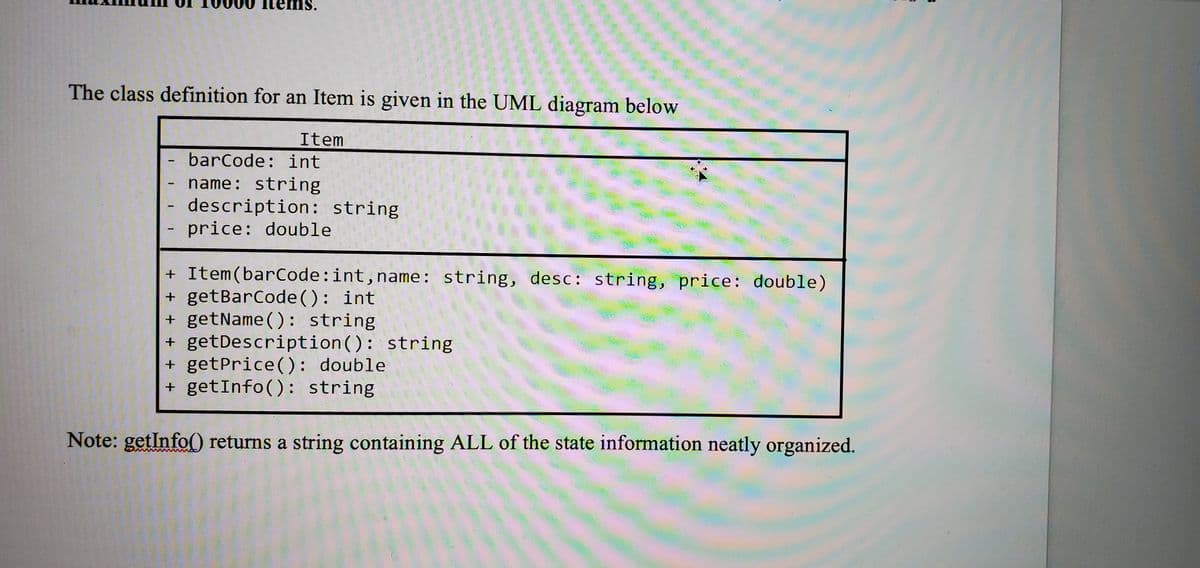

Step by step
Solved in 2 steps

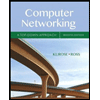
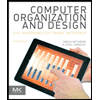
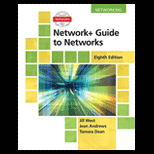
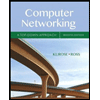
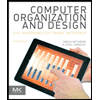
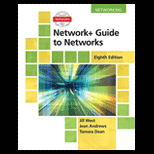
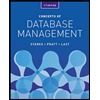
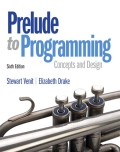
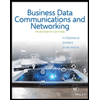