Write in Java. Given an array of integers A, find the Majority Element. Majority Element in an array of size N in an element that appears more than N/2 times. Write a function: int findMajority(int[] A) that accepts an array A. The function should return the Majority Element in the array. If no majority element then return 0. Use following methods to solve the problem: int findCandidate(int a[]) that accepts the array and find a candidate for the majority boolean isMajority(int a[], int cand) that accept the array and the candidate element and check if the candidate occurs more than n/2 times Input: 5 1 2 1 2 2 Where, First line represents the size of an array. Second line represents array elements separated by single space. Output: 2 Here for the given array, 2 appears 3 times in the array of size 5. No space after the element in the output. Assume that, N is an integer within the range [1 to 10000]. Array elements are within the range [-2147483648 to 2147483647]. - Starter Code: import java.util.*; import java.lang.*; import java.io.*; class HW2_P4 { public int findMajority(int[] array) { } int findCandidate(int a[]) { } boolean isMajority(int a[], int cand) { } } class DriverMain { public static void main(String args[]) { HW2_P4 hw = new HW2_P4(); Scanner s = new Scanner(System.in); int N = s.nextInt(); int A[] = new int[N]; for (int i = 0; i < N; i++) { A[i] = s.nextInt(); } System.out.print(hw.findMajority(A)); } }
Write in Java.
Given an array of integers A, find the Majority Element.
Majority Element in an array of size N in an element that appears more than N/2 times.
Write a function:
int findMajority(int[] A)
that accepts an array A. The function should return the Majority Element in the array. If no majority element then return 0.
Use following methods to solve the problem:
- int findCandidate(int a[])
- that accepts the array and find a candidate for the majority
- boolean isMajority(int a[], int cand)
- that accept the array and the candidate element and check if the candidate occurs more than n/2 times
Input:
5
1 2 1 2 2
Where,
- First line represents the size of an array.
- Second line represents array elements separated by single space.
Output:
2
-
Here for the given array, 2 appears 3 times in the array of size 5.
-
No space after the element in the output.
Assume that,
- N is an integer within the range [1 to 10000].
- Array elements are within the range [-2147483648 to 2147483647].
- Starter Code:
import java.util.*;
import java.lang.*;
import java.io.*;
class HW2_P4 {
public int findMajority(int[] array) {
}
int findCandidate(int a[]) {
}
boolean isMajority(int a[], int cand) {
}
}
class DriverMain {
public static void main(String args[]) {
HW2_P4 hw = new HW2_P4();
Scanner s = new Scanner(System.in);
int N = s.nextInt();
int A[] = new int[N];
for (int i = 0; i < N; i++) {
A[i] = s.nextInt();
}
System.out.print(hw.findMajority(A));
}
}

Code:
import java.util.Scanner;
class DriverMain {
public static void main(String args[]) {//main method
HW3_P4 hw3P4 = new HW3_P4();
Scanner s = new Scanner(System.in);//scanner class for user input
System.out.println("Enter the size of the array:");
int N = s.nextInt();//size of the array
int A[] = new int[N];
System.out.println("Enter array elements:");
for (int i = 0; i < N; i++) {//loop for taking array
A[i] = s.nextInt();//taking user input
}
System.out.println("Majority element in the array is:");
System.out.print(hw3P4.findMajority(A));//calling the function and printing
}
}
class HW3_P4 {//class for checking majority
public int findMajority(int[] array) {//defining the function
int n = findCandidate(array);
return n;
}
/* Function to find the candidate for Majority */
int findCandidate(int a[]) {
boolean bool;
for (int i = 0; i < a.length; i++) {
bool = isMajority(a, a[i]);
if (bool)
return a[i];
}
return 0;
}
boolean isMajority(int a[], int cand) {//Function to check if the candidate occurs more than n/2 times
int count = 0;
for (int i = 0; i < a.length; i++) {
if (cand == a[i]) {
count++;
}
}
if (count > a.length / 2)
return true;
else
return false;
}
}
Code Screenshot:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

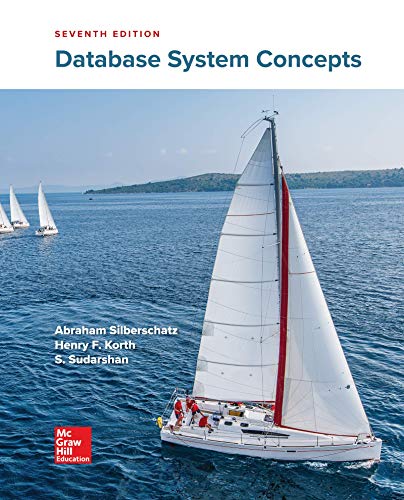
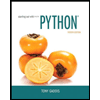
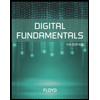
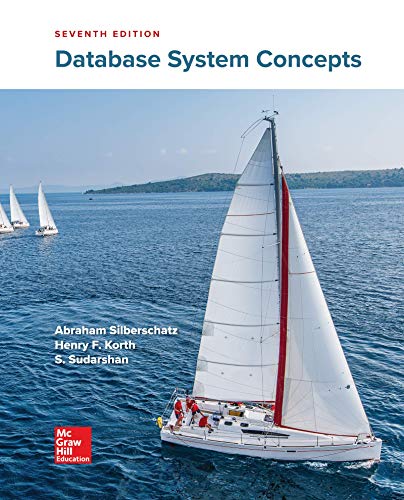
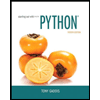
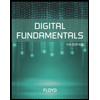
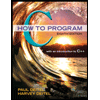
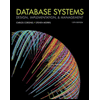
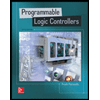