Write two local classes named Pipe and Pool as described below. Then, write a main function that reads the flowSpeed and area of a Pipe objed (p) both as double values, main will also read the width, the height, and the length of a Pool object (gardenPool) all as double values. Finally, the inputPipe of gardenPool will be the p Pipe object. Finally, the program should calculate the time it takes the gardenPool to be completely filled will water and prints it to the screen as a double value. Pipe Class • A private double field named "flowSpeed" representing the flow speed of the pipe. • A private double field named "area" representing the cross-section area (ie, kesit alan) of the pipe. * A constructor that gets two double values and initializes the object. An emply constructor. • A public function named calculateFlowRate that does not get any parameter, multiplies the flowSpeed and area of the pipe, and returns it as a double value. Pool Class • A private double field named "width" representing the width of the pool. A private double field named "height" representing the height of the pool. A private double field named "length" representing the length of the pool. A private Pipe field named "inputPipe" representing the pipe that provides water to this pool. A constructor that gets three double values, a Pipe value, and initializes the object. • A public function named calculateVolume that does not get any parameter, calculates the volume of the pool using the rectangular prism volume formula given below and returns it as a double value: Volume-height"width* length • A public function named calculateTimeToFill that does not get any parameter, calculates the time it takes for the pool to be filled with water from the pipe by dividing the volume of the pool by the flow rate of the pipe and returns it as a double value. The main function will, 1. Instantiate one Pipe object called p and instantiate a Pool object called gardenPool as explained above. 2. Use the calculateTimeToFill method of the Pool class to print out the time it takes to fill the pool and print it out. NOTE: flowSpeed and area will always be given as positive values. NOTE: All the functions MUST only do the tasks described above, nothing else. NOTE: Both classes MUST be written as described. Input Output 15564 24 25782 112 351512
Write two local classes named Pipe and Pool as described below. Then, write a main function that reads the flowSpeed and area of a Pipe objed (p) both as double values, main will also read the width, the height, and the length of a Pool object (gardenPool) all as double values. Finally, the inputPipe of gardenPool will be the p Pipe object. Finally, the program should calculate the time it takes the gardenPool to be completely filled will water and prints it to the screen as a double value. Pipe Class • A private double field named "flowSpeed" representing the flow speed of the pipe. • A private double field named "area" representing the cross-section area (ie, kesit alan) of the pipe. * A constructor that gets two double values and initializes the object. An emply constructor. • A public function named calculateFlowRate that does not get any parameter, multiplies the flowSpeed and area of the pipe, and returns it as a double value. Pool Class • A private double field named "width" representing the width of the pool. A private double field named "height" representing the height of the pool. A private double field named "length" representing the length of the pool. A private Pipe field named "inputPipe" representing the pipe that provides water to this pool. A constructor that gets three double values, a Pipe value, and initializes the object. • A public function named calculateVolume that does not get any parameter, calculates the volume of the pool using the rectangular prism volume formula given below and returns it as a double value: Volume-height"width* length • A public function named calculateTimeToFill that does not get any parameter, calculates the time it takes for the pool to be filled with water from the pipe by dividing the volume of the pool by the flow rate of the pipe and returns it as a double value. The main function will, 1. Instantiate one Pipe object called p and instantiate a Pool object called gardenPool as explained above. 2. Use the calculateTimeToFill method of the Pool class to print out the time it takes to fill the pool and print it out. NOTE: flowSpeed and area will always be given as positive values. NOTE: All the functions MUST only do the tasks described above, nothing else. NOTE: Both classes MUST be written as described. Input Output 15564 24 25782 112 351512
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 9PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
quick and fast plz
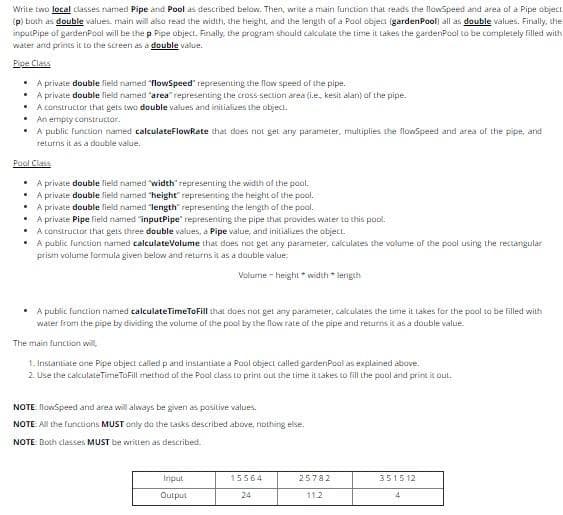
Transcribed Image Text:Write two local classes named Pipe and Pool as described below. Then, write a main function that reads the flowSpeed and area of a Pipe object
(p) both as double values, main will also read the width, the height, and the length of a Pool object (gardenPool) all as double values. Finally, the
inputPipe of gardenPool will be the p Pipe object. Finally, the program should calculate the time it takes the gardenPool to be completely filled with
water and prints it to the screen as a double value.
Pipe Class
• A private double field named "flowSpeed" representing the flow speed of the pipe.
• A private double field named "area" representing the cross-section area (i.e, kesit alan) of the pipe.
•
A constructor that gets two double values and initializes the object.
An emply constructor.
•
A public function named calculateFlowRate that does not get any parameter, multiplies the flowSpeed and area of the pipe, and
returns it as a double value.
Pool Class
•
A private double field named "width" representing the width of the pool.
•
A private double field named "height" representing the height of the pool.
• A private double field named "length representing the length of the pool.
•
A private Pipe field named "inputPipe representing the pipe that provides water to this pool.
.
A construct that gets three double values, a Pipe value, and initializes the object.
.
A public function named calculateVolume that does not get any parameter, calculates the volume of the pool using the rectangular
prism volume formula given below and returns it as a double value:
Volume-height*width* length
• A public function named calculateTimeToFill that does not get any parameter, calculates the time it takes for the pool to be filled with
water from the pipe by dividing the volume of the pool by the flow rate of the pipe and returns it as a double value.
The main function will
1. Instantiate one Pipe object called p and instantiate a Pool object called gardenPool as explained above.
2. Use the calculateTimeToFill method of the Pool class to print out the time it takes to fill the pool and print it out.
NOTE: flowSpeed and area will always be given as positive values.
NOTE: All the functions MUST only do the tasks described above, nothing else.
NOTE. Both classes MUST be written as described.
Input
Output
15564
24
25782
11.2
351512
4
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
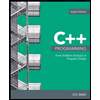
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
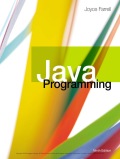
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
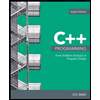
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
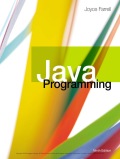
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT