// Write your code for Ex 6.1 above this line. // Ex 6.2 // // Write a function called "print_rand" which returns no result // and takes, as a single argument, a pointer to an unsigned 32-bit // integer.
// Write your code for Ex 6.1 above this line. // Ex 6.2 // // Write a function called "print_rand" which returns no result // and takes, as a single argument, a pointer to an unsigned 32-bit // integer.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
// Ex 6.1
//
// Write a function prototype for a function called "next"
// which takes no parameters and returns no result.
// Write your code for Ex 6.1 above this line.
// Ex 6.2
//
// Write a function called "print_rand" which returns no result
// and takes, as a single argument, a pointer to an unsigned 32-bit
// integer.
//
// The function should inspect the least significant two bits
// of the integer pointed to by the argument, and depending on
// the value of the bits, execute one of the following function
// calls:
//
// 0b00: putchar('1');
// 0b01: putchar('2');
// 0b10: putchar('3');
// 0b11: putchar('4');
//
// Once you have written this function uncomment the second printf()
// statement in main() such that its operation can be tested.
// Write your code for Ex 6.2 above this line.
// Ex 6.3
//
// Complete below the implementation for the function next().
//
// next() should:
// 1) Right shift the bits in "state" by 1 bit position.
// 2) If the bit shifted out of state was set (1), take the XOR
// of "state" with 0xA8E831E0, and store the result in "state".
//
// Once you have written this function uncomment the third printf()
// statement in main() such that its operation can be tested.
void next(void) {
}
// Write your code for Ex 6.3 above this line.
// This is main(). It it the entry point for a C programme.
int main(void) {
serial_init();
// Uncomment the line below when Ex 6.0 is complete
//printf("Ex 6.0: state = 0x%08lX\n", state);
// Uncomment the line below when Ex 6.2 is complete
//uint32_t test = 0b1100; printf("Ex 6.2: output = "); print_rand(&test); test >>= 1; print_rand(&test); test >>= 1; print_rand(&test); test >>= 1; print_rand(&test); printf("\n");
// Uncomment the line below when Ex 6.3 is complete
//next(); printf("Ex 6.3: state = 0x%08lX", state); next(); next(); next(); next(); printf(", state = 0x%08lX\n", state);
// Ex 6.4
//
// The variable "state" together with the function next()
// you have written above implement a linear-feedback shift
// register, which can produce a pseudorandom number sequence.
// We seeded this sequence (i.e. set the initial value) with
// your student number at the start of the programme. The tests
// above have already made a few calls to next() so you should
// expect the value currently stored in "state" to be different
// from the initial seed.
//
// The function print_rand() you wrote above can be used to
// operate on the variable "state" to print a digit in the
// range 1-4.
//
// Write code below to print the next 32 digits (from 1-4)
// derived from this pseudorandom number sequence using
// next() and print_rand(). All the digits should be printed
// on a single line without spaces. Note: you should call next()
// to advance "state" prior to printing the first digit.
//
// Tip: You will likely want to use a loop to complete the required
// number of repeated function calls.
//
// Tip: Recall that the unary operator "&" can be used to get a
// reference (pointer) to some data.
printf("Ex 6.4: ");
// Write your code for Ex 6.4 below this line.
// Write your code for Ex 6.4 above this line.
printf("\n");
// END OF TUTORIAL06 EXERCISES //
// DO NOT EDIT BELOW THIS LINE //
while(1) {
// Loop forever
}
} // end main()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
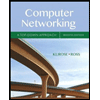
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
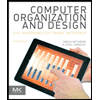
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
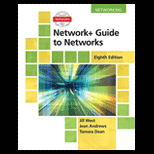
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
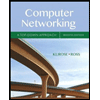
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
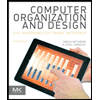
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
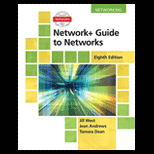
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
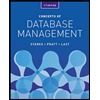
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
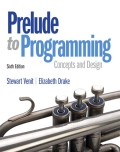
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
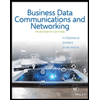
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY