X 1. write a program using an array to perform the sum and average for the zig zag elements shown in the above figure.
X 1. write a program using an array to perform the sum and average for the zig zag elements shown in the above figure.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 15PE
Related questions
Question
Please do number 1 using C language
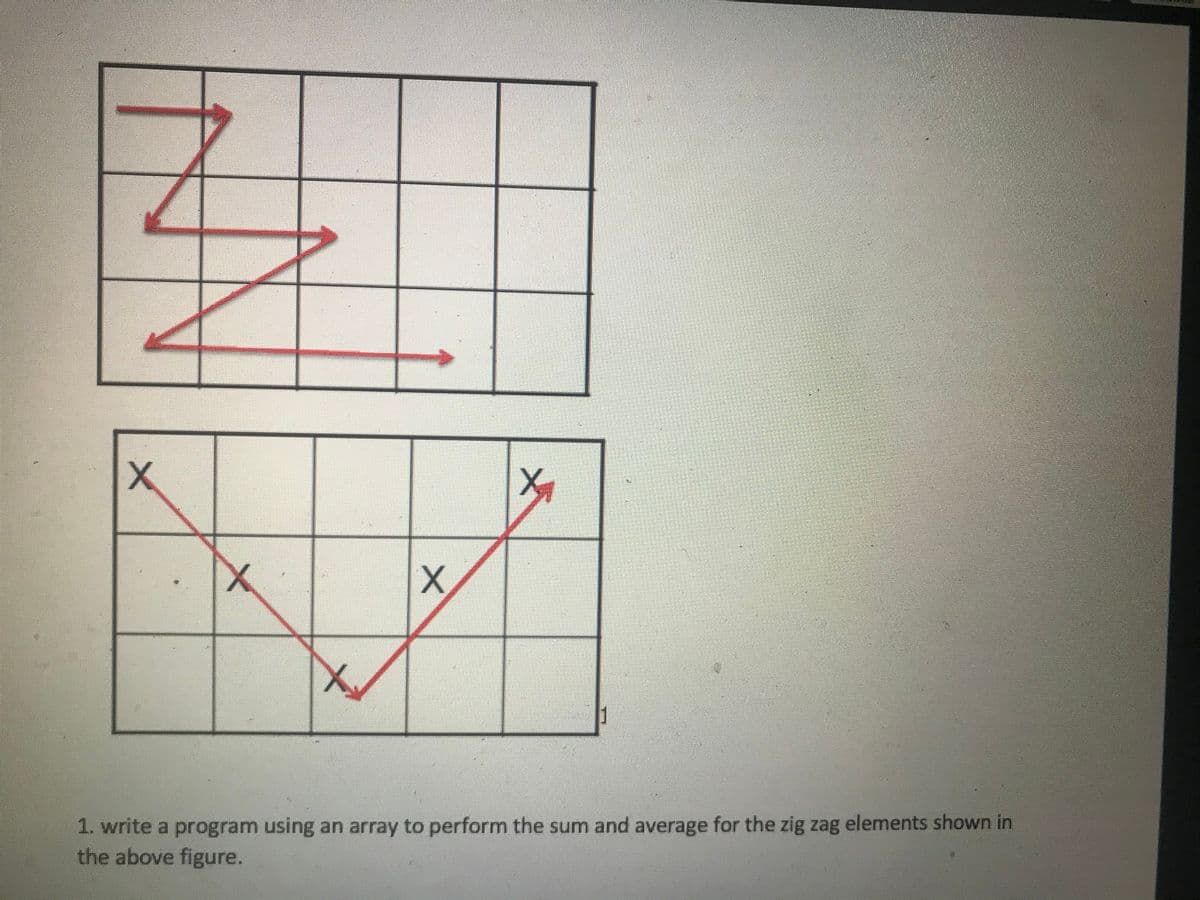
Transcribed Image Text:1
1. write a program using an array to perform the sum and average for the zig zag elements shown in
the above figure.
Expert Solution

Step 1
List of variables used:
- Considering an array "arr" of rows: 3 and columns: 5.
- Variables i,j,k for looping purpose.
- Array sum[2]: to store the sum of given figures zigzag elements.
- Array avg[2]: to store the average of zigzag elements given figures.
- Array count[2]: To count the number of elements in zigzag
Finding and calculating sum and average of first zigzag elements:
- variables i and j are used for accessing elements in the array.
- Where i represents the row.
- where j represents the column.
- Initialize i=0, j=0 means accessing first-row first-element.
- Loop for accessing rows begins with termination condition i<3
- Loop for accessing columns begins with termination condition j<=(i+1)
- Adding arr[i][j] to sum[0].
- sum[0] += arr[i][j]
- Increase count value by 1.
- count[0]++
- Increase j value by 1.
- If the termination condition j<=(i+1) satisfies, exit from the loop. Otherwise, repeat the loop.
- Adding arr[i][j] to sum[0].
- Increase value of i by 1.
- If the termination condition i<3 satisfies, exit from the loop. Otherwise, repeat the loop.
- Loop for accessing columns begins with termination condition j<=(i+1)
- Calculating average of zigzag elemnts.
- avg[0]=sum[0]/count[0]
Finding and calculating sum and average of second zigzag elements:
- variables i and j are used for accessing elements in the array.
- Where i represents the row.
- where j represents the column.
- Initialize i=0, j=0 and k=4.
- Loop for accessing rows begins with termination condition i<3
- Loop for accessing columns begins with termination condition j<5
- if value of i equals to j ( j == i) then
- Adding arr[i][j] to sum[1].
- sum[1] += arr[i][j]
- Increase count value by 1.
- count[1]++
- Adding arr[i][j] to sum[1].
- if value of k equals to j ( j == k) and value of i not equals to j (j != i) then
- Adding arr[i][j] to sum[1].
- sum[1] += arr[i][j]
- Increase count value by 1.
- count[1]++
- Adding arr[i][j] to sum[1].
- Increase j value by 1.
- If the termination condition j<5 satisfies, exit from the loop. Otherwise, repeat the loop.
- if value of i equals to j ( j == i) then
- Increase value of i by 1.
- decrement value of k by 1.
- If the termination condition i<3 satisfies, exit from the loop. Otherwise, repeat the loop.
- Loop for accessing columns begins with termination condition j<5
- Calculating average of zigzag elemnts.
- avg[1]=sum[1]/count[1]
Step 2
CODE:
#include <stdio.h>
int main()
{
// Declaring variables for array, sum, average and looping.
int arr[3][5], sum[2] = {0};
float avg[2] = {0};
int i, j, k, count[2] = {0};
//Reading array elements
printf("Enter Array element of size 3 * 5:");
for (i = 0; i < 3; i++)
{
printf("\nEnter %d row 5 elements:\n", i + 1);
for (j = 0; j < 5; j++)
{
scanf("%d", &arr[i][j]);
}
}
//Displaying Array elements
printf("\nArray elements:");
for (i = 0; i < 3; i++)
{
printf("\n");
for (j = 0; j < 5; j++)
{
printf("%d\t", arr[i][j]);
}
}
// calculating sum and average of first zigzag elements
//Displatying First set of zigzag elements
printf("\nFirst zigzag puzzle:");
for (i = 0; i < 3; i++)
{
printf("\n");
for (j = 0; j <= (i + 1); j++)
{
printf("%d\t", arr[i][j]);
sum[0] += arr[i][j];
count[0]++;
}
}
avg[0] = (float)sum[0] / count[0];
// calculating sum and average of second zigzag elements
//Displatying second set of zigzag elements
printf("\nSecond zigzag puzzle:");
for (i = 0, k = 4; i < 3; i++, k--)
{
printf("\n");
for (j = 0; j < 5; j++)
{
if (j == i)
{
printf("%d", arr[i][j]);
sum[1] += arr[i][j];
count[1]++;
}
if (j == k && i != j)
{
printf("%d", arr[i][j]);
sum[1] += arr[i][j];
count[1]++;
}
printf(" \t");
}
}
avg[1] = (float)sum[1] / count[1];
//Dsipalying sum and averages of both first and second set of zigzag elements.
printf("\nFirst zigzag puzzle:");
printf("\n\tSum : %d", sum[0]);
printf("\n\tAverage : %f", avg[0]);
printf("\nSecond zigzag puzzle:");
printf("\n\tSum : %d", sum[1]);
printf("\n\tAverage : %f", avg[1]);
}
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
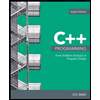
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
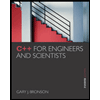
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
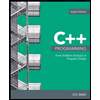
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
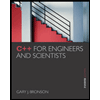
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
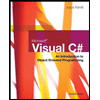
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,