X435: Generics - Comparable Cage Modify the Cage class to implement Comparable. The definition of the Comparable interface can be found here. Remember that Comparable has one abstract method, int compareTo (T o). Two Cages can be compared based on their size obtained via getSize(). You must (1) implement the interface, and (2) define the compareTo method. Your Answer: Feedback 1 class Cage implements Comparable> { private int size; private P pet; 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 23 24 25 26 26 27 27 28 29 30 31 32 33 34 35 36} 37 public Cage (P p, int s) { size = s; pet = p; } public boolean checkSize() { if(pet.getSize() > size) { return false; } public int getSize() { return size; } } return true; public P getPet() { return pet; } public int compareTo (Cage other) { } if(this.getSize() > other.getSize()) { return 1; } else if(this.getSize() > other.getSize()) { return -1; } else { } return 0; Result Behavior ✓ ✔ - ✓ ✔ size2Cage.compare To(size 1 Cage) > 0 -> true size4Cage.compare To(size 5Cage) > 0 -> false sizeNeg3Cage.compare To(size0Cage) < 0 -> true Expected: but was: size2Cage.compare To(size1 Cage) < 0 -> false size2Cage.compare To (otherSize2Cage) == 0 -> true size2Cage.compare To(size 1 Cage) == 0 -> false
X435: Generics - Comparable Cage Modify the Cage class to implement Comparable. The definition of the Comparable interface can be found here. Remember that Comparable has one abstract method, int compareTo (T o). Two Cages can be compared based on their size obtained via getSize(). You must (1) implement the interface, and (2) define the compareTo method. Your Answer: Feedback 1 class Cage implements Comparable> { private int size; private P pet; 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 23 24 25 26 26 27 27 28 29 30 31 32 33 34 35 36} 37 public Cage (P p, int s) { size = s; pet = p; } public boolean checkSize() { if(pet.getSize() > size) { return false; } public int getSize() { return size; } } return true; public P getPet() { return pet; } public int compareTo (Cage other) { } if(this.getSize() > other.getSize()) { return 1; } else if(this.getSize() > other.getSize()) { return -1; } else { } return 0; Result Behavior ✓ ✔ - ✓ ✔ size2Cage.compare To(size 1 Cage) > 0 -> true size4Cage.compare To(size 5Cage) > 0 -> false sizeNeg3Cage.compare To(size0Cage) < 0 -> true Expected: but was: size2Cage.compare To(size1 Cage) < 0 -> false size2Cage.compare To (otherSize2Cage) == 0 -> true size2Cage.compare To(size 1 Cage) == 0 -> false
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 10PE
Related questions
Question
100%
Please fix according to feedback
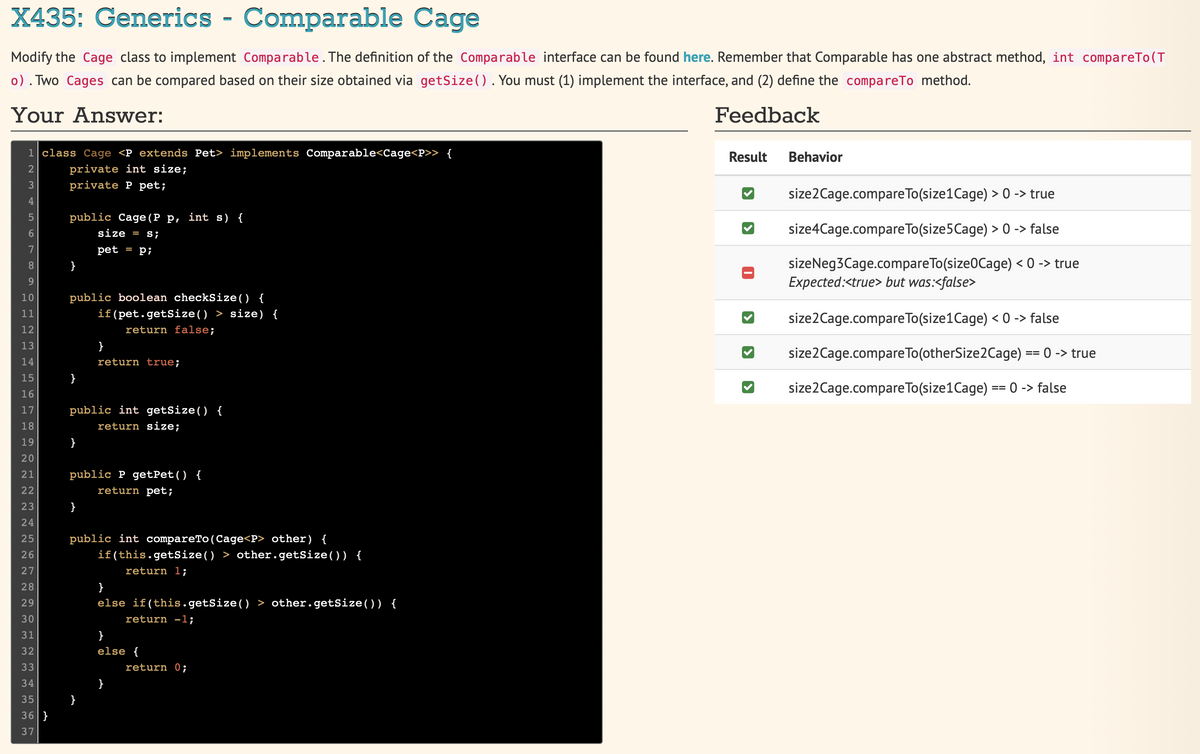
Transcribed Image Text:X435: Generics - Comparable Cage
Modify the Cage class to implement Comparable. The definition of the Comparable interface can be found here. Remember that Comparable has one abstract method, int compareTo(T
o). Two Cages can be compared based on their size obtained via getSize() . You must (1) implement the interface, and (2) define the compareTo method.
Your Answer:
Feedback
1 class Cage <P extends Pet> implements Comparable<Cage<P>> {
HNm gi Lo Lo -
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
HNN N N N N N N
23
24
25
26
27
28
29
30
31
32
33
34
35
36}
37
private int size;
private P pet;
public Cage (P p, int s) {
size = s;
pet = p;
public boolean checkSize() {
if(pet.getSize() > size) {
return false;
}
public int getSize() {
return size;
}
}
return true;
public P getPet() {
return pet;
}
public int compareTo (Cage<P> other) {
if(this.getSize() > other.getSize()) {
return 1;
}
}
else if(this.getSize() > other.getSize()) {
return -1;
}
else {
}
return 0;
Result
›
Behavior
size2Cage.compareTo(size1 Cage) > 0 -> true
size4Cage.compare To(size 5 Cage) > 0 -> false
sizeNeg3Cage.compareTo(size0Cage) < 0 -> true
Expected:<true> but was:<false>
size2Cage.compareTo(size1Cage) < 0 -> false
size2Cage.compare To (otherSize2Cage) == 0 -> true
size2Cage.compareTo(size1Cage) =
==
0 -> false
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
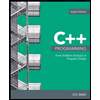
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
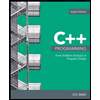
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning