private Purchase [] orders; private class Node{ Purchase data; Node next; public Node (Purchase value) { data = value; next = null; }} private Node head; private int length; public PurchaseList() { length = 0; head = null; }
private Purchase [] orders; private class Node{ Purchase data; Node next; public Node (Purchase value) { data = value; next = null; }} private Node head; private int length; public PurchaseList() { length = 0; head = null; }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
how do i add the purchase instances to the front of the list and is my deep copy method correct for a singly linked list
![public class PurchaseList {
private Purchase[] orders;
private class Node{
Purchase data;
Node next;
public Node (Purchase value) {
data = value;
next = null;
}
}
private Node head;
private int length;
public PurchaseList() {
length = 0;
head = null;
}
Purchase List (Purchase List other) {
Node otherNode = head;
Purchase purchase = new Purchase (otherNode.data);
Node newNode = new Node (purchase);
this.orders = new Purchase [other.orders.length];
this.length = other.length;
for (int i = 0; i < other.length; i++) {
orders[i].itemName = other.orders[i].itemName;
orders[i].itemPrice other.orders[i].itemPrice;
orders[i].quantity = other.orders[i].quantity;
}
this.head = other.head;
this.length = other.length;
}
public void add (Purchase p) {
Node temp;
System.out.println(temp.p);
temp = new Node();
Purchase.p = temp.p;
temp.next = head;
head = temp;
length++;
}
public void add (Purchase List pl) {
if(head
==
null) {
new Node (pl);
}
head
return;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8c247e94-aadc-4ba9-befa-a63b46c0c923%2F8a855606-8bee-479e-a8d2-28a1f0fe45ec%2Fqyvahzr_processed.png&w=3840&q=75)
Transcribed Image Text:public class PurchaseList {
private Purchase[] orders;
private class Node{
Purchase data;
Node next;
public Node (Purchase value) {
data = value;
next = null;
}
}
private Node head;
private int length;
public PurchaseList() {
length = 0;
head = null;
}
Purchase List (Purchase List other) {
Node otherNode = head;
Purchase purchase = new Purchase (otherNode.data);
Node newNode = new Node (purchase);
this.orders = new Purchase [other.orders.length];
this.length = other.length;
for (int i = 0; i < other.length; i++) {
orders[i].itemName = other.orders[i].itemName;
orders[i].itemPrice other.orders[i].itemPrice;
orders[i].quantity = other.orders[i].quantity;
}
this.head = other.head;
this.length = other.length;
}
public void add (Purchase p) {
Node temp;
System.out.println(temp.p);
temp = new Node();
Purchase.p = temp.p;
temp.next = head;
head = temp;
length++;
}
public void add (Purchase List pl) {
if(head
==
null) {
new Node (pl);
}
head
return;

Transcribed Image Text:public class Purchase {
String itemName;
double quantity;
double itemPrice;
public Purchase(){
}
itemName="empty";
quantity=0.0;
itemPrice=0.0;
}
|
public Purchase(String i, double q, double ip){
this.itemName=1;
this.quantity-q;
this.itemPrice=ip;
}
public Purchase (Purchase other){
this.itemName-other.itemName;
this.quantity-other.quantity;
this.itemPrice-other.itemPrice;
}
public String getItemName() {
return itemName;
}
public double getQuantity(){
return quantity;
}
public double getItemPrice(){
return itemPrice;
}
public void setItemName(String itemName) {
this.itemName itemName;
}
public void setQuantity (double quantity){
this.quantity = quantity;
}
public void setItemPrice(double itemPrice){
this.itemPrice = itemPrice;
}
public double cost() {
}
double cost-itemPrice quantity;
return cost;
public Purchase makeCopy(){
}
Purchase P = new Purchase();
itemName P.itemName;
itemPrice= P.itemPrice;
quantity= P.quantity;
return P;
@Override
public boolean equals(Object obj){
if(obj ==this){
return true; }
if(!(obj instanceof Purchase))
{return false;}
Purchase p (Purchase)obj;
return Double.compare(itemPrice,p.itemPrice) == 0 && itemName.equals(p.itemName) && quantity == p.quantity;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
yea but how do i implement it in java that's my issue i don't know how to code it
Solution
Recommended textbooks for you
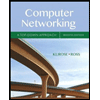
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
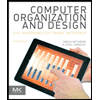
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
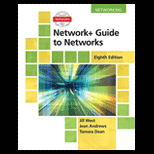
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
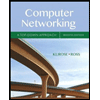
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
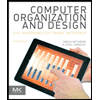
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
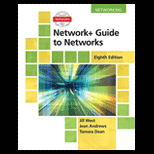
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
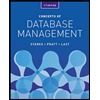
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
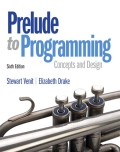
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
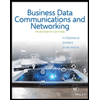
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY