You are expected to work on your own Preferred visual studio, but you can use repl as well. • Must make sure to compile, build and run the code and program should be (a) compilation error free and (b) logical error free. • Must Validate the program with different types of data (both data arrangements and type of data). • Must attach the results along with your source code. Assignment: Write four different functions: a) void SelectionSort(Data "A, int N), where A is the array and N is number of data in the array. Data is the datatype, which you can declare first as a global declaration, by using typedef int Data; This helps by changing the datatype of the data to be sorted casily. b) int Min(Data *A, int start, int N); // this will return the index min, for the smallest data from the data set Afstart] to A[N-1] e) void InsertionSort(Data A, int N): d) void PrintArray(Data * A, int N), when called this function will display all the data in the array A Also, write the driver function (i.e. the main), main should do the following: i) Declare the array dynamically, so that memory can be used efficiently. To declare an array dynamically, the following steps may be used: • Declare a pointer of type Data Data * A: • Declare number of data N, and read a value for N • Allocate memory A= new Data (N] ii) Prompt the user to enter N number of data, and store those data in the array, by using a loop and cin statement to read the data. iii) Declare a variable sortType as integer and ask user to enter a value for sort Type.
You are expected to work on your own Preferred visual studio, but you can use repl as well. • Must make sure to compile, build and run the code and program should be (a) compilation error free and (b) logical error free. • Must Validate the program with different types of data (both data arrangements and type of data). • Must attach the results along with your source code. Assignment: Write four different functions: a) void SelectionSort(Data "A, int N), where A is the array and N is number of data in the array. Data is the datatype, which you can declare first as a global declaration, by using typedef int Data; This helps by changing the datatype of the data to be sorted casily. b) int Min(Data *A, int start, int N); // this will return the index min, for the smallest data from the data set Afstart] to A[N-1] e) void InsertionSort(Data A, int N): d) void PrintArray(Data * A, int N), when called this function will display all the data in the array A Also, write the driver function (i.e. the main), main should do the following: i) Declare the array dynamically, so that memory can be used efficiently. To declare an array dynamically, the following steps may be used: • Declare a pointer of type Data Data * A: • Declare number of data N, and read a value for N • Allocate memory A= new Data (N] ii) Prompt the user to enter N number of data, and store those data in the array, by using a loop and cin statement to read the data. iii) Declare a variable sortType as integer and ask user to enter a value for sort Type.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.5: Case Studies
Problem 6E: (List maintenance) The following letters are stored in an alphabet array: B, J, K, M, S, and Z....
Related questions
Question
![Instructions for the lab:
You are expected to work on your own. Preferred visual studio,
but you can use repl as well.
• Must make sure to compile, build and run the code and
program should be (a) compilation error free and (b) logical
error free.
• Must Validate the program with different types of data (both
data arrangements and type of data).
• Must attach the results along with your source code.
Assignment:
Write four different functions:
a) void SelectionSort(Data *A, int N), where A is the array
and N is number of data in the array. Data is the datatype,
which you can declare first as a global declaration, by using
typedef int Data;
This helps by changing the datatype of the data to be sorted
easily.
b) int Min(Data *A, int start, int N): / this will return the
index min, for the smallest data from the data set A[start] to
A[N-1]
e) void InsertionSort(Data * A, int N):
d) void PrintArray(Data • A, int N): / when called this
function will display all the data in the array A
Also, write the driver function (i.c. the main), main should
do the following:
i) Declare the array dynamically, so that memory can be
used efficiently.
To declare an array dynamically, the following steps
may be used:
• Declare a pointer of type Data
Data * A:
• Declare number of data N, and read a value for N
Allocate memory
A = new Data (N]
i) Prompt the user to enter N number of data, and store
those data in the array, by using a loop and cin
statement to read the data.
i) Declare a variable sortType as integer and ask user to
enter a value for sort Type.
(sortType = 1 for selection sort, = 2 for insertion sort)
iv) Write a switch statement using sort Type as controlling](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc6f3d9e4-0018-4fd7-a57a-62424510b6b8%2F5309069a-b867-4bfa-b462-dad110ba5fc4%2F2j06byn_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Instructions for the lab:
You are expected to work on your own. Preferred visual studio,
but you can use repl as well.
• Must make sure to compile, build and run the code and
program should be (a) compilation error free and (b) logical
error free.
• Must Validate the program with different types of data (both
data arrangements and type of data).
• Must attach the results along with your source code.
Assignment:
Write four different functions:
a) void SelectionSort(Data *A, int N), where A is the array
and N is number of data in the array. Data is the datatype,
which you can declare first as a global declaration, by using
typedef int Data;
This helps by changing the datatype of the data to be sorted
easily.
b) int Min(Data *A, int start, int N): / this will return the
index min, for the smallest data from the data set A[start] to
A[N-1]
e) void InsertionSort(Data * A, int N):
d) void PrintArray(Data • A, int N): / when called this
function will display all the data in the array A
Also, write the driver function (i.c. the main), main should
do the following:
i) Declare the array dynamically, so that memory can be
used efficiently.
To declare an array dynamically, the following steps
may be used:
• Declare a pointer of type Data
Data * A:
• Declare number of data N, and read a value for N
Allocate memory
A = new Data (N]
i) Prompt the user to enter N number of data, and store
those data in the array, by using a loop and cin
statement to read the data.
i) Declare a variable sortType as integer and ask user to
enter a value for sort Type.
(sortType = 1 for selection sort, = 2 for insertion sort)
iv) Write a switch statement using sort Type as controlling
![Assignment:
Write four different functions:
a) void SelectionSort(Data *A, int N), where A is the array
and N is number of data in the array. Data is the datatype,
which you can declare first as a global declaration, by using
typedef int Data;
This helps by changing the datatype of the data to be sorted
easily.
b) int Min(Data *A, int start, int N); // this will retun the
index min, for the smallest data from the data set A[start] to
A[N-1]
c) void InsertionSort(Data A, int N):
d) void PrintArray(Data * A, int N); // when called this
function will display all the data in the array A
Also, write the driver function (i.e. the main), main should
do the following:
i) Declare the array dynamically, so that memory can be
used efficiently.
To declare an array dynamically, the following steps
may be used:
• Declare a pointer of type Data
Data * A;
• Declare number of data N, and read a value for N
• Allocate memory
A = new Data [N]
ii) Prompt the user to enter N number of data, and store
those data in the array, by using a loop and cin
statement to read the data.
ii) Declare a variable sortType as integer and ask user to
enter a value for sortType.
(sortType = 1 for selection sort, = 2 for insertion sort)
iv) Write a switch statement using sortType as controlling
parameter, and each case is designed to call the proper
algorithm (Case 1, call selection sort, and then call the
PrintArray function, case 2: call the insertion sort and
then call the PrintArray function.
MUST DEFINE EACH OF THE FOUR functions properly. You
can take help from the power point notes to complete this
еxercise.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc6f3d9e4-0018-4fd7-a57a-62424510b6b8%2F5309069a-b867-4bfa-b462-dad110ba5fc4%2Fk3mufm8_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Assignment:
Write four different functions:
a) void SelectionSort(Data *A, int N), where A is the array
and N is number of data in the array. Data is the datatype,
which you can declare first as a global declaration, by using
typedef int Data;
This helps by changing the datatype of the data to be sorted
easily.
b) int Min(Data *A, int start, int N); // this will retun the
index min, for the smallest data from the data set A[start] to
A[N-1]
c) void InsertionSort(Data A, int N):
d) void PrintArray(Data * A, int N); // when called this
function will display all the data in the array A
Also, write the driver function (i.e. the main), main should
do the following:
i) Declare the array dynamically, so that memory can be
used efficiently.
To declare an array dynamically, the following steps
may be used:
• Declare a pointer of type Data
Data * A;
• Declare number of data N, and read a value for N
• Allocate memory
A = new Data [N]
ii) Prompt the user to enter N number of data, and store
those data in the array, by using a loop and cin
statement to read the data.
ii) Declare a variable sortType as integer and ask user to
enter a value for sortType.
(sortType = 1 for selection sort, = 2 for insertion sort)
iv) Write a switch statement using sortType as controlling
parameter, and each case is designed to call the proper
algorithm (Case 1, call selection sort, and then call the
PrintArray function, case 2: call the insertion sort and
then call the PrintArray function.
MUST DEFINE EACH OF THE FOUR functions properly. You
can take help from the power point notes to complete this
еxercise.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
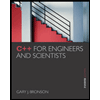
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
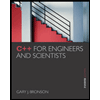
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr