You are to automate some repetitive tasks. The tasks are as follows: 1) Add a new product to the queue. 2) Deliver the next product of the queue and print the product information delivered. 3) Query how many products of a given manufacturer is currently present in the queue. 4) Query how many products of a given manufacturer has been shipped already. Initially, the product queue is empty. It is also guaranteed that when new products are added, all information is consistent, i.e., it is a valid product from a valid partner manufacturer.
You are approached by an online delivery shipping firm, which asks you to write them code for some tasks they face. The products they ship have some attributes, which are as follows:
- Product ID (Integer) [This is unique for every product]
- Product label (String)
- Manufacturer (String)
All strings are of maximum length 100 and contain only alphanumeric characters.
The products arrive one by one, and a common queue is maintained for all of them. Also, there is a fixed set of manufacturers the company has a tie-up with:
- Nike
- Adidas
- Reebok
- Puma
- Diadora
You are to automate some repetitive tasks. The tasks are as follows:
1) Add a new product to the queue.
2) Deliver the next product of the queue and print the product information delivered.
3) Query how many products of a given manufacturer is currently present in the queue.
4) Query how many products of a given manufacturer has been shipped already.
Initially, the product queue is empty. It is also guaranteed that when new products are added, all information is consistent, i.e., it is a valid product from a valid partner manufacturer.
**It is necessary to maintain the queue as a linked list.
Input Format
The first line contains an integer n, denoting the number of tasks to be performed. The following n lines can be of the following types:
- 1 Product_ID Product_label Manufacturer (Eg. 1 12 Bottle Puma)
- 2
- 3 Manufacturer (Eg. 3 Adidas)
- 4 Manufacturer (Eg. 4 Nike)
Output Format
We are supposed to take the following actions for each type of input from {1,2,3,4,5}
1) Insert the product with the given attributes at the back of the queue. Then print Product_ID ADDED (Eg. 23 ADDED)
2) If the queue is non empty, remove the product at the front of the queue and print all 3 attributes of the delivered product in a space separated manner. If queue is empty, print NOTHING TO DELIVER NOW
3) Print an integer corresponding to the answer (print -1 if the manufacturer is not a partner manufacturer)
4) Print an integer corresponding to the answer (print -1 if the manufacturer is not a partner manufacturer)
Note that all outputs are in a new line.
Example Input
6Example Output
NOTHING TO DELIVER NOWExplanation
Initially, queue is empty. So nothing to deliver initially.#include<stdio.h>
#include <stdlib.h>
struct node
{
int prod_ID;
char label[100];
char manufacturer[20];
struct node* next;
};
int main()
{
// Insert your code here.
return 0;
}
I WANT A CODE IN C.

Step by step
Solved in 2 steps

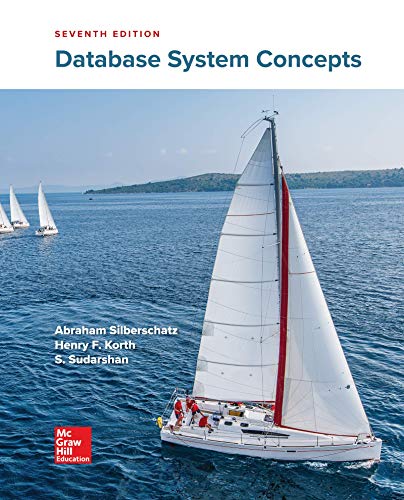
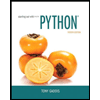
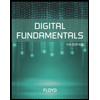
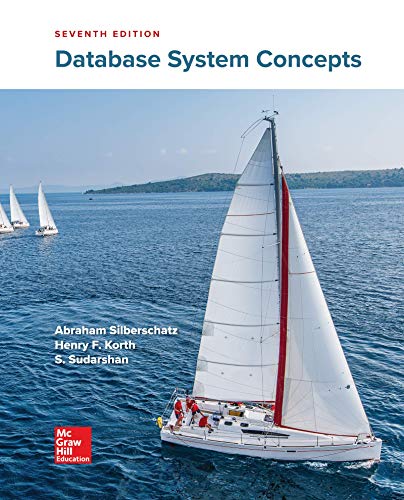
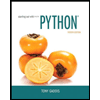
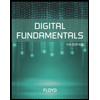
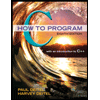
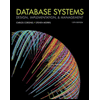
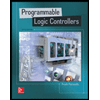