You should run your program using array sizes of 1,000, 10,000, and 100,000. Record the sort times. Add a comment to the top of the program that reports the times. (You might be interested in applying Arrays.sort() to a million-element array, but don't try that with Selection Sort or Insertion Sort!) Note: The general method for getting the run time of a code segment is: long startTime = System.currentTimeMillis(); doSomething(); long runTime = System.currentTimeMillis() - startTime; This gives the run time in milliseconds. If you want the time in seconds, you can use runTime/1000.0.
Kindly help as my inputs are not giving me the required OUTPUT. KINDLY SLOT THE INPUT.
You should run your program using array sizes of 1,000, 10,000, and 100,000. Record the sort times. Add a comment to the top of the program that reports the times. (You might be interested in applying Arrays.sort() to a million-element array, but don't try that with Selection Sort or Insertion Sort!)
Note: The general method for getting the run time of a code segment is:
long startTime = System.currentTimeMillis();
doSomething();
long runTime = System.currentTimeMillis() - startTime;
This gives the run time in milliseconds. If you want the time in seconds, you can use runTime/1000.0.
![eciipse-workspace - Lab1/src/iviain.java - ECilipse iDE
File Edit Source Refactor Navigate Search Project Run Window Help
*- O- Q
,や
A Package Explorer X E Project Explorer
v Lab1
> JRE System Library [JavaSE-15]
D MathQuizjava
D TextlO.java
D AdditionProblem.java
D Main.java 8
E Outline 3
- ILW LIILL AL
69
70 for(int i = 0;i<arr.length;i++) {
71
72 arr[i] = (int) (Math.random() * 100);
73
74 }
75
76 long startTime = System.currentTimeMillis();
77
78 selectionSort(arr);
79
80 long runTime = System.currentTimeMillis() - startTime;
81
82 System.out.println("\nRun time of the Selection sort is:"+runTime/1000.0+" Seconds");
83
84 long startTimel = System.currentTimeMillis();
85
86 sortByInBuilt(arr);
87
88 long runTimel = System.currentTimeMillis()-startTimel;
89
90 System.out.println("\nRun time of the In Built Sort method: "+runTimel/1000.0+" Seconds");
91
92 printArrayBySelectionSort(arr);
93
94 }
v 9. Main
oS selectionSort(int[]) : void
os printArrayBySelectionSort(int[])
o$ sortBylnBuilt(int[]) : void
main(String[) : void
v src
v # (default package)
> D AdditionProblem.java
> D Main.java
> D MathQuiz.java
> D TextlO.java
95
96 }
O Problems @ Javadoc e Declaration e Console 3
目關 回
Main [Java Application] C:\Users\user\.p2\pool\plugins\org.eclipse.justj.openjdk.hotspot.jre.full.win32.x86_64_15.0.2.v20210201-0955\jre\bin\javaw.exe (Jun 23, 2021, 11:24:24 PM)
Enter the limit of the array:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5dd53dd1-02d0-442f-939d-39f076a6afbe%2F805a13c9-b3a3-405f-a65b-44b6ef91328d%2Fcg2x51k_processed.png&w=3840&q=75)
![e eclipse-workspace - Lab1/src/Main.java - Eclipse IDE
File Edit Source Refactor Navigate Search Project Run Window Help
国 T: ▼ ,や
母
E Package Explorer 3 E Project Explorer
回名 。
D MathQuizjava
D TextIO.java
D AdditionProblem.java
D Main.java 3
E Outline X
v Lab1
EA JRE System Library [JavaSE-15]
1
20 import java.util.Arrays;]
v 9, Main
OS
v A src
selectionSort(int([]) : void
OS printArrayBySelectionSort(int[]
O$ sortBylnBuilt(int[]) : void
O main(String[]) : void
6 public class Main {
v E (default package)
> D AdditionProblem.java
> D Main.java
> D MathQuiz.java
> D Textl0.java
7
8e public static void selectionSort(int arr[])
10 {
11
12 int n = arr.length;
13
14 // One by one move boundary of unsorted subarcay
15
16 for (int i = 0; i < n-1; i++) Rectangular Snip
17
18 {
19
20 // Find the minimum element in unsorted array
21
22 int min_idx = i;
23
24 for (intj = i+1; j < n; j++)
25
26 if (arr[j] < arr[min_idx])
27
28 min_idx = j;
29
30
// Swap the found minimum element with the first
R Problems @ Javadoc e Declaration e Console X
O X * B EI N 9 - - e - - - E
Main [Java Application] C:\Users\user\.p2\pool\plugins\org.eclipse.justj.openjdk.hotspot.jre.full.win32.x86_64_15.0.2.v20210201-0955\jre\bin\javaw.exe (Jun 23, 2021, 11:24:24 PM)
Enter the limit of the array:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5dd53dd1-02d0-442f-939d-39f076a6afbe%2F805a13c9-b3a3-405f-a65b-44b6ef91328d%2Felxu9jt_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

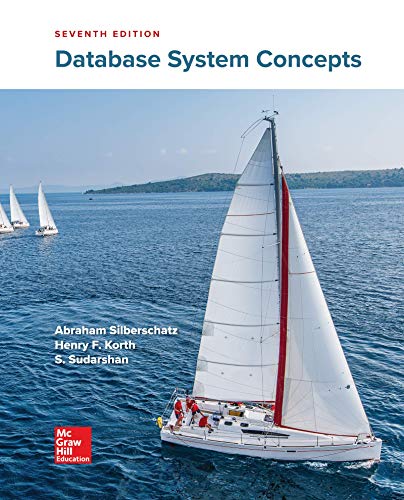
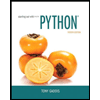
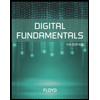
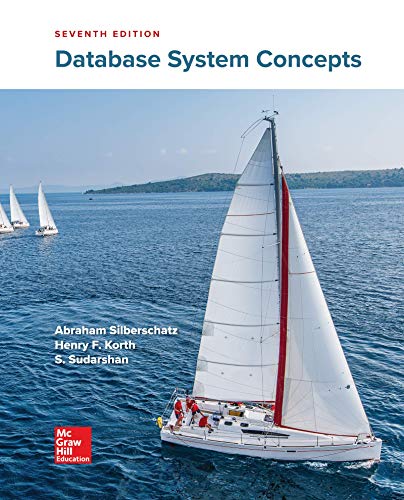
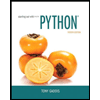
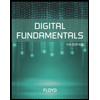
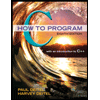
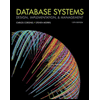
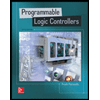