you will be identifying which organisms are the tastiest. The tastiest organisms are the ones that appear the most times as a food source. For example if aquatic.txt was the input file, file_list will be: ["Bird,Prawn,Mussels,Crab,Limpets,Whelk\n", "Crab,Mussels,Limpets\n", "Fish,Prawn\n", "Limpets,Seaweed\n", "Lobster,Crab,Mussels,Limpets,Whelk\n", "Mussels,Phytoplankton,Zooplankton\n", "Prawn,Zooplankton\n", "Whelk,Limpets,Mussels\n", "Zooplankton,Phytoplankton"] Limpets and mussels appear the most times as a food source, making them the tastiest organisms. Here is a sample output using aquatic.txt: Enter the food web file name: aquatic.txt Predators and Prey: Bird eats Crab, Limpets, Mussels, Prawn, and Whelk Crab eats Limpets and Mussels Fish eats Prawn Limpets eats Seaweed Lobster eats Crab, Limpets, Mussels, and Whelk Mussels eats Phytoplankton and Zooplankton Prawn eats Zooplankton Whelk eats Limpets and Mussels Zooplankton eats Phytoplankton Apex Predators: Bird, Fish, and Lobster Producers: Phytoplankton and Seaweed Most Flexible Eaters: Bird Tastiest: Limpets and Mussels Once again, each animal is sorted alphabetically. my code: # TODO: Write your header comment here. # DO NOT REMOVE file_name = input("Enter the food web file name: ") raw_file = open(file_name) file_list = raw_file.readlines() raw_file.close() file_list = [line.strip() for line in file_list] print("\n\nPredators and Prey:") all_prey = [] all_predators = [] predator_prey_count = {} prey_predator_count = {} tastiest_prey = [] for line in file_list: animals = line.split(',') predator = animals[0] all_predators.append(predator) predator = animals[0] prey = animals[1:] prey.sort() all_prey += prey predator_prey_count[predator] = len(prey) # prey_predator_count[prey] = len(predator) output = " " + predator + " eats " for i in range(len(prey)): if len(prey) == 1: output += prey[i] elif i == 0: output += prey[i] elif i == len(prey) - 1 and len(prey) -1 > 1: output += ", and " + prey[i] elif i == len(prey)-1 and len(prey) -1 == 1: output += " and " + prey[i] else: output += ", " + prey[i] prey_predator_count[prey] = len(predator) print(output) apex_predators = [predator for predator in all_predators if predator not in all_prey] apex_predators.sort() print("\nApex Predators:", end=" ") if len(apex_predators) == 1: print(apex_predators[0]) elif len(apex_predators) == 2: print(f"{apex_predators[0]} and {apex_predators[1]}") else: for i in range(len(apex_predators) - 1): print(apex_predators[i], end=", ") print(f"and {apex_predators[-1]}") producers = list(set(all_prey) - set(all_predators)) producers.sort() print("Producers:", end=" ") for i in range(len(producers)): if i == len(producers) - 1: print(f"{producers[i]}") else: print(producers[i], end=" and ") max_prey_count = 0 for predator, count in predator_prey_count.items(): if count > max_prey_count: max_prey_count = count most_flexible_eaters = [] for predator, count in predator_prey_count.items(): if count == max_prey_count: max_prey_count = count most_flexible_eaters.append(predator) most_flexible_eaters.sort() print("Most Flexible Eaters:", end=" ") if len(most_flexible_eaters) == 1: print(most_flexible_eaters[0]) elif len(most_flexible_eaters) == 2: print(f"{most_flexible_eaters[0]} and {most_flexible_eaters[1]}") else: for i in range(len(most_flexible_eaters) - 1): print(most_flexible_eaters[i], end=", ") print(f"and {most_flexible_eaters[-1]}") for predator, count in prey_predator_count.items(): if count == max_prey_count: max_prey_count = count tastiest_prey.append(prey) tastiest_prey.sort() print("Tastiest:", end = " ") if len(tastiest_prey) == 1: print(tastiest_prey[0]) elif len(tastiest_prey) == 2: print(f"{tastiest_prey[0]} and {tastiest_prey[1]}") else: for i in range(len(tastiest_prey) - 1): print(tastiest_prey[i], end = ", ") print(f"and {tastiest_prey[-1]}")
you will be identifying which organisms are the tastiest. The tastiest organisms are the ones that appear the most times as a food source.
For example if aquatic.txt was the input file, file_list will be:
["Bird,Prawn,Mussels,Crab,Limpets,Whelk\n", "Crab,Mussels,Limpets\n", "Fish,Prawn\n", "Limpets,Seaweed\n", "Lobster,Crab,Mussels,Limpets,Whelk\n", "Mussels,Phytoplankton,Zooplankton\n", "Prawn,Zooplankton\n", "Whelk,Limpets,Mussels\n", "Zooplankton,Phytoplankton"]
Limpets and mussels appear the most times as a food source, making them the tastiest organisms.
Here is a sample output using aquatic.txt:
Enter the food web file name: aquatic.txt
Predators and Prey: Bird eats Crab, Limpets, Mussels, Prawn, and Whelk Crab eats Limpets and Mussels Fish eats Prawn Limpets eats Seaweed Lobster eats Crab, Limpets, Mussels, and Whelk Mussels eats Phytoplankton and Zooplankton Prawn eats Zooplankton Whelk eats Limpets and Mussels Zooplankton eats Phytoplankton
Apex Predators: Bird, Fish, and Lobster
Producers: Phytoplankton and Seaweed
Most Flexible Eaters: Bird
Tastiest: Limpets and Mussels
Once again, each animal is sorted alphabetically.
my code:

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

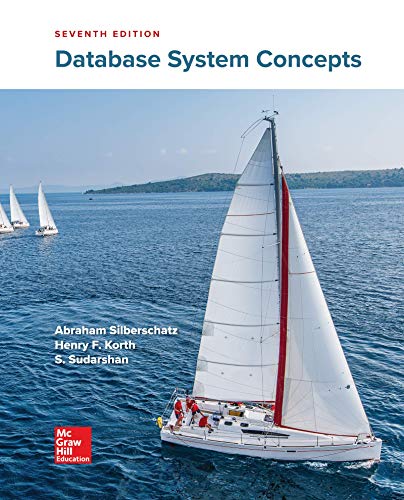
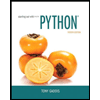
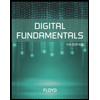
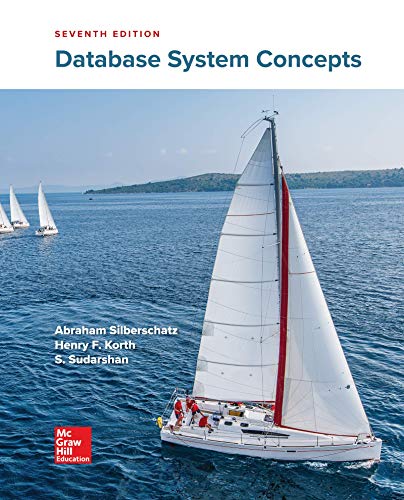
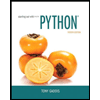
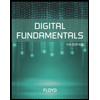
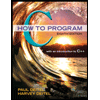
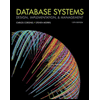
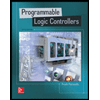