You will write a C++ program to process the lines in a text file using a linked list ADT and raw pointers. Node class You will create a class “Node” with the following private data attributes: line – line from a file (string) next - (pointer to a Node) Put your class definition in a header file and the implementation of the methods in a .cpp file. Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file. You will have the following public methods: Accessors and mutators for each attribute Constructor that initializes the attributes to nulls (empty string and nullptr)
You will write a C++ program to process the lines in a text file using a linked list ADT and raw pointers.
Node class
You will create a class “Node” with the following private data attributes:
-
line – line from a file (string)
-
next - (pointer to a Node)
Put your class definition in a header file and the implementation of the methods in a .cpp file.
Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file. You will have the following public methods:
-
Accessors and mutators for each attribute
Constructor that initializes the attributes to nulls (empty string and nullptr)
LinkedList class
You will create a class “LinkedList” with the following private data attributes:
-
headPtr – raw pointer to the head of the list
-
numItems – number of items in the list
Put your class definition in a header file and the implementation of the methods in a .cpp file.
Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file. You will have the following public methods:
-
Accessor to get the current size (numItems)
-
Constructor that initializes numItems to zero and headPtr to nullptr
-
Add a node – this method will take as input one string value. It will then create a node object and set the attribute. Then it will put it in the linked list at the correct position – ascending order – avoiding duplicates. You will do all of the work while building the linked list.
toVector – returns
Client program
Your program will ask the user for the file name, open the text file , read each line and invoke the addNode
method. Make sure the file opened correctly before you start processing it. You do not know how many lines are in the file so your program should read until it gets to the end of the file.
It will display the contents of the list (the lines that were read from the file in sorted order with no duplicates) using the LinkedList class method “toVector” that puts all of the lines into a vector and returns it to the program that will display it.
Your client program will do the following:
-
Read file name from user
-
Display the number of lines that were read out of the file and were passed to the addNode method.
-
Display the lines in your linked list using the class method toVector to get the lines.
Display the number of lines in the vector.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

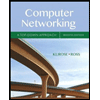
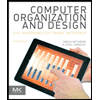
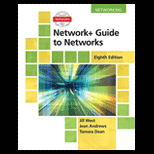
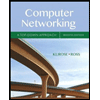
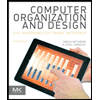
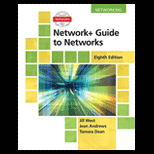
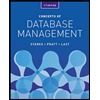
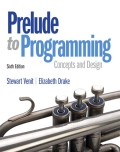
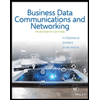