Your program must use the following five function headers exactly as they appear here: void getVectorSize(int& size); void readData(vector& scores); void sortData(vector& scores); vector::iterator findLocationOfLargest( const vector::iterator startingLocation, const vector::iterator endingLocation); void displayData(const vector& scores); The size parameter from the given code won't be needed now, since a vector knows its own size. using namespace std; const int MAX_NAME_SIZE = 24; struct Highscore{ char name[MAX_NAME_SIZE]; int score; }; void getArraySize(int& size); void readData(Highscore highScores[], int size); void sortData(Highscore highScores[], int size); int findIndexOfLargest(const Highscore highScores[], int startingIndex, int size); void displayData(const Highscore highScores[], int size); int main() { Highscore* highScores; int size; getArraySize(size); highScores = new Highscore[size]; readData(highScores, size); sortData(highScores, size); displayData(highScores, size); delete [] highScores; } void getArraySize(int& size){ cout << "How many scores will you enter?: "; cin >> size; cin.ignore(); } void readData(Highscore highScores[], int size) { for(int index = 0; index < size; index++) { cout << "Enter the name for score #" << (index + 1) << ": "; cin.getline(highScores[index].name, MAX_NAME_SIZE, '\n'); cout << "Enter the score for score #" << (index + 1) << ": "; cin >> highScores[index].score; cin.ignore(); } cout << endl; } void sortData(Highscore highScores[], int numItems) { for (int count = 0; count < numItems - 1; count++){ swap(highScores[findIndexOfLargest(highScores, count, numItems)], highScores[count]); } } int findIndexOfLargest(const Highscore highScores[], int startingIndex, int numItems){ int indexOfLargest = startingIndex; for (int count = startingIndex + 1; count < numItems; count++){ if (highScores[count].score > highScores[indexOfLargest].score){ indexOfLargest = count; } } return indexOfLargest; } void displayData(const Highscore highScores[], int size) { cout << "Top Scorers: " << endl; for(int index = 0; index < size; index++) { cout << highScores[index].name << ": " << highScores[index].score << endl; } } Notice that the findLocationOfLargest() function does not need the vector itself as a parameter, since you can access the vector using the provided iterator parameters. The name field in the struct must still be a c-string The focus of this assignment is to use iterators. You must use iterators wherever possible to access the vector. As a result, you must not use square brackets, the push_back() function, the at() function, etc. Also, the word "index" shouldn't appear in your code anywhere. You won't get full credit if you miss an opportunity to use iterators. You should still ask the user to enter the number of scores there will be, and then you should create a vector with the required capacity. You can do this by using the vector class's constructor that creates a vector with the capacity indicated by its parameter. For example, to create a vector of size 100, use this: vector myExampleVector(100); It is possible to write our program without bothering to indicate a size if we simply use push_back() to add each high score struct to the vector. We aren't doing it that way, because I want you to practice using iterators. You could sort the scores by simply calling the STL sort() algorithm. I would suggest that you try this out because it's something you should know, but for your submitted program you are required to sort the vector as it is done in the given code, except using iterators to access the items in the vector. You won't get credit for the assignment if you use the STL sort() function. In your displayData() function you'll need to use const_iterator instead of iterator. See lesson 19.4. Here is an example that shows how to use an iterator to access a particular member of a struct: swap((*iter1).firstmember, (*iter2).firstmember); This will work just fine, but there is a C++ operator that combines these two (dereference and then select). I works like this:swap(iter1 -> firstmember, iter2 -> firstmember); (Note that you won't actually use this example code, because when you swap, you should just swap the entire struct. There's no need to swap the individual members of the struct separately.
-
Your program must use the following five function headers exactly as they appear here:
void getVectorSize(int& size); void readData(vector <Highscore>& scores); void sortData(vector<Highscore>& scores); vector<Highscore>::iterator findLocationOfLargest( const vector<Highscore>::iterator startingLocation, const vector<Highscore>::iterator endingLocation); void displayData(const vector<Highscore>& scores); -
The size parameter from the given code won't be needed now, since a vector knows its own size.
using namespace std; const int MAX_NAME_SIZE = 24; struct Highscore{ char name[MAX_NAME_SIZE]; int score; }; void getArraySize(int& size); void readData(Highscore highScores[], int size); void sortData(Highscore highScores[], int size); int findIndexOfLargest(const Highscore highScores[], int startingIndex, int size); void displayData(const Highscore highScores[], int size); int main() { Highscore* highScores; int size; getArraySize(size); highScores = new Highscore[size]; readData(highScores, size); sortData(highScores, size); displayData(highScores, size); delete [] highScores; } void getArraySize(int& size){ cout << "How many scores will you enter?: "; cin >> size; cin.ignore(); } void readData(Highscore highScores[], int size) { for(int index = 0; index < size; index++) { cout << "Enter the name for score #" << (index + 1) << ": "; cin.getline(highScores[index].name, MAX_NAME_SIZE, '\n'); cout << "Enter the score for score #" << (index + 1) << ": "; cin >> highScores[index].score; cin.ignore(); } cout << endl; } void sortData(Highscore highScores[], int numItems) { for (int count = 0; count < numItems - 1; count++){ swap(highScores[findIndexOfLargest(highScores, count, numItems)], highScores[count]); } } int findIndexOfLargest(const Highscore highScores[], int startingIndex, int numItems){ int indexOfLargest = startingIndex; for (int count = startingIndex + 1; count < numItems; count++){ if (highScores[count].score > highScores[indexOfLargest].score){ indexOfLargest = count; } } return indexOfLargest; } void displayData(const Highscore highScores[], int size) { cout << "Top Scorers: " << endl; for(int index = 0; index < size; index++) { cout << highScores[index].name << ": " << highScores[index].score << endl; } }
-
Notice that the findLocationOfLargest() function does not need the vector itself as a parameter, since you can access the vector using the provided iterator parameters.
-
The name field in the struct must still be a c-string
-
The focus of this assignment is to use iterators. You must use iterators wherever possible to access the vector. As a result, you must not use square brackets, the push_back() function, the at() function, etc. Also, the word "index" shouldn't appear in your code anywhere. You won't get full credit if you miss an opportunity to use iterators.
-
You should still ask the user to enter the number of scores there will be, and then you should create a vector with the required capacity. You can do this by using the vector class's constructor that creates a vector with the capacity indicated by its parameter. For example, to create a vector of size 100, use this:
vector<sometype> myExampleVector(100);It is possible to write our program without bothering to indicate a size if we simply use push_back() to add each high score struct to the vector. We aren't doing it that way, because I want you to practice using iterators.
-
You could sort the scores by simply calling the STL sort()
algorithm . I would suggest that you try this out because it's something you should know, but for your submitted program you are required to sort the vector as it is done in the given code, except using iterators to access the items in the vector. You won't get credit for the assignment if you use the STL sort() function. -
In your displayData() function you'll need to use const_iterator instead of iterator. See lesson 19.4.
-
Here is an example that shows how to use an iterator to access a particular member of a struct:
swap((*iter1).firstmember, (*iter2).firstmember); This will work just fine, but there is a C++ operator that combines these two (dereference and then select). I works like this:swap(iter1 -> firstmember, iter2 -> firstmember);(Note that you won't actually use this example code, because when you swap, you should just swap the entire struct. There's no need to swap the individual members of the struct separately.)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

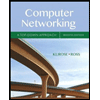
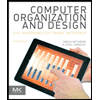
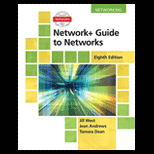
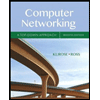
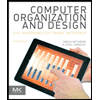
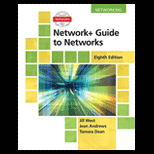
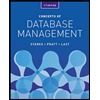
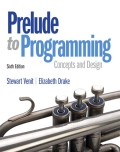
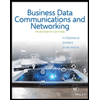