Write a menu-driven program for Food Court. Display the food menu to a user (Just show the 5 options' names and prices - No need to show the details!) Use numbers for the options and the number "6" to exit. If the user enters "6", your code should not ask any other questions, just show a message like "Thank you, hope to see you again!" Ask the user what he/she wants and how many of them. (Check the user inputs) Keep asking the user until he/she chooses the exit option. Use a data structure to save the quantities of the orders. You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data. Calculate the price. Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price. Display(print) the bill to the user. The bill includes: The food items The quantities The cost of them The total before tax Tax Total price after tax You can have your own design. The names of functions are also up to your design but the names should show what the functions do. Only display 2 decimal points when displaying all the numbers. Create a class of orders or other needed classes. Use at least one superclass and one or more subclasses and overridden methods. (For example, a Burger class can be a superclass and other types of burgers can be subclasses (the methods related to the price and the name can be overridden). The person class is also a good idea to be a superclass and Staff and Student can be the subclasses (the methods related to the tax can be overridden) Important=> Put each part and class in different Python files and import them when you want to use them (use "from" and "import" keywords). Create an object for the Order class to run the code. Print the bill on the screen Save the bill in a file. **********************************************************************************************
Write a menu-driven program for Food Court. Display the food menu to a user (Just show the 5 options' names and prices - No need to show the details!) Use numbers for the options and the number "6" to exit. If the user enters "6", your code should not ask any other questions, just show a message like "Thank you, hope to see you again!" Ask the user what he/she wants and how many of them. (Check the user inputs) Keep asking the user until he/she chooses the exit option. Use a data structure to save the quantities of the orders. You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data. Calculate the price. Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price. Display(print) the bill to the user. The bill includes: The food items The quantities The cost of them The total before tax Tax Total price after tax You can have your own design. The names of functions are also up to your design but the names should show what the functions do. Only display 2 decimal points when displaying all the numbers. Create a class of orders or other needed classes. Use at least one superclass and one or more subclasses and overridden methods. (For example, a Burger class can be a superclass and other types of burgers can be subclasses (the methods related to the price and the name can be overridden). The person class is also a good idea to be a superclass and Staff and Student can be the subclasses (the methods related to the tax can be overridden) Important=> Put each part and class in different Python files and import them when you want to use them (use "from" and "import" keywords). Create an object for the Order class to run the code. Print the bill on the screen Save the bill in a file. **********************************************************************************************
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter2: Elements Of High-quality Programs
Section: Chapter Questions
Problem 1GZ
Related questions
Question
Python help.
Write a menu-driven program for Food Court.
Display the food menu to a user (Just show the 5 options' names and prices - No need to show the details!)
Use numbers for the options and the number "6" to exit. If the user enters "6", your code should not ask any other questions, just show a message like "Thank you, hope to see you again!"
Ask the user what he/she wants and how many of them. (Check the user inputs)
Keep asking the user until he/she chooses the exit option.
Use a data structure to save the quantities of the orders. You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data.
Calculate the price.
Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price.
Display(print) the bill to the user. The bill includes:
The food items
The quantities
The cost of them
The total before tax
Tax
Total price after tax
You can have your own design. The names of functions are also up to your design but the names should show what the functions do.
Only display 2 decimal points when displaying all the numbers.
Create a class of orders or other needed classes.
Use at least one superclass and one or more subclasses and overridden methods. (For example, a Burger class can be a superclass and other types of burgers can be subclasses (the methods related to the price and the name can be overridden). The person class is also a good idea to be a superclass and Staff and Student can be the subclasses (the methods related to the tax can be overridden)
Important=> Put each part and class in different Python files and import them when you want to use them (use "from" and "import" keywords).
Create an object for the Order class to run the code.
Print the bill on the screen
Save the bill in a file.
**************************************************************************************************
Sample template:
(As I mentioned, these all are samples and you can have your own design and naming.)
# Creating an object of the class "Order"
class Order:
'''
The doc string for this class
'''
def __init__(self):
self._priceBtax = 0
self._priceAtax = 0
self._tax = 0
def displayMenu(self):
'''
The doc string for this method
'''
#This is a sample and you can have your own design
print("\n----------- Food Court -----------")
def getInputs(self):
'''
The doc string for this method
'''
#You need to fill it
# Calculate the price before and after tax
def calculate(self):
'''
The doc string for this method
'''
# Print the bill on the console
def printBill(self):
'''
The doc string for this method
'''
# Save the bill in a file
def saveToFile(self):
'''
The doc string for this method
'''
#You need to fill it
with open(orderTimeStamp,'w') as fileHandleToSaveTheBill :
#Save the bill in the file
#hint: it's like printBill but you need to use "write"
In a separate file create two objects and run the related methods:
from theFileYouSavedOrderClass import Order
if __name__ == "__main__":
flag = True
while flag:
order = Order()
order.displayMenu()
order.getInputs()
order.calculate()
order.printBill()
order.saveToFile()
userInputToContinue = input("Continue for another order(Any keys= Yes, n= No)?")
if userInputToContinue.lower() == 'n':
print("The system is shutting down!")
flag = Flase
You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data.
Use at least one subclass and one superclass.
Don't forget Doc Strings for the class and all methods
Only display 2 decimal points when displaying all the numbers.
Put at least two outputs (results after you run your code) at the end of your code as a multi-line comment.
Don't forget to put your name and a short description of your code on top of your code.
Don't forget to test your code with Positive and Negative Testing! => Your code should show an error if you enter invalid inputs.
Do not forget to submit TWO output text files (their names should be based on the format mentioned above) with your Python file here.
Submit your Python code and TWO output text files here.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
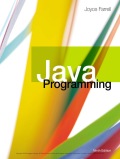
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
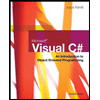
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
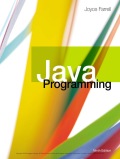
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
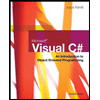
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,