. Start Eclipse and create a new Java project Lab13. 2. Download lab13.zip and extract it on your computer. 3. Import the two java files into your project Lab13 using right click src in Lab13 -> Import -> General -> File System -> next -> Browse (the CSC 1106 THE ART OF PROGRAMMING LAB 13 1directory you save IntegerList.java and IntegerListTest.java) -> open -> check IntegerList.java and IntegerListTest.java from right window -> Finish. 4. In the IntegerListTest, create a new list, print and check if it returns the correct list; 5. Add a method void replaceFirst(int oldVal, int newVal) to the IntegerList class that replaces the first occurrence of oldVal in the list with newVal. If oldVal does not appear in the list, it should do nothing (but it’s not an error). If oldVal appears more than once, only the first occurrence should be replaced; (Note: you already have a method to find oldVal in the list, so use it!) 6. Add an option to the menu in IntegerListTest to test the above new method.
These are the directions my professor gave me.
I would really appreciate any help you could give me with this practice lab, I have been stuck on it for a while...
1. Start Eclipse and create a new Java project Lab13.
2. Download lab13.zip and extract it on your computer.
3. Import the two java files into your project Lab13 using right click src in Lab13
-> Import -> General -> File System -> next -> Browse (the
CSC 1106 THE ART OF
-> open -> check IntegerList.java and IntegerListTest.java
from right window -> Finish.
4. In the IntegerListTest, create a new list, print and check if it returns the correct list;
5. Add a method void replaceFirst(int oldVal, int newVal) to the
IntegerList class that replaces the first occurrence of oldVal in the list with newVal.
If oldVal does not appear in the list, it should do nothing (but it’s not an error). If oldVal
appears more than once, only the first occurrence should be replaced; (Note: you already
have a method to find oldVal in the list, so use it!)
6. Add an option to the menu in IntegerListTest to test the above new method.
![public class IntegerList
{
int[] list; //values in the list
//-------
//create a list of the given size
//----
public IntegerList(int size)
{
list = new int[size];
}
//--
//fill array with integers between 1 and 100, inclusive
//-----
public void randomize()
{
for (int i=0; i<list.length; i++)||
list[i] = (int) (Math.random() * 100) + 1;
}
//--
//print array elements with indices
//---
public void print()
{
for (int i=0; i<list.length; i++)
System.out.println(i + ":\t" + list[i]);
{
//-
//return the index of the first occurrence of target in the list.
//return -1 if target does not appear in the list
//----
public int search(int target)
{
int location
-1;
for (int i=0; i<list.length && location
if (list[i]
location = i;
return location;
}
-1; i++)
==
target)
==
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8d1ebabc-ed8b-4a09-8d1c-406d2b940ee4%2F2def06a1-4ba9-4427-8aca-cec1e8794f83%2F5m7igh_processed.png&w=3840&q=75)
![public static void main(String[] args)
{
printMenu();
int choice = scan.nextInt();
while (choice != 0)
{
dispatch(choice);
printMenu();
choice - scan.nextInt();
}
}
//
// Do what the menu item calls for
/-
public static void dispatch(int choice)
{
int loc;
switch(choice)
{
case 0:
System.out.println("Bye!");
break;
case 1:
System.out.println("How big should the list be?");
int size = scan.nextInt();
list = new IntegerList(size);
list.randomize();
break;
case 2:
System.out.print("Enter the value to look for: ");
loc =
if (loc != -1)
System.out.println("Found at location
else
list.search(scan.nextInt());
+ loc);
System.out.println("Not in list");
break;
case 3:
list.print();
break;
default:
System.out.println("Sorry, invalid choice");
}
//-
// Print the user's choices
//-----
public static void printMenu()
{
System.out.println("\n
System.out.println("
System.out.println("0: Quit");
System.out.println("1: Create a new list (** do this first!! **)");
System.out.println("2: Find an element in the list using linear search");
System.out.println("3: Print the list");
System.out.print("\nEnter your choice: ");
}
");
====");
Menu](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8d1ebabc-ed8b-4a09-8d1c-406d2b940ee4%2F2def06a1-4ba9-4427-8aca-cec1e8794f83%2Ftbgasf7_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

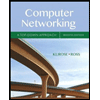
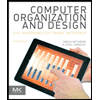
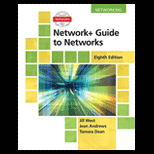
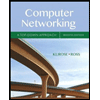
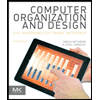
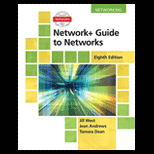
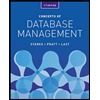
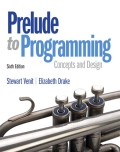
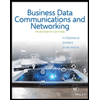