.. write a function that, given a word and a set of characters, filters the all occurrences of those characters from the word. For e.g. remove_chars("abcdeeeeefmnop", "ebo") returns: acdfmnp i.e all occurrences of e, b and o have been removed from abcdeeeeefmnop You should acquire the word and set of characters both from the user and then pass them to the function. Note: std:cin terminates when it encounters a space, you should take the word and character set as separate inputs: std:cin >> word >> charset; Where word and charset are arbitrarily named variables to store the word and character set. Hint: You can use substr(i, I) function where i is the index into the string and I is the number of characters to extract. It would be easier if you stored the output in a separate variable. Write this function recursively. Do not use any loops. Hint: You will need to create a helper function (also recursive) that checks if any given letter in the word is present in the charset. This function can be prototyped as follows: bool contains(string letter, string charset) If you do the recursion properly, you will realize your code is more concise and readable
.. write a function that, given a word and a set of characters, filters the all occurrences of those characters from the word. For e.g. remove_chars("abcdeeeeefmnop", "ebo") returns: acdfmnp i.e all occurrences of e, b and o have been removed from abcdeeeeefmnop You should acquire the word and set of characters both from the user and then pass them to the function. Note: std:cin terminates when it encounters a space, you should take the word and character set as separate inputs: std:cin >> word >> charset; Where word and charset are arbitrarily named variables to store the word and character set. Hint: You can use substr(i, I) function where i is the index into the string and I is the number of characters to extract. It would be easier if you stored the output in a separate variable. Write this function recursively. Do not use any loops. Hint: You will need to create a helper function (also recursive) that checks if any given letter in the word is present in the charset. This function can be prototyped as follows: bool contains(string letter, string charset) If you do the recursion properly, you will realize your code is more concise and readable
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
in c++.

Transcribed Image Text:write a function that, given a word and a set of characters,
filters the all occurrences of those characters from the word. For e.g.
remove_chars("abcdeeeeefmnop", "ebo")
returns: acdfmnp
i.e all occurrences of e, b and o have been removed from abcdeeeeefmnop
You should acquire the word and set of characters both from the user and then pass them to the
function.
Note: std:cin terminates when it encounters a space, you should take the word and character set
as separate inputs:
std:cin >> word > charset;
Where word and charset are arbitrarily named variables to store the word and character set.
Hint: You can use substr(i, I) function where i is the index into the string and I is the number of
characters to extract. It would be easier if you stored the output in a separate variable.
Write this function recursively. Do not use any loops.
Hint: You will need to create a helper function (also recursive) that checks if any given letter in the
word is present in the charset. This function can be prototyped as follows:
bool contains(string letter, string charset)
If you do the recursion properly, you will realize your code is more concise and readable
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
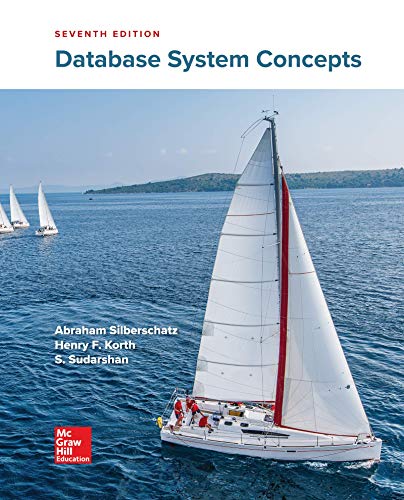
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
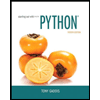
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
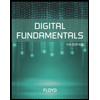
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
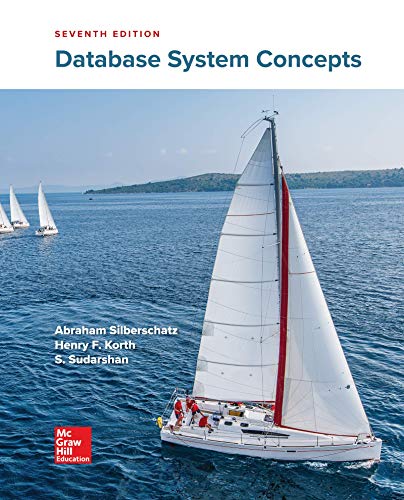
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
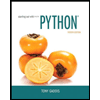
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
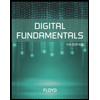
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
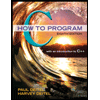
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
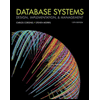
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
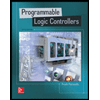
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education