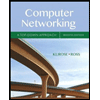
Consider an input string TAM of letters ‘A’, ‘M’, and ‘T’. This string, which
is given by the user, ends with ‘#’. It should be stored in a table (or array), called TAMUK. The
number of each of these letters is unknown. We have a function, called SWAP(TAM,i,j), which
places the i
th letter in the j
th entry of string TAM and the j
th letter in the i
th entry of TAM. Note that
SWAP(TAM,i,j) is defined for all integers i and j between 0 and length(TAM)–1, where
length(TAM) is the number of letters of TAM.
1. Using our algorithmic language, write an
letters in the array TAMUK in a way that all T’s appear first, followed by all A’s, and
followed by all M’s. The algorithm Sort_TAM should have one parameter: The array
TAMUK. Also, your solution is correct only if the following four constraints are satisfied:
- Constraint 1: Each letter (‘A’, ‘M’, or ‘T’) is evaluated only once.
- Constraint 2: The function SWAP(TAM,i,j) is used only when it is necessary.
- Constraint 3: No extra space can be used by the algorithm Sort_TAM. In other words,
only the array TAMUK can be used to sort the ‘A’, ‘M’, or ‘T’.
- Constraint 4: You cannot count the number of each letter ‘A’, ‘M’, or ‘T’.
2. Show that the algorithm Sort_TAM is correct using an informal proof (i.e., discussion).
3. Give a program corresponding to Sort_TAM using your favorite programming language.
Need code in HTML with output and detailed explanation.
The output should be with swapping not sorting (i.e, TTAAMMM not AAMMTT)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Write a python function called replacer() that takes two strings as arguments. Argument1: A sentence where you have to make the changes. Argument2: A word with the values either “vowel” or “consonant”.Your function should replace the characters of the sentence with “V” or “C” depending on the 2nd argument. If the word is “vowel”, then replace all the vowels with “V”. If the word is “consonant”, then replace all the consonants with “C”.[You are not allowed to use the replace() function]Function call1:replacer("It is MAT110 final", "vowels")Sample Output1:Vt Vs MVT110 fVnVlFunction call2:replacer("It is CSE110 final", "consonants")Sample Output 02:IC iC CCE110 CiCaCarrow_forwardWe are going to write a program to create a word puzzle. For now, what we need is a function promptletnum that will prompt the user for one letter and an integer in the range from 1 to 5 (inclusive), error-checking until the user enters valid values, and then return them. The function will convert upper case letters to lower case. This function might be called from the following script wordpuz: wordpuz.m (1, n] = promptletnum; fprintf('We will find words that contain the \n') fprintf('letter ''tc'' %d times.\n', 1, n)arrow_forwardWrite a Python function (give it any name you choose) to analyze a text string given to it. The function will receive one single string as argument It will return two tuples of two elements each: One tuple contains the word with the maximum occurrence in the input text and the number of its occurrence. The other tuple contains the longest word with in the input text and the number of characters in it. The function should be called like function1(text), where text is a string variable. Use the paragraph below to test your code: text = ‘’’During a wet autumnal walk, I was explaining to my girlfriend about my recent presentations. I've been doing my 'Getting Started with Python' talk at Aruba Airheads meet-ups. I recorded an early version of it, see below. One point I mentioned is that the reaction is always mixed. When I ask who is learning Python, about 5% of each audience put their hands up. Regularly people object to the idea of network engineers learning Python. The arguments…arrow_forward
- Write a program to compute some election results. A. Define a struct called Candidate that is able to record the name (string) and number of votes a candidates receives. B. Write a function sumVotes that accepts two arguments: an array of Candidate and the size of the array. The function should return the total number of votes received by all the candidates. This function should not do any output. C. Your main function should declare an array of ContactInfo structs of size 4, input the data into the array, and call the function to compute the total votes cast, and output this value. It should then output a table of the names of each candidate and the percentage of votes they received. The percentage can be computed by multiplying the candidates votes time 100.0 divided by the total votes. Ex: If the input is: Miller 5000 Guzman 4000 Harris 6000 Kimmel 1800 Then the output is Total 16800 Miller 29.76 Guzman 23.81 Harris 35.71 Kimmel 10.71 Do not use any prompts to get the input values.…arrow_forwardCan you help me write a C++ program to do the following: Create a function append(v, a, n) that adds to the end of vector v a copy of all the elements of array a. For example, if v contains 1, 2, 3, and a contains 4, 5, 6, then v will end up containing 1, 2, 3, 4, 5,6. The argument n is the size of the array. The argument v is a vector ofintegers and a is an array of integers. Write a test driverarrow_forwardProblem Statement: Consider an input string TAM of letters ‘A’, ‘M’, and ‘T’. This string, which is given by the user, ends with ‘#’. It should be stored in a table (or array), called TAMUK. The number of each of these letters is unknown. We have a function, called SWAP(TAM,i,j), which places the ith letter in the jth entry of string TAM and the jth letter in the ith entry of TAM. Note that SWAP(TAM,i,j) is defined for all integers i and j between 0 and length(TAM)–1, where length(TAM) is the number of letters of TAM. 1. Using our algorithmic language, write an algorithm, called Sort_TAM, which sorts the letters in the array TAMUK in a way that all T’s appear first, followed by all A’s, and followed by all M’s. The algorithm Sort_TAM should have one parameter: The array TAMUK. Also, your solution is correct only if the following four constraints are satisfied: - Constraint 1: Each letter (‘A’, ‘M’, or ‘T’) is evaluated only once. - Constraint 2: The function SWAP(TAM,i,j) is used only…arrow_forward
- Write a python function count_matches that, given two strings, counts the number of positions at which the characters are the same. For example, count_matches('conflate', 'banana') returns 2, conflate banana ^ ^ since the n's match at index 1 and the a's match at index 5. The strings to not need to be the same length. The count_matches function does not read input or print output.arrow_forwardIn c++, please! Thank you! Write a void function SelectionSortDescendTrace() that takes an integer array and sorts the array into descending order. The function should use nested loops and output the array after each iteration of the outer loop, thus outputting the array N-1 times (where N is the size). Complete main() to read in a list of up to 10 positive integers (ending in -1) and then call the SelectionSortDescendTrace() function. If the input is: 20 10 30 40 -1 then the output is: 40 10 30 20 40 30 10 20 40 30 20 10 The following code is given: #include <iostream> using namespace std; // TODO: Write a void function SelectionSortDescendTrace() that takes// an integer array and the number of elements in the array as arguments,// and sorts the array into descending order.void SelectionSortDescendTrace(int numbers [], int numElements) { } int main() { int input, i = 0; int numElements = 0; int numbers [10]; // TODO: Read in a list of up to 10 positive…arrow_forwardCreate a function append(v, a, n) that adds to the end of vector v a copy of all the elements of array a. For example, if v contains 1,2, 3, and a contains 4, 5, 6, then v will end up containing 1, 2, 3, 4, 5,6. The argument n is the size of the array. The argument v is a vector ofintegers and a is an array of integers. Write a test driver.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
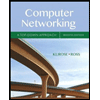
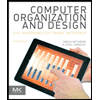
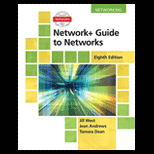
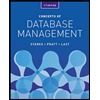
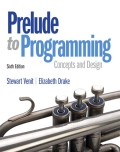
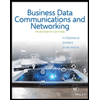