... #include #include #include /* * Function to check whether two passed strings are anagram or not */ int isAnagram(char *firstString, char *secondString) { int firstCharCounter[256] = {0}, secondCharCounter[256] = {0}; int counter; // Two Strings cannot be anagram if their length is not equal if(strlen(firstString) != strlen(secondString)) return 0; // count frequency of characters of firstString for(counter = 0; firstString[counter] != '\0'; counter++) { firstCharCounter[firstString[counter]]++; } // count frequency of characters of secondString for(counter = 0; secondString[counter] != '\0'; counter++) { secondCharCounter[secondString[counter]]++; } // compare character counts of both strings, // If not equal return 0, otherwise 1 for (counter = 0; counter < 256; counter++) { if (firstCharCounter[counter] != secondCharCounter[counter]) return 0; } return 1; } int main() { char firstString[100], secondArray[100]; printf("First string? "); gets(firstString); printf("Second string? "); gets(secondArray); if(isAnagram(firstString, secondArray) == 1) printf("%s and %s are Anagrams\n",firstString,secondArray); else printf("%s and %s are not Anagrams\n",firstString,secondArray); getch(); return 0; }
... #include #include #include /* * Function to check whether two passed strings are anagram or not */ int isAnagram(char *firstString, char *secondString) { int firstCharCounter[256] = {0}, secondCharCounter[256] = {0}; int counter; // Two Strings cannot be anagram if their length is not equal if(strlen(firstString) != strlen(secondString)) return 0; // count frequency of characters of firstString for(counter = 0; firstString[counter] != '\0'; counter++) { firstCharCounter[firstString[counter]]++; } // count frequency of characters of secondString for(counter = 0; secondString[counter] != '\0'; counter++) { secondCharCounter[secondString[counter]]++; } // compare character counts of both strings, // If not equal return 0, otherwise 1 for (counter = 0; counter < 256; counter++) { if (firstCharCounter[counter] != secondCharCounter[counter]) return 0; } return 1; } int main() { char firstString[100], secondArray[100]; printf("First string? "); gets(firstString); printf("Second string? "); gets(secondArray); if(isAnagram(firstString, secondArray) == 1) printf("%s and %s are Anagrams\n",firstString,secondArray); else printf("%s and %s are not Anagrams\n",firstString,secondArray); getch(); return 0; }
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.4: A Case Study: Rectangular To Polar Coordinate Conversion
Problem 9E: (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question
PLease help me to add a loop that will let program repeat until the user will type 'exit' C language
...
#include <stdio.h>
#include <conio.h>
#include <string.h>
/*
* Function to check whether two passed strings are anagram or not
*/
int isAnagram(char *firstString, char *secondString)
{
int firstCharCounter[256] = {0}, secondCharCounter[256] = {0};
int counter;
// Two Strings cannot be anagram if their length is not equal
if(strlen(firstString) != strlen(secondString))
return 0;
// count frequency of characters of firstString
for(counter = 0; firstString[counter] != '\0'; counter++)
{
firstCharCounter[firstString[counter]]++;
}
// count frequency of characters of secondString
for(counter = 0; secondString[counter] != '\0'; counter++)
{
secondCharCounter[secondString[counter]]++;
}
// compare character counts of both strings,
// If not equal return 0, otherwise 1
for (counter = 0; counter < 256; counter++)
{
if (firstCharCounter[counter] != secondCharCounter[counter])
return 0;
}
return 1;
}
int main()
{
char firstString[100], secondArray[100];
printf("First string? ");
gets(firstString);
printf("Second string? ");
gets(secondArray);
if(isAnagram(firstString, secondArray) == 1)
printf("%s and %s are Anagrams\n",firstString,secondArray);
else
printf("%s and %s are not Anagrams\n",firstString,secondArray);
getch();
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
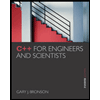
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
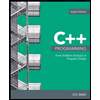
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
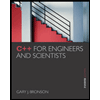
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
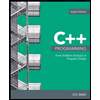
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning