Remove error from following cpp program: CODE: #include #include using namespace std; class student { public: string name[100]; int rollno[100], oop[100], dld[100], psychology[100], pie[100], n; void get() { cout << "Enter the no. of students whose data do you want to enter:"; cin >> n; for (int i = 0; i < n; i++) { cout << "Enter student's #" << i + 1 << " name:"; cin >> name[i]; cout << "Enter student's #" << i + 1 << " roll no."; cin >> rollno[i]; cout << "Enter student's #" << i + 1 << " OOP marks:"; cin >> oop[i]; cout << "Enter student's #" << i + 1 << " DLD marks:"; cin >> dld[i]; cout << "Enter student's #" << i + 1 << " psychology marks:"; cin >> psychology[i]; cout << "Enter student's #" << i + 1 << " pie marks:"; cin >> pie[i]; } }; class modify :public student { public: student student st; void change() { int roll; cout << "Enter student roll no. whose record do you want to modify:"; cin >> roll; for (int i = 0; i < n; i++) { if (roll == st.rollno[i]) { cout << "Enter student's new name:"; cin >> st.name[i]; cout << "Enter student's new roll no."; cin >> st.rollno[i]; cout << "Enter student's new OOP marks:"; cin >> st.oop[i]; cout << "Enter student's new DLD marks:"; cin >> st.dld[i]; cout << "Enter student's new psychology marks:"; cin >> st.psychology[i]; cout << "Enter student's new pie marks:"; cin >> st.pie[i]; } } } }; class search::public student { public: student st; void searchrec() { int roll; cout << "Enter student roll no. whose record do you want to see:"; cin >> roll; for (int i = 0; i < n; i++) { if (roll == st.rollno[i]) { cout << "Student's #" << i + 1 << " name is: " << st.name[i]; cout << "Student's #" << i + 1 << " roll no. is: " << st.rolllno[i]; cout << "Student's #" << i + 1 << " OOP marks are: " << st.oop[i]; cout << "Student's #" << i + 1 << " DLD marks are: " << st.dld[i]; cout << "Student's #" << i + 1 << " psychology marks are: " << st.psychology[i]; cout << "Student's #" << i + 1 << " pie marks: " << st.pie[i]; } } } }; class grades::public student { public: void gradecal(int n) { string ch; if (n <= 100 && n >= 95) { ch = "A+"; } else if (n <= 95 && n >= 90) { ch = "A"; } else if (n <= 90 && n >= 85) { ch = "A-"; } else if (n <= 85 && n >= 80) { ch = "B+"; } else if (n <= 80 && n >= 75) { ch = "B"; } else if (n <= 75 && n >= 70) { ch = "B-"; } else if (n <= 70 && n >= 65) { ch = "C+"; } else if (n <= 65 && n >= 60) { ch = "C"; } else if (n <= 60 && n >= 55) { ch = "C-"; } else if (n <= 55 && n >= 50) { ch = "D+"; } else if (n <= 50 && n >= 45) { ch = "D"; } else if (n <= 45 && n >= 40) { ch = "D-"; } else if (n < 40) { ch = "F"; } return ch; void show() { for (int i = 0; i < n; i++) { cout << "Student's #" << i + 1 << " name is: " << name[i] << endl; cout << "Student's #" << i + 1 << " roll no. is: " << rolllno[i] << endl; cout << "Student's #" << i + 1 << " OOP marks are: " << oop[i] << endl; cout << "Student's #" << i + 1 << " DLD marks are: " << dld[i] << endl; cout << "Student's #" << i + 1 << " psychology marks are: " << psychology[i] << endl; cout << "Student's #" << i + 1 << " pie marks: " << pie[i] << endl; cout << "Student #" << i + 1 << " grade in OOP is: " << gradecal(st.oop[i]) << endl; cout << "Student #" << i + 1 << " grade in OOP is: " << gradecal(st.dld[i]) << endl; cout << "Student #" << i + 1 << " grade in OOP is: " << gradecal(st.psychology[i]) << endl; cout << "Student #" << i + 1 << " grade in OOP is: " << gradecal(st.pie[i]) << endl; } } }; int main() { student stu; modify mod; search sea; grades grad; char ch, n; do { cout << "1. Enter new record" << endl; cout << "2. Modify existing record" << endl; cout << "3. Search any record" << endl; cout << "4. Show all records" << endl; cout << "Enter option do you want to select(1-4):"; cin >> ch; if (ch == '1') { stu.get(); } if (ch == '2') { mod.change(); } if (ch == '3') { sea.searchrec(); } if (ch == '4') { grad.show(); } cout << "Do you want to perform the operation again then press y else n:"; cin >> n; } while (n == 'y' || n == 'Y'); }
Remove error from following cpp program:
CODE:
#include<iostream>
#include <iomanip>
using namespace std;
class student
{
public:
string name[100];
int rollno[100], oop[100], dld[100], psychology[100], pie[100], n;
void get() {
cout << "Enter the no. of students whose data do you want to enter:";
cin >> n;
for (int i = 0; i < n; i++)
{
cout << "Enter student's #" << i + 1 << " name:";
cin >> name[i];
cout << "Enter student's #" << i + 1 << " roll no.";
cin >> rollno[i];
cout << "Enter student's #" << i + 1 << " OOP marks:";
cin >> oop[i];
cout << "Enter student's #" << i + 1 << " DLD marks:";
cin >> dld[i];
cout << "Enter student's #" << i + 1 << " psychology marks:";
cin >> psychology[i];
cout << "Enter student's #" << i + 1 << " pie marks:";
cin >> pie[i];
}
};
class modify :public student
{
public: student
student st;
void change() {
int roll;
cout << "Enter student roll no. whose record do you want to modify:";
cin >> roll;
for (int i = 0; i < n; i++)
{
if (roll == st.rollno[i])
{
cout << "Enter student's new name:";
cin >> st.name[i];
cout << "Enter student's new roll no.";
cin >> st.rollno[i];
cout << "Enter student's new OOP marks:";
cin >> st.oop[i];
cout << "Enter student's new DLD marks:";
cin >> st.dld[i];
cout << "Enter student's new psychology marks:";
cin >> st.psychology[i];
cout << "Enter student's new pie marks:";
cin >> st.pie[i];
}
}
}
};
class search::public student
{
public:
student st;
void searchrec() {
int roll;
cout << "Enter student roll no. whose record do you want to see:";
cin >> roll;
for (int i = 0; i < n; i++)
{
if (roll == st.rollno[i])
{
cout << "Student's #" << i + 1 << " name is: " << st.name[i];
cout << "Student's #" << i + 1 << " roll no. is: " << st.rolllno[i];
cout << "Student's #" << i + 1 << " OOP marks are: " << st.oop[i];
cout << "Student's #" << i + 1 << " DLD marks are: " << st.dld[i];
cout << "Student's #" << i + 1 << " psychology marks are: " << st.psychology[i];
cout << "Student's #" << i + 1 << " pie marks: " << st.pie[i];
}
}
}
};
class grades::public student
{
public:
void gradecal(int n)
{
string ch;
if (n <= 100 && n >= 95)
{
ch = "A+";
}
else if (n <= 95 && n >= 90)
{
ch = "A";
}
else if (n <= 90 && n >= 85)
{
ch = "A-";
}
else if (n <= 85 && n >= 80)
{
ch = "B+";
}
else if (n <= 80 && n >= 75)
{
ch = "B";
}
else if (n <= 75 && n >= 70)
{
ch = "B-";
}
else if (n <= 70 && n >= 65)
{
ch = "C+";
}
else if (n <= 65 && n >= 60)
{
ch = "C";
}
else if (n <= 60 && n >= 55)
{
ch = "C-";
}
else if (n <= 55 && n >= 50)
{
ch = "D+";
}
else if (n <= 50 && n >= 45)
{
ch = "D";
}
else if (n <= 45 && n >= 40)
{
ch = "D-";
}
else if (n < 40)
{
ch = "F";
}
return ch;
void show() {
for (int i = 0; i < n; i++)
{
cout << "Student's #" << i + 1 << " name is: " << name[i] << endl;
cout << "Student's #" << i + 1 << " roll no. is: " << rolllno[i] << endl;
cout << "Student's #" << i + 1 << " OOP marks are: " << oop[i] << endl;
cout << "Student's #" << i + 1 << " DLD marks are: " << dld[i] << endl;
cout << "Student's #" << i + 1 << " psychology marks are: " << psychology[i] << endl;
cout << "Student's #" << i + 1 << " pie marks: " << pie[i] << endl;
cout << "Student #" << i + 1 << " grade in OOP is: " << gradecal(st.oop[i]) << endl;
cout << "Student #" << i + 1 << " grade in OOP is: " << gradecal(st.dld[i]) << endl;
cout << "Student #" << i + 1 << " grade in OOP is: " << gradecal(st.psychology[i]) << endl;
cout << "Student #" << i + 1 << " grade in OOP is: " << gradecal(st.pie[i]) << endl;
}
}
};
int main()
{
student stu;
modify mod;
search sea;
grades grad;
char ch, n;
do
{
cout << "1. Enter new record" << endl;
cout << "2. Modify existing record" << endl;
cout << "3. Search any record" << endl;
cout << "4. Show all records" << endl;
cout << "Enter option do you want to select(1-4):";
cin >> ch;
if (ch == '1')
{
stu.get();
}
if (ch == '2')
{
mod.change();
}
if (ch == '3')
{
sea.searchrec();
}
if (ch == '4')
{
grad.show();
}
cout << "Do you want to perform the operation again then press y else n:";
cin >> n;
} while (n == 'y' || n == 'Y');
}

Step by step
Solved in 3 steps with 3 images

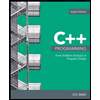
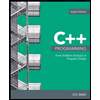