.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import javafx.event.*;
import javafx.scene.text.*;
public class SweepstakesJavaFX extends Application
{
private TextField first = new TextField();
private TextField last = new TextField();
private TextField phone = new TextField();
private TextField email = new TextField();
private TextField luckyNum = new TextField();
private TextField dob = new TextField();
public Label error = new Label();
public Label title = new Label("Sweepstakes Entry Form\nPlease complete the fields below");
public static void main(String[] args)
{
Application.launch(args);
}
@Override
public void start(Stage primaryStage)
{
VBox
.scene.control.*; import javafx.scene.layout.*; import javafx.stage.Stage; import javafx.event.*; import javafx.scene.text.*; public class SweepstakesJavaFX extends Application { private TextField first = new TextField(); private TextField last = new TextField(); private TextField phone = new TextField(); private TextField email = new TextField(); private TextField luckyNum = new TextField(); private TextField dob = new TextField(); public Label error = new Label(); public Label title = new Label("Sweepstakes Entry Form\nPlease complete the fields below"); public static void main(String[] args) { Application.launch(args); } @Override public void start(Stage primaryStage) { VBox
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter8: Arrays
Section: Chapter Questions
Problem 3E
Related questions
Question
import javafx.application.Application;
import javafx.geometry.*;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import javafx.event.*;
import javafx.scene.text.*;
public class SweepstakesJavaFX extends Application
{
private TextField first = new TextField();
private TextField last = new TextField();
private TextField phone = new TextField();
private TextField email = new TextField();
private TextField luckyNum = new TextField();
private TextField dob = new TextField();
public Label error = new Label();
public Label title = new Label("Sweepstakes Entry Form\nPlease complete the fields below");
public static void main(String[] args)
{
Application.launch(args);
}
@Override
public void start(Stage primaryStage)
{
VBox labels = new VBox();
GridPane pane = new GridPane();
pane.setAlignment(Pos.CENTER);
labels.setAlignment(Pos.CENTER);
pane.setPadding(new Insets(10, 5, 5, 5));
labels.setPadding(new Insets(5, 5, 5, 5));
pane.setHgap(45.5);
pane.setVgap(20.5);
title.setStyle("-fx-font-weight: bold; -fx-font-size:20;");
error.setStyle("-fx-text-fill: red;");
labels.getChildren().add(title);
labels.getChildren().add(error);
pane.add(new Label("First Name: "), 0, 0);
pane.add(first, 1, 0);
pane.add(new Label("Last Name: "), 0, 1);
pane.add(last, 1, 1);
pane.add(new Label("Phone Number: "), 0, 2);
pane.add(phone, 1, 2);
pane.add(new Label("Email Address: "), 0, 3);
pane.add(email, 1, 3);
pane.add(new Label("Lucky Number: "), 0, 4);
pane.add(luckyNum, 1, 4);
pane.add(new Label("Date of Birth: "), 0, 5);
pane.add(dob, 1, 5);
Button btnSubmit = new Button("Submit");
pane.add(btnSubmit,1,6);
GridPane.setHalignment(btnSubmit, HPos.RIGHT);
Scene scene = new Scene(new VBox(labels,pane), 450, 525);
primaryStage.setTitle("Sign up form"); // Set the stage title
primaryStage.setScene(scene); // Place the scene in the stage
primaryStage.show(); // Display the stage
btnSubmit.setOnAction(new EventHandler()
{
@Override
public void handle(ActionEvent e)
{
String fName = first.getText();
String lName = last.getText();
String inPhone = phone.getText();
String inEmail = email.getText();
String inLuckyNum = luckyNum.getText();
String inDob = dob.getText();
error.setText("");
// Call the validation methods here.
if (error.getText() == "")
{
primaryStage.hide();
resultsPage();
}
}
});
}
public void checkFirst(String first)
{
}
public void checkLast(String last)
{
}
public void checkPhone(String phone)
{
}
public void checkEmail(String email)
{
}
public void checkLuckyNum(String luckyNum)
{
}
public void checkDob(String dob)
{
}
public void resultsPage()
{
Stage resultsStage = new Stage();
VBox results = new VBox();
results.setAlignment(Pos.CENTER);
results.setPadding(new Insets(10, 5, 5, 5));
Label congrats = new Label("Congrats!");
Label display = new Label("The fields have been validated!");
congrats.setStyle("-fx-font-weight: bold; -fx-font-size:20; -fx-text-fill:blue;");
display.setStyle("-fx-font-weight: bold; -fx-font-size:20; -fx-text-fill:blue;");
results.getChildren().add(congrats);
results.getChildren().add(display);
Scene scene = new Scene(results, 400, 400);
resultsStage.setTitle("Results Page");
resultsStage.setScene(scene);
resultsStage.show();
}
}

Transcribed Image Text:Validation requirements
Every field is required
First Name and Last Name: at least 2 characters, and only contains
uppercase and lowercase letters
Phone Number: Format is ###-###-####, where #'s can only be digits
Email Address: Format is xxx@xxx.yyy, where xxx and yyy stand in for
any number of characters (at least one). yyy's can be any letter or digit.
xxx's can be any letter, digit, or special characters:. _ or -
Lucky Number: Can be any number between 1 and 100.
Date of Birth: Format is mm/dd/yyyy or m/d/yyyy, where m, d, and y's
can only be digits. The first two values for yy must be either 19 or 20.
question
1
For each of the six form fields, draft a regular expression that you think will work for each field-try
to write them out by hand first! The six fields are:
First Name, Last Name, Phone Number, Email Address, Lucky Number, Date of Birth
One at a time, test each regular expression - it is very important to test one regex at a time, because it
is much easier to troubleshoot! For each regex that you test, follow these steps:
• Add one pattern attribute to an input tag and use your regular expression.
•
Save your work and refresh the page.
Test your regex by trying to input both valid and invalid data.
Great work! You were able to create a functioning HTML form with validation! Now, you need to
create this same form in Java. You have been given the JavaFX code for the layout; you will just need
to write the code that does the validation.
As you saw in the previous research lab, JavaFX is a set of packages which creates event-driven
applications. Applications are laid out differently than the types of programs we have been writing so
far. Look at the main method - there is only one line, which calls the Application. launch method.
This method then calls the start method, which is directly below main. Read through the beginning of
this method (lines 28-65), to get a general sense of what is happening in this section of code.
Unlike in HTML, Java does not have attributes to accomplish data validation. Instead, you need to
create validation methods, utilizing the String methods.
Since there can be multiple errors on a form, you will need to accumulate your error messages. In other
words, if the form has more than one error, it should show ALL of the error messages. In this program,
what type of object is error? Look up this class in the JavaFX 8 documentation. What method can
retrieve the current value stored in error? What method allows you to update the value in error?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
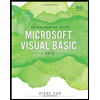
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
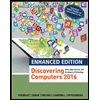
Enhanced Discovering Computers 2017 (Shelly Cashm…
Computer Science
ISBN:
9781305657458
Author:
Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Publisher:
Cengage Learning
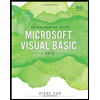
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
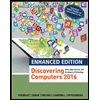
Enhanced Discovering Computers 2017 (Shelly Cashm…
Computer Science
ISBN:
9781305657458
Author:
Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Publisher:
Cengage Learning