1) BuildaMaxHeap 1) A MaxHeap interface will be provided. Based on the interface complete the MaxHeap implementation Confirm your MaxHeap works as expected by running it through the MaxHeap test harness Part 3 Pay Bus Fare - $1.80 (Using your Max Heap) A BusFareHandler interface will be provided Implement your BusFareHandler implementation. Confirm your BusFareHandler works as expected by running it through the test harness • Note: i) BusFareHandlerhas-a ii) Comments on methods: (1) grabChange(): Load your maxheap with random coins – nickel thru silver dollar (2) getMaxFromHeap(): use your MaxHeap instance to get the largest coin (3) payBusFare(int): (recursive method) retrieves the next coin via (a) maxHeap.extractMax() from your maxheap instance until you come up with exactly $1.80. BusFareHandler.java package BusFare; public interface BusFareHandler { /** * Initializes your available change. * * * create a MaxHeap with a 1,5,10,25,50,100 coin randomly inserted */ public void grabChange(); /** * get the largest ( in this case the 100 cent ) coin from your maxHeap * * @return max */ public int getMaxFromHeap(); /** * (1) The param for this method should be the result returned from getMaxFromHeap() * * (2) RECURSIVSELY build up the bus fare. * * * (3) Constraint: Bus only accepts 5,25,50,100 cent coins, but you should have other coins in your collection. * * * @param coin */ public int payBusFare(int coin); int getChange(); //week1 test recursive call by selecting next element out of a sorted array instead of calling getMaxFromHeap() } BusFareHandlerImpl.java package BusFare; import java.util.Stack; import MaxHeap.*; public class BusFareHandlerImpl implements BusFareHandler { private Stack changeStack; private MaxHeap coinHeap; private int change; public BusFareHandlerImpl() { this.coinHeap = new MaxHeapImpl(10); this.change = 0; } @Override public void grabChange() { coinHeap = new MaxHeapImpl(15); coinHeap.insert(50); coinHeap.insert(1); coinHeap.insert(25); coinHeap.insert(10); coinHeap.insert(100); coinHeap.insert(5); changeStack = new Stack<>(); } @Override public int getMaxFromHeap() { return this.coinHeap.extractMax(); } @Override public int payBusFare(int amount) { if (amount == 180) { System.out.println("Payment complete."); return amount; } int coin = this.getMaxFromHeap(); if (amount + coin <= 180) { amount += coin; System.out.println("Paid " + coin + " cents. Current total: " + amount); this.payBusFare(amount); } else { System.out.println("Cannot pay with current coins. Need to get change."); this.change = amount + coin - 180; this.payBusFare(180); } return coin; } @Override public int getChange() { int totalChange = 0; while (!changeStack.isEmpty()) { totalChange += changeStack.pop(); } return this.change; } } BusFareTester.java package BusFare; public class BusFareTester { public static void main(String[] args) { BusFareHandler fareHandler = new BusFareHandlerImpl(); fareHandler.grabChange(); System.out.println("Coins in heap:"); fareHandler.coinHeap.display(); System.out.println(); fareHandler.payBusFare(0); System.out.println("Change: " + fareHandler.getChange()); } } Rider.java package BusRider; public interface Rider { /* b. Your task is to: Implement the Rider Interface such that: Your implementation contains private members including: String name Int id // 4 digit code Your implementation overrides the hashcode() and equals() methods: // Hashcode: id %10 //equals – compare name & id 2. Test Harness: a. Create 6 riders – i. 2 with unique id’s & names ii. 2 with different name but same id’s iii. 2 with same name & id b. Demonstrate equality and collision by running your riders thru the test harness & displaying hashcode/equals results for each */ boolean equals(Object object); //provide a simple hash code public int hashCode(); //accessors & mutators public String getName(); public String getType(); public String toString(); } RiderImpl.java package BusRider; public class RiderImpl implements Rider { public String name; private String type = "student"; private int id; public RiderImpl (String name, int id) { this.name = name; this.id = id; } public String getName() { return name; } public String getType() { return type; } public String toString() { return "Rider's Name: "+name+", Type: "+type+ ",ID: " + id; } public boolean equals(Object obj) { if(obj == this) return true; if(!(obj instanceof RiderImpl)) { return false; } RiderImpl other = (RiderImpl) obj; return this.name.equals(other.name) && this.id == other.id; } //provide a simple hash code public int hashCode() { return id%10; } } RiderTester.java MaxHeap.java MaxHeapImpl.java MaxHeapTester.java
1) BuildaMaxHeap
1) A MaxHeap interface will be provided.
- Based on the interface complete the MaxHeap implementation
- Confirm your MaxHeap works as expected by running it through the
MaxHeap test harness
Part 3
Pay Bus Fare - $1.80 (Using your Max Heap)
A BusFareHandler interface will be provided
- Implement your BusFareHandler implementation.
- Confirm your BusFareHandler works as expected by running it through the
test harness • Note:
- i) BusFareHandlerhas-a ii) Comments on methods:
(1) grabChange(): Load your maxheap with random coins – nickel thru silver dollar
(2) getMaxFromHeap(): use your MaxHeap instance to get the largest coin
(3) payBusFare(int): (recursive method) retrieves the next coin via
(a) maxHeap.extractMax() from your maxheap instance until you come up with exactly $1.80.
BusFareHandler.java
package BusFare;
public interface BusFareHandler {
/**
* Initializes your available change.
*
*
* create a MaxHeap with a 1,5,10,25,50,100 coin randomly inserted
*/
public void grabChange();
/**
* get the largest ( in this case the 100 cent ) coin from your maxHeap
*
* @return max
*/
public int getMaxFromHeap();
/**
* (1) The param for this method should be the result returned from getMaxFromHeap()
*
* (2) RECURSIVSELY build up the bus fare.
*
*
* (3) Constraint: Bus only accepts 5,25,50,100 cent coins, but you should have other coins in your collection.
*
*
* @param coin
*/
public int payBusFare(int coin);
int getChange();
//week1 test recursive call by selecting next element out of a sorted array instead of calling getMaxFromHeap()
}
BusFareHandlerImpl.java
package BusFare;
import java.util.Stack;
import MaxHeap.*;
public class BusFareHandlerImpl implements BusFareHandler {
private Stack<Integer> changeStack;
private MaxHeap coinHeap;
private int change;
public BusFareHandlerImpl() {
this.coinHeap = new MaxHeapImpl(10);
this.change = 0;
}
@Override
public void grabChange() {
coinHeap = new MaxHeapImpl(15);
coinHeap.insert(50);
coinHeap.insert(1);
coinHeap.insert(25);
coinHeap.insert(10);
coinHeap.insert(100);
coinHeap.insert(5);
changeStack = new Stack<>();
}
@Override
public int getMaxFromHeap() {
return this.coinHeap.extractMax();
}
@Override
public int payBusFare(int amount) {
if (amount == 180) {
System.out.println("Payment complete.");
return amount;
}
int coin = this.getMaxFromHeap();
if (amount + coin <= 180) {
amount += coin;
System.out.println("Paid " + coin + " cents. Current total: " + amount);
this.payBusFare(amount);
} else {
System.out.println("Cannot pay with current coins. Need to get change.");
this.change = amount + coin - 180;
this.payBusFare(180);
}
return coin;
}
@Override
public int getChange() {
int totalChange = 0;
while (!changeStack.isEmpty()) {
totalChange += changeStack.pop();
}
return this.change;
}
}
BusFareTester.java
package BusFare;
public class BusFareTester {
public static void main(String[] args) {
BusFareHandler fareHandler = new BusFareHandlerImpl();
fareHandler.grabChange();
System.out.println("Coins in heap:");
fareHandler.coinHeap.display();
System.out.println();
fareHandler.payBusFare(0);
System.out.println("Change: " + fareHandler.getChange());
}
}
Rider.java
package BusRider;
public interface Rider {
/*
b. Your task is to:
Implement the Rider Interface such that:
Your implementation contains private members including:
String name
Int id // 4 digit code
Your implementation overrides the hashcode() and equals() methods:
// Hashcode: id %10
//equals – compare name & id
2. Test Harness:
a. Create 6 riders –
i. 2 with unique id’s & names
ii. 2 with different name but same id’s
iii. 2 with same name & id
b. Demonstrate equality and collision by running your riders thru the test harness & displaying hashcode/equals results for each
*/
boolean equals(Object object);
//provide a simple hash code
public int hashCode();
//accessors & mutators
public String getName();
public String getType();
public String toString();
}
RiderImpl.java
package BusRider;
public class RiderImpl implements Rider
{
public String name;
private String type = "student";
private int id;
public RiderImpl (String name, int id) {
this.name = name;
this.id = id;
}
public String getName()
{
return name;
}
public String getType() {
return type;
}
public String toString()
{
return "Rider's Name: "+name+", Type: "+type+ ",ID: " + id;
}
public boolean equals(Object obj)
{
if(obj == this)
return true;
if(!(obj instanceof RiderImpl))
{
return false;
}
RiderImpl other = (RiderImpl) obj;
return this.name.equals(other.name) && this.id == other.id; }
//provide a simple hash code
public int hashCode()
{
return id%10;
}
}
RiderTester.java
MaxHeap.java
MaxHeapImpl.java
MaxHeapTester.java

Step by step
Solved in 5 steps

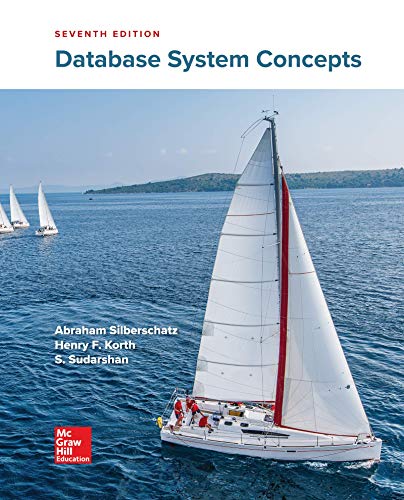
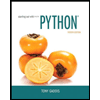
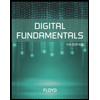
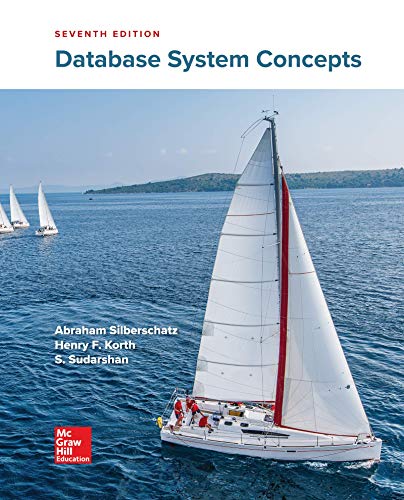
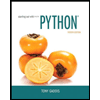
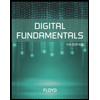
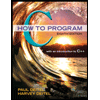
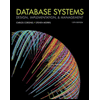
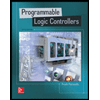