(1) Create two files. SongEntry.java - Class declaration Playlist.java - Contains main() method Build the SongEntry class per the following specifications. Note: Some methods can initially be method stubs (empty methods), to be completed in later steps. Private fields String uniqueID - Initialized to "none" in the default constructor string songName - Initialized to "none" in default constructor string artistName - Initialized to "none" in default constructor int songLength - Initialized to 0 in default constructor SongEntry nextNode - Initialized to null in default constructor Default constructor Parameterized constructor Public member methods void insertAfter(SongEntry currNode) void setNext(SongEntry nextNode) - Mutator String getID()- Accessor String getSongName() - Accessor String getArtistName() - Accessor int getSongLength() - Accessor SongEntry getNext() - Accessor void printPlaylistSongs() Ex. of printPlaylistSongs output: Unique ID: S123 Song Name: Peg Artist Name: Steely Dan Song Length (in seconds): 237 (2) In main(), prompt the user for the title of the playlist. Ex: Enter playlist's title: (3) Implement the printMenu() method. printMenu() takes the playlist title as a parameter and outputs a menu of options to manipulate the playlist.
(1) Create two files.
- SongEntry.java - Class declaration
- Playlist.java - Contains main() method
Build the SongEntry class per the following specifications. Note: Some methods can initially be method stubs (empty methods), to be completed in later steps.
- Private fields
- String uniqueID - Initialized to "none" in the default constructor
- string songName - Initialized to "none" in default constructor
- string artistName - Initialized to "none" in default constructor
- int songLength - Initialized to 0 in default constructor
- SongEntry nextNode - Initialized to null in default constructor
- Default constructor
- Parameterized constructor
- Public member methods
- void insertAfter(SongEntry currNode)
- void setNext(SongEntry nextNode) - Mutator
- String getID()- Accessor
- String getSongName() - Accessor
- String getArtistName() - Accessor
- int getSongLength() - Accessor
- SongEntry getNext() - Accessor
- void printPlaylistSongs()
Ex. of printPlaylistSongs output:
Unique ID: S123 Song Name: Peg Artist Name: Steely Dan Song Length (in seconds): 237
(2) In main(), prompt the user for the title of the playlist.
Ex:
Enter playlist's title:
(3) Implement the printMenu() method. printMenu() takes the playlist title as a parameter and outputs a menu of options to manipulate the playlist.
(4) Implement the executeMenu() method that takes 4 parameters: a character representing the user's choice, a playlist title, the head node of a playlist, and a Scanner object. executeMenu() performs the menu options (described below) according to the user's choice, and returns the head node of the playlist.
(5) In main(), call printMenu() and prompt for the user's choice of menu options. Each option is represented by a single character.
If an invalid character is entered, continue to prompt for a valid choice. When a valid option is entered, execute the option by calling executeMenu() and overwrite the head of the playlist with the returned object. Then, print the menu, and prompt for a new option. Continue until the user enters 'q'. Hint: Implement Quit before implementing other options.
(6) Implement "Output full playlist" menu option in executeMenu(). If the list is empty, output: Playlist is empty
(7) Implement the "Add song" menu option in executeMenu(). New additions are added to the end of the list.
(8) Implement the "Remove song" menu option in executeMenu(). Prompt the user for the unique ID of the song to be removed.
(9) Implement the "Change position of song" menu option in executeMenu(). Prompt the user for the current position of the song and the desired new position. Valid new positions are 1 - n (the number of nodes). If the user enters a new position that is less than 1, move the node to the position 1 (the head). If the user enters a new position greater than n, move the node to position n (the tail). 6 cases will be tested:
- Moving the head node
- Moving the tail node
- Moving a node to the head
- Moving a node to the tail
- Moving a node up the list
- Moving a node down the list
(10) Implement the "Output songs by specific artist" menu option in executeMenu(). Prompt the user for the artist's name, and output the node's information, starting with the node's current position.
(11) Implement the "Output total time of playlist" menu option in executeMenu(). Output the sum of the time of the playlist's songs (in seconds).

![1 import java.util.Scanner;
2 public class Playlist {
3 public static void printMenu(String playlistTitle) {
4
/* Type code here. */
5
6
7
8
9
HELL
10 public static void main(String[] args) {
11
Scanner scnr = new Scanner(System.in);
/* Type code here. */
12
13
m & in
14
Current file: Playlist.java
15 }
}
public static Song Entry executeMenu(char option, String playlistTitle, SongEntry headNode, Scanner scnr) {
/* Type code here. */
return headNode;
}
Load default template](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9e6fa127-a003-469d-9a51-b1d4610918ed%2Fe9d10382-2c39-4337-bf67-7ed3b96c027b%2Fz4xvorc_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

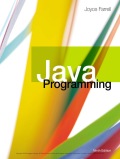
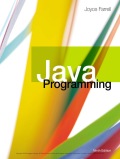