1- Implement the class "cylinder" with member variables radius and height, which are private of type double. Define the global constant PI=3.1415 and use it in calculating the volume of the cylinder (PI*radius*radius*height). Implement in the class cylinder the following functions: a- A default constructor with default values of one. b- One constructor with two arguments. This constructor should check that the variable is positive and does not exceed 20, otherwise the variable will be assigned its default value of one. c- A reader for each variable. d- A writer for each variable. e- A reader and a writer for diameter. f- A member function “volume" which calculates the volume of a cylinder. g- A member function “print" which prints the radius, diameter, and height of a cylinder. h- Á member function display_name() which displays “cylinder". 2- Write a program which declares an array of n cylinders (use n=5). Initialize the array values by writing a loop which asks the user to input the radius and the height of each array element. 3- Add to the class cylinder a private static variable which is used to count the number of cylinders generated. For this purpose, you need to perform the following: a- Declare and initialize the variable and add a reader to read this variable. b- Modify the constructors as needed and add a destructor. c- Add also a copy constructor which uses a call by reference. 4- Declare an array of n pointers to cylinders (use n=5) and initialize it to the addresses of the array of question 2. Sort this array of pointers to facilitate access to cylinders in ascending order of height. 5- Write a program that implements the equivalent functionality of strlen. This function takes a pointer to a char and determines the length of the array.
c++
i have the solution for first 2 questions, please solve as much as you can(no problem the time)
qusetion1:
#include <iostream>
using namespace std;
// Global constant variable
const int PI = 3.1415;
// Cylinder class definition
class cylinder{
// Private member variables
private:
double radius,height,diameter;
public:
// Default constructor
cylinder(){
radius = 1;
height = 1;
}
// Parametrized constructor to check that the
// variable is positive and less than 20, otherwise
// variable will be assigned its default value of 1.
cylinder(double r,double h){
if(r>0 && r<=20)
radius = r;
else
radius = 1;
if(h>0 && h<=20)
height = h;
else
height = 1;
}
// A reader for radius variable
double getRadius(){
return radius;
}
// A reader for height variable
double getHeight(){
return height;
}
// A writer for radius variable
void setRadius(double r){
radius = r;
}
// A writer for height variable
void setHeight(double h){
height = h;
}
// A reader for diameter
double getDiameter(){
return diameter;
}
// A writer for daimeter
void setDiameter(double d){
diameter = d;
}
// A member function “volume” which
// calculates the volume of a cylinder.
double volume(){
return PI*radius*radius*height;
}
// A member function “print” which prints
// the radius, diameter, and height of a cylinder.
void print(){
cout<<"\nRadius = "<<radius;
cout<<"\nDiameter = "<<diameter;
cout<<"\nHeight = "<<height;
}
// A member function display_name() which displays “cylinder
void display_name(){
cout<<"\nCylinder volume = "<<this->volume();
}
};
// Driver Program
int main()
{
// Creating cylinder class object
cylinder c(12,15);
c.setDiameter(24);
c.print();
c.display_name();
return 0;
}
question 2:
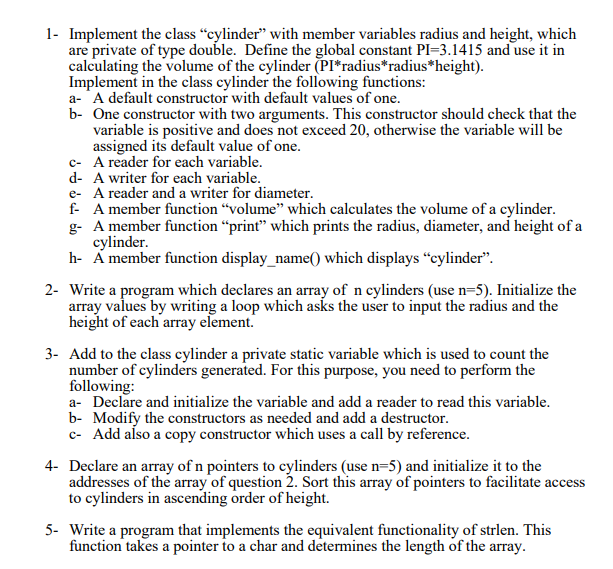

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

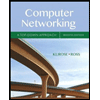
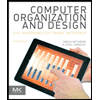
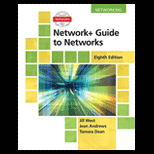
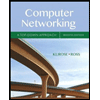
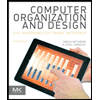
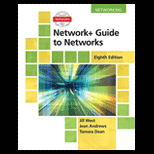
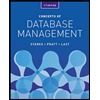
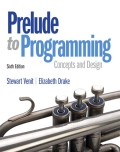
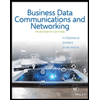