1) What is the purpose of the code below? 2) What is each function used for in the code? 3) What are the names of the parameters/ arguments and what are they responsible for? 4) Why did you use a while loop in you code? 5) How did you accomplish the sort() to sort numbers? Code:
1) What is the purpose of the code below?
2) What is each function used for in the code?
3) What are the names of the parameters/ arguments and what are they responsible for?
4) Why did you use a while loop in you code?
5) How did you accomplish the sort() to sort numbers?
Code:
#predefined modules
import random
import math
#function to sort the list in ascending order
def sort(x):
#predefined function sort()
x.sort()
#print the sorted list
print("\nSorted list is: ",str(x))
#function to find the sum of list elements
def sum_of_list(x):
#predefined function sum()
Sum=sum(x)
#return the sum of list elements
return Sum
#function to list the maximum from the list
def list_max(x):
#predefined function max()
maximum=max(x)
#return maximum
return maximum
#function to test the above three function
def main():
#set a flag variable
flag=True
#create a list
list1=list()
#initialize the list element by using randrange() predefined function of random module
list1=[random.randrange(1, 50, 1) for i in range(0,7)]
#print the original list
print("\nThe list element is shown below:\n" + str(list1))
#repeatative strurure while
while(flag):
# print the menu
print("\n******** Menu ********\n1. Sort the element\n2. Find the maximum from the list\n3. Find the sum of list element\n4. To exit the
# input user choice
ch = int(input("\nEnter your choice: "))
#decision struture
#if ch is 1
if(ch==1):
# sort the list
sort(list1)
#otherwise, if ch is 2
elif(ch==2):
# print the maximum from list
print("\nMaximum from the list element is: ", list_max(list1))
#otherwise, if ch is 3
elif(ch==3):
#print the sum of list
print("\nSum of list element is: ",sum_of_list(list1))
#otherwise
else:
flag=False
#function call
main()

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

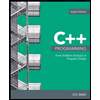
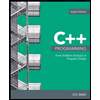