1. (Composition) Write a Pizza class so that this client code works. Please note that it is ok if the toppings are listed in a different order. >>> pie = Pizza() >>> pie Pizza('M',set()) >> pie.setsize('L') >>> pie.getsize() 'L' >>> pie.addTopping('pepperoni') >>> pie.addTopping ('anchovies') >>> pie.addTopping('mushrooms') >>> pie Pizza('L',{'anchovies', 'mushrooms', >>> pie.addTopping('pepperoni') >>> pie Pizza('L',{'anchovies', >>> pie.removeTopping('anchovies') >>> pie Pizza('L',{'mushrooms', 'pepperoni'}) >>> pie.price() pepperoni'}) 'mushrooms', pepperoni'}) 16.65 >>> pie2 = Pizza('L',{'mushrooms','pepperoni'}) >>> pie2 Pizza('L',{'mushrooms', >>> pie==pie2 'pepperoni'}) True The Pizza class should have two attributes(data items): size - a single character str, one of 'S','M’,L" toppings – a set containing the toppings. If you don't remember how to use a set, make sure you look it up in the book. Please note that toppings may be listed in a different order, but hw2TEST.py takes that into account. The Pizza class should have the following methods/operators): init - constructs a Pizza of a given size (defaults to 'M') and with a given set of toppings (defaults to empty set). I highly recommend you look at the Queue class in the book to see how to get this to work correctly. setSize – set pizza size to one of 'S','M’or 'L' getSize – returns size addTopping – adds a topping to the pizza, no duplicates, i.e., adding 'pepperoni' twice only adds it once removeTopping – removes a topping from the pizza price – returns the price of the pizza according to the following scheme: 'S': $6.25 plus 70 cents per topping 'M': $9.95 plus $1.45 per topping 'L': $12.95 plus $1.85 per topping repr - returns representation as a string – see output sample above. Note that toppings may be listed in a different order. _eq - two pizzas are equal if they have the same size and same toppings (toppings don't need to be in the same order)
1. (Composition) Write a Pizza class so that this client code works. Please note that it is ok if the toppings are listed in a different order. >>> pie = Pizza() >>> pie Pizza('M',set()) >> pie.setsize('L') >>> pie.getsize() 'L' >>> pie.addTopping('pepperoni') >>> pie.addTopping ('anchovies') >>> pie.addTopping('mushrooms') >>> pie Pizza('L',{'anchovies', 'mushrooms', >>> pie.addTopping('pepperoni') >>> pie Pizza('L',{'anchovies', >>> pie.removeTopping('anchovies') >>> pie Pizza('L',{'mushrooms', 'pepperoni'}) >>> pie.price() pepperoni'}) 'mushrooms', pepperoni'}) 16.65 >>> pie2 = Pizza('L',{'mushrooms','pepperoni'}) >>> pie2 Pizza('L',{'mushrooms', >>> pie==pie2 'pepperoni'}) True The Pizza class should have two attributes(data items): size - a single character str, one of 'S','M’,L" toppings – a set containing the toppings. If you don't remember how to use a set, make sure you look it up in the book. Please note that toppings may be listed in a different order, but hw2TEST.py takes that into account. The Pizza class should have the following methods/operators): init - constructs a Pizza of a given size (defaults to 'M') and with a given set of toppings (defaults to empty set). I highly recommend you look at the Queue class in the book to see how to get this to work correctly. setSize – set pizza size to one of 'S','M’or 'L' getSize – returns size addTopping – adds a topping to the pizza, no duplicates, i.e., adding 'pepperoni' twice only adds it once removeTopping – removes a topping from the pizza price – returns the price of the pizza according to the following scheme: 'S': $6.25 plus 70 cents per topping 'M': $9.95 plus $1.45 per topping 'L': $12.95 plus $1.85 per topping repr - returns representation as a string – see output sample above. Note that toppings may be listed in a different order. _eq - two pizzas are equal if they have the same size and same toppings (toppings don't need to be in the same order)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In Python IDLE: How do I write a class to satisfy the problem in the attached image?
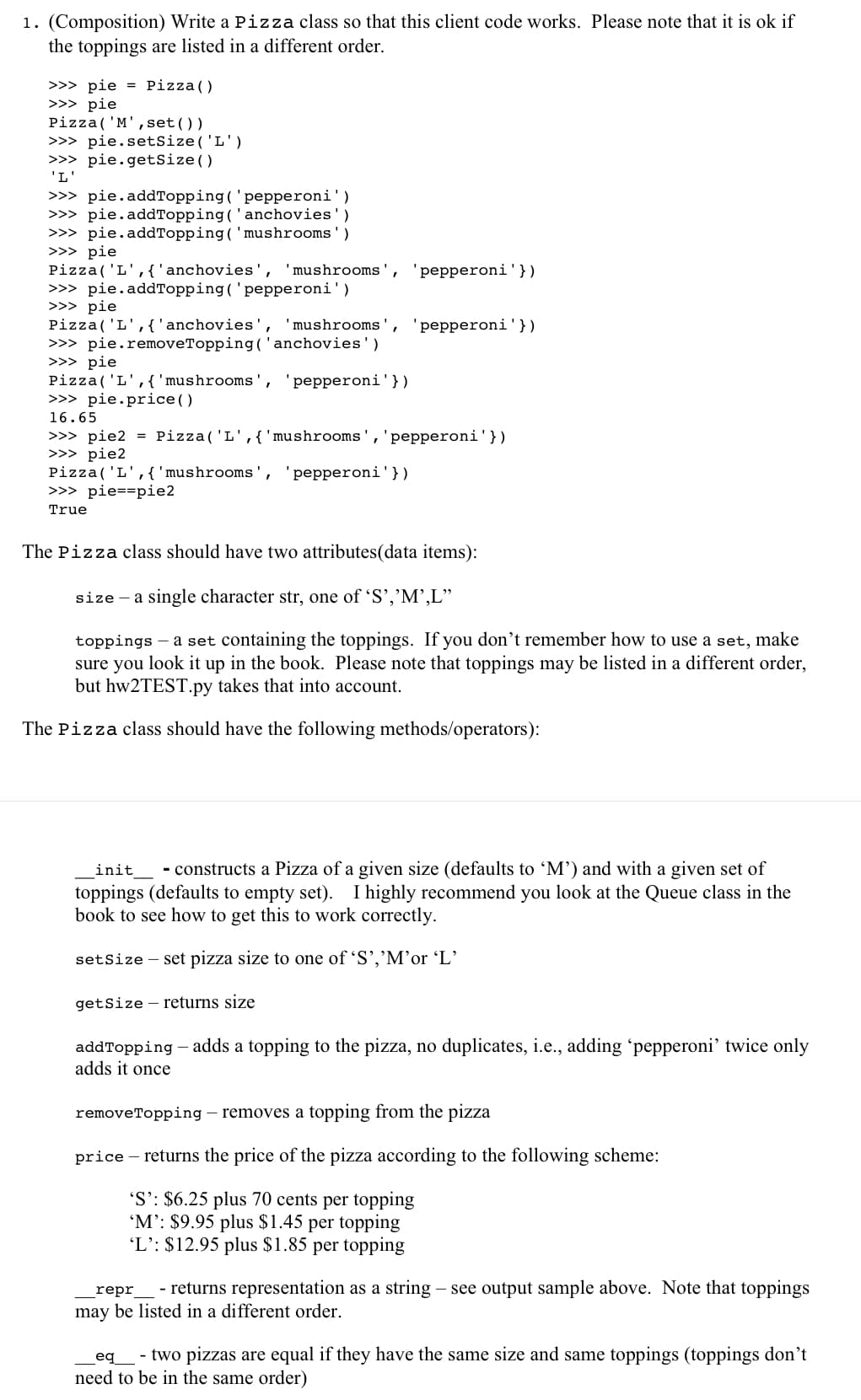
Transcribed Image Text:1. (Composition) Write a Pizza class so that this client code works. Please note that it is ok if
the toppings are listed in a different order.
>>> pie = Pizza()
>>> pie
Pizza('M',set())
>>> pie.setSize('L')
>>> pie.getSize()
'L'
>>> pie.addTopping('pepperoni')
>>> pie.addTopping('anchovies')
>>> pie.addTopping( 'mushrooms')
>>> pie
Pizza('L',{'anchovies',
>>> pie.addTopping('pepperoni')
>>> pie
Pizza('L',{'anchovies',
>>> pie.removeTopping('anchovies')
>>> pie
Pizza('L',{'mushrooms'
>>> pie.price()
'mushrooms'
'pepperoni'})
'mushrooms',
pepperoni'})
pepperoni'})
16.65
>>> pie2 = Pizza('L',{'mushrooms','pepperoni'})
>>> pie2
Pizza('L',{ 'mushrooms', 'pepperoni'})
>>> pie==pie2
True
The Pizza class should have two attributes(data items):
size - a single character str, one of 'S’,’M',L"
toppings – a set containing the toppings. If you don’t remember how to use a set, make
sure you look it up in the book. Please note that toppings may be listed in a different order,
but hw2TEST.py takes that into account.
The Pizza class should have the following methods/operators):
init
toppings (defaults to empty set). I highly recommend you look at the Queue class in the
book to see how to get this to work correctly.
- constructs a Pizza of a given size (defaults to 'M’) and with a given set of
setSize – set pizza size to one of 'S’,’M’or 'L'
getSize – returns size
addTopping – adds a topping to the pizza, no duplicates, i.e., adding 'pepperoni' twice only
adds it once
removeTopping – removes a topping from the pizza
price – returns the price of the pizza according to the following scheme:
'S': $6.25 plus 70 cents per topping
'M': $9.95 plus $1.45 per topping
'L': $12.95 plus $1.85 per topping
repr_ - returns representation as a string – see output sample above. Note that toppings
may be listed in a different order.
eq_ - two pizzas are equal if they have the same size and same toppings (toppings don't
need to be in the same order)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
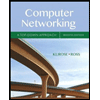
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
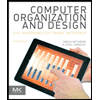
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
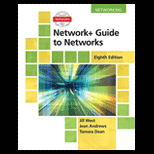
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
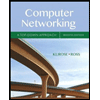
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
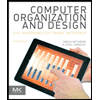
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
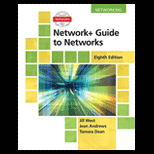
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
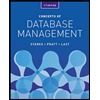
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
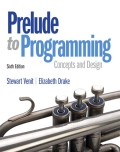
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
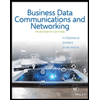
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY