1. Create a class called EuclideanAlgorithm. 2. Create the main method inside the EuclideanAlgorithm class. 3. Inside the main method, create the following variables: a. Declare and initialize an int named num1 with any integer in the range [1, 1000] of your choice. b. Declare and initialize an int named num2 with any integer in the range [1, 1000] of your choice. c. Declare an int named steps and initialize it a value of 0. d. Declare an int named dividend and initialize it to the value of num1 (do not hardcode using the literal value). e. Declare an int named divisor and initialize it to the value of num2 (do not hardcode using the literal value). f. Declare an int named gcd. g. Declare an int named quotient. h. Declare an int named remainder. 4. Using printf with appropriate format specifiers, print the following on its own line: Finding the greatest common divisor of {num1} and {num2}. 5. Using an if-else statement: a. If divisor is greater than dividend: i. Print the following on its own line: The inputs would have caused an unnecessary step. ii. Swap the values of divisor and dividend. b. Otherwise: i. Print: An extra step was avoided. 6. Within a do-while loop, perform the following steps while remainder is not equal to zero: a. Increment steps. b. Assign dividend / divisor to quotient. c. Assign the remainder of dividend / divisor to remainder. d. Using printf with appropriate format specifiers, print the following on its own line: Step {steps}: {dividend} = {divisor} * {quotient} + {remainder} e. Update gcd to the value of divisor. f. Update dividend to the value of divisor. g. Update divisor to the value of remainder. 8. Using a switch statement, print one of the following statements on its own line depending on the value of steps: a. 1: Only one step was needed! b. 2: Two steps were taken! c. 3: This process took three steps. d. 4: Wow! Four steps. e. In all other cases: (steps} steps is a lot of steps! 9. Using a ternary expression (?:) and printf, print one of the following statements on its own line depending on whether num1 and num2 are relatively prime: a. If num1 and num2 are relatively prime: i. {num1} and {num2} are relatively prime. b. Otherwise: i. {num1} and {num2} are not relatively prime.
1. Create a class called EuclideanAlgorithm. 2. Create the main method inside the EuclideanAlgorithm class. 3. Inside the main method, create the following variables: a. Declare and initialize an int named num1 with any integer in the range [1, 1000] of your choice. b. Declare and initialize an int named num2 with any integer in the range [1, 1000] of your choice. c. Declare an int named steps and initialize it a value of 0. d. Declare an int named dividend and initialize it to the value of num1 (do not hardcode using the literal value). e. Declare an int named divisor and initialize it to the value of num2 (do not hardcode using the literal value). f. Declare an int named gcd. g. Declare an int named quotient. h. Declare an int named remainder. 4. Using printf with appropriate format specifiers, print the following on its own line: Finding the greatest common divisor of {num1} and {num2}. 5. Using an if-else statement: a. If divisor is greater than dividend: i. Print the following on its own line: The inputs would have caused an unnecessary step. ii. Swap the values of divisor and dividend. b. Otherwise: i. Print: An extra step was avoided. 6. Within a do-while loop, perform the following steps while remainder is not equal to zero: a. Increment steps. b. Assign dividend / divisor to quotient. c. Assign the remainder of dividend / divisor to remainder. d. Using printf with appropriate format specifiers, print the following on its own line: Step {steps}: {dividend} = {divisor} * {quotient} + {remainder} e. Update gcd to the value of divisor. f. Update dividend to the value of divisor. g. Update divisor to the value of remainder. 8. Using a switch statement, print one of the following statements on its own line depending on the value of steps: a. 1: Only one step was needed! b. 2: Two steps were taken! c. 3: This process took three steps. d. 4: Wow! Four steps. e. In all other cases: (steps} steps is a lot of steps! 9. Using a ternary expression (?:) and printf, print one of the following statements on its own line depending on whether num1 and num2 are relatively prime: a. If num1 and num2 are relatively prime: i. {num1} and {num2} are relatively prime. b. Otherwise: i. {num1} and {num2} are not relatively prime.
Chapter8: Arrays
Section: Chapter Questions
Problem 2CP
Related questions
Question
For 8e, how would one go about creating a case for all numbers higher than x? Using >= and > seem to be illegal useages in Java.
For 9. Just confused on how to ternary expressions to check the GCDs.
Any help would be much appreciated!
![1. Create a class called EuclideanAlgorithm.
2. Create the main method inside the EuclideanAlgorithm class.
3. Inside the main method, create the following variables:
a. Declare and initialize an int named num1 with any integer in the range [1, 1000] of your choice.
b. Declare and initialize an int named num2 with any integer in the range [1, 1000] of your choice.
c. Declare an int named steps and initialize it a value of 0.
d. Declare an int named dividend and initialize it to the value of num1 (do not hardcode using the
literal value).
e. Declare an int named divisor and initialize it to the value of num2 (do not hardcode using the
literal value).
f. Declare an int named gcd.
g. Declare an int named quotient.
h. Declare an int named remainder.
4. Using printf with appropriate format specifiers, print the following on its own line: Finding the greatest
common divisor of {num1} and {num2}.
5. Using an if-else statement:
a. If divisor is greater than dividend:
i. Print the following on its own line: The inputs would have caused an unnecessary step.
ii. Swap the values of divisor and dividend.
b. Otherwise:
i. Print: An extra step was avoided.
6. Within a do-while loop, perform the following steps while remainder is not equal to zero:
a. Increment steps.
b. Assign dividend / divisor to quotient.
c. Assign the remainder of dividend / divisor to remainder.
d. Using printf with appropriate format specifiers, print the following on its own line: Step {steps}:
{dividend} = {divisor} * {quotient} + {remainder}
e. Update gcd to the value of divisor.
f. Update dividend to the value of divisor.
g. Update divisor to the value of remainder.
8. Using a switch statement, print one of the following statements on its own line depending on the value
of steps:
a. 1: Only one step was needed!
b. 2: Two steps were taken!
c. 3: This process took three steps.
d. 4: Wow! Four steps.
e. In all other cases: (steps} steps is a lot of steps!
9. Using a ternary expression (?:) and printf, print one of the following statements on its own line
depending on whether num1 and num2 are relatively prime:
a. If num1 and num2 are relatively prime: i. {num1} and {num2} are relatively prime.
b. Otherwise: i. {num1} and {num2} are not relatively prime.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F295c436f-1881-4143-81a2-89f6f40be87f%2Fdca8a8a4-cd17-4d3a-866f-6ebcf140be07%2F8jcv8xv_processed.png&w=3840&q=75)
Transcribed Image Text:1. Create a class called EuclideanAlgorithm.
2. Create the main method inside the EuclideanAlgorithm class.
3. Inside the main method, create the following variables:
a. Declare and initialize an int named num1 with any integer in the range [1, 1000] of your choice.
b. Declare and initialize an int named num2 with any integer in the range [1, 1000] of your choice.
c. Declare an int named steps and initialize it a value of 0.
d. Declare an int named dividend and initialize it to the value of num1 (do not hardcode using the
literal value).
e. Declare an int named divisor and initialize it to the value of num2 (do not hardcode using the
literal value).
f. Declare an int named gcd.
g. Declare an int named quotient.
h. Declare an int named remainder.
4. Using printf with appropriate format specifiers, print the following on its own line: Finding the greatest
common divisor of {num1} and {num2}.
5. Using an if-else statement:
a. If divisor is greater than dividend:
i. Print the following on its own line: The inputs would have caused an unnecessary step.
ii. Swap the values of divisor and dividend.
b. Otherwise:
i. Print: An extra step was avoided.
6. Within a do-while loop, perform the following steps while remainder is not equal to zero:
a. Increment steps.
b. Assign dividend / divisor to quotient.
c. Assign the remainder of dividend / divisor to remainder.
d. Using printf with appropriate format specifiers, print the following on its own line: Step {steps}:
{dividend} = {divisor} * {quotient} + {remainder}
e. Update gcd to the value of divisor.
f. Update dividend to the value of divisor.
g. Update divisor to the value of remainder.
8. Using a switch statement, print one of the following statements on its own line depending on the value
of steps:
a. 1: Only one step was needed!
b. 2: Two steps were taken!
c. 3: This process took three steps.
d. 4: Wow! Four steps.
e. In all other cases: (steps} steps is a lot of steps!
9. Using a ternary expression (?:) and printf, print one of the following statements on its own line
depending on whether num1 and num2 are relatively prime:
a. If num1 and num2 are relatively prime: i. {num1} and {num2} are relatively prime.
b. Otherwise: i. {num1} and {num2} are not relatively prime.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
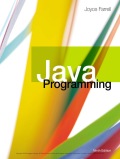
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
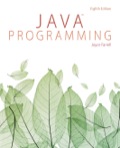
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
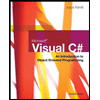
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
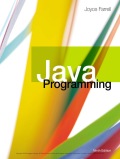
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
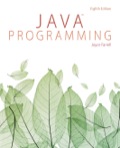
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
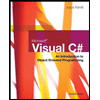
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,