ent that holds an apartment number as aptNumber, number of bedrooms as bedrooms, number of baths as baths, and rent amount as rent. Create a default constructor that accepts no arguments and an overloaded constructor that accepts values for each data field. Also create a get method for each field. Part B Write an application called TestApartments tha
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Part A
Create a class named Apartment that holds an apartment number as aptNumber, number of bedrooms as bedrooms, number of baths as baths, and rent amount as rent.
Create a default constructor that accepts no arguments and an overloaded constructor that accepts values for each data field.
Also create a get method for each field.
Part B
Write an application called TestApartments that creates at least five Apartment objects. Then prompt a user to enter a minimum number of bedrooms required, a minimum number of baths required, and a maximum rent the user is willing to pay. Display data for all the Apartment objects that meet the user’s criteria or an appropriate message if no such apartments are available.
An example of the program is shown below:
Enter minimum number of bedrooms needed >> 2
Enter minimum number of bathrooms needed >> 1.5
Enter maximum rent willing to pay >> 1200
Apartments meeting criteria of
at least 2 bedrooms, at least 1.5 baths, and no more than $1200.0 rent:
Apt #102 2 bedrooms, and 1.5 baths. Rent $775.0
Apt #103 3 bedrooms, and 2.0 baths. Rent $870.0
Apt #104 3 bedrooms, and 2.5 baths. Rent $960.0
Apt #105 3 bedrooms, and 3.0 baths. Rent $1100.0
Task 01: Create the Apartment class.
Task 02: Create the data fields for the Apartment class.
Task 03: Create a default constructor and an overloaded constructor for the Apartment class.
Task 04: Create the get method for the aptNumber data field.
Task 05: Create the get method for the bedrooms data field.
Task 06: Create the get method for the baths data field.
Task 07: Create the get method for the rent data field.
Task 08: Create the TestApartments class.
Task 09: The TestApartments class accepts user input for an Apartment object.
![|||| 0 a 2 400
G
Q testautomobile x Qapartment java X
CENGAGE COMPANION: COMMANDS
Open Companion Tab
Refresh Companion Tab
Bundle Current Workspace
https://literate-sniffle-x5wx9wg5x7w4fr5r.github.dev/?folder=/workspaces/9780357673423_java-programming-10e-5...
J TestApartments.java X J Apartment.java
J TestApartments.java > TestApartments
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68 }
// Check and display apartments meeting criteria
boolean isExist = false;
}
chegg.com/hon X
for (int i = 0; i < apts.length; i++) {
if (checkApt(apts[i], bdrms, baths, rent)) {
display (apts[i]);
isExist = true;
}
}
MindTap - Ceng X
}
// Displaying a message if no apartments meet the criteria
if (!isExist) {
System.out.println("No apartments met your criteria");
€03
> Codespaces: literate sniffle Ⓡ0A01 Java: Lightweight Mode
}
// Method to check if an apartment meets the criteria
public static boolean checkApt (Apartment apt, int bdrms, double baths,
if (apt.getBedrooms() >= bdrms && apt.getBaths() >= baths && apt.g
return true;
return false;
student [Codespaces: literate sniffle]
// Method to display apartment details
public static void display (Apartment apt) {
System.out.println(" Apt #" + apt.getAptNumber() + " " + apt.getBe
ER
Companion - st X
HouTLE MA
WAL
*
YJUMI TELAH
man
J Apartment.java
Part A
Success Confirm X
All
Q
Companion X
New tab
()
+
·1+
De 08
0:
Create a class named Apartment that holds an apartment number as aptNumber
number of bedrooms as bedrooms, number of baths as baths, and rent amoun
as rent.
Create a default constructor that accepts no arguments and an overloade
constructor that accepts values for each data field.
Also create a get method for each field.
Part B
Write an application called TestApartments that creates at least five Apartmen
objects. Then prompt a user to enter a minimum number of bedrooms requirec
a minimum number of baths required, and a maximum rent the user is willing t
pay. Display data for all the Apartment objects that meet the user's criteria or a
appropriate message if no such apartments are available.
An example of the program is shown below:
Enter minimum number of bedrooms needed >> 2
Enter minimum number of bathrooms needed >> 1.5
Enter maximum rent willing to pay >> 1200
Apartments meeting criteria of
at least 2 bedrooms, at least 1.5 baths, and no more than $1200.0 rent
Apt #102 2 bedrooms, and 1.5 baths. Rent $775.0
Apt #103
3 bedrooms, and 2.0 baths. Rent $870.0
Apt #104
3 bedrooms, and 2.5 baths. Rent $960.0
Apt #105
3 bedrooms, and 3.0 baths. Rent $1100.0
B
4
✪
<>
Layout: US
...
U](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6cfcbabe-0283-4f69-afa3-58c47651b2d0%2Ffa87a50d-47f9-4996-8d52-62f8b7017355%2F07giieq_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

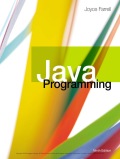
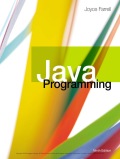